How to Wait for a Thread to Finish in C#
-
Wait for a Thread to Finish With the
Task.WaitAll()
Method inC#
-
Wait for a Thread to Finish With the
Thread.Join()
Method inC#
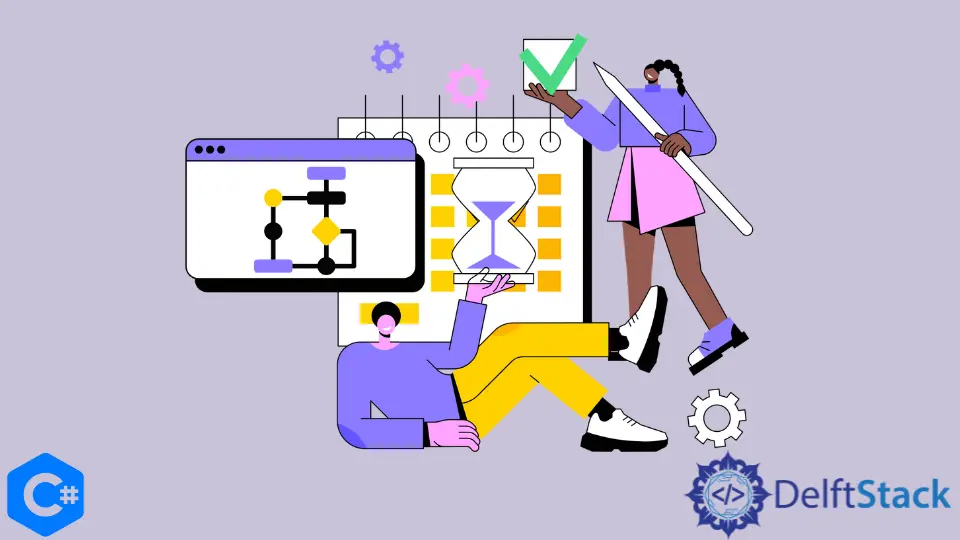
This tutorial will discuss the methods of waiting for the completion of a thread in C#.
Wait for a Thread to Finish With the Task.WaitAll()
Method in C#
The Task.WaitAll()
method in C# is used to wait for the completion of all the objects of the Task
class. The Task
class represents an asynchronous task in C#. We can start threads with the Task
class and wait for the threads to finish with the Task.WaitAll()
method in C#.
using System;
using System.Threading.Tasks;
namespace wait_for_thread {
class Program {
static void fun1() {
for (int i = 0; i < 2; i++) {
Console.WriteLine("Thread 1");
}
}
static void fun2() {
for (int i = 0; i < 2; i++) {
Console.WriteLine("Thread 2");
}
}
static void Main(string[] args) {
Task thread1 = Task.Factory.StartNew(() => fun1());
Task thread2 = Task.Factory.StartNew(() => fun2());
Task.WaitAll(thread1, thread2);
Console.WriteLine("The End");
}
}
}
Output:
Thread 1
Thread 1
Thread 2
Thread 2
The End
In the above code, we waited for the completion of the thread1
and thread2
tasks inside the main thread with the Task.WaitAll()
method in C#.
Wait for a Thread to Finish With the Thread.Join()
Method in C#
In the above section, we discussed how we could wait for a thread with the Task.WaitAll()
method in C#. We can also achieve the same goal with the Thread.Join()
method in C#. The Thread.Join()
method halts the execution of the calling thread until the current thread completes its execution. The following code example shows us how to wait for a thread to complete its execution with the Thread.Join()
method in C#.
using System;
using System.Threading.Tasks;
namespace wait_for_thread {
class Program {
static void fun1() {
for (int i = 0; i < 2; i++) {
Console.WriteLine("Thread 1");
}
}
static void fun2() {
for (int i = 0; i < 2; i++) {
Console.WriteLine("Thread 2");
}
}
static void Main(string[] args) {
Thread thread1 = new Thread(new ThreadStart(fun1));
Thread thread2 = new Thread(new ThreadStart(fun2));
thread1.Start();
thread1.Join();
thread2.Start();
thread2.Join();
Console.WriteLine("The End");
}
}
}
Output:
Thread 1
Thread 1
Thread 2
Thread 2
The End
In the above code, we waited for the thread1
and thread2
threads to finish inside the main thread with the Thread.Join()
method in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn