How to Create Scheduled Tasks in C#
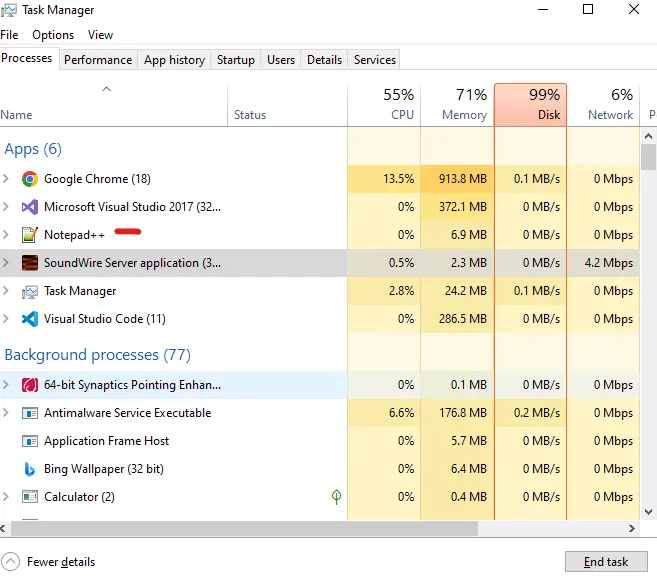
The following article will guide you on scheduling tasks using the programming language C#.
C# Task Scheduler
In the following example, we’ll utilize Windows Task Scheduler
, a component that automatically executes tasks at predefined times or in reaction to triggered events. Tasks can be scheduled to execute at various times, such as when the computer boots up or when a user checks in.
Task scheduler allows you to set up events that will trigger the launch of predefined tasks and run commands and execute scripts at certain times and dates.
Add NuGet
Reference Package
We’ll add the TaskScheduler
package for Windows task scheduling operations. To do that, follow the below steps.
-
Open
Visual Studio
, create aConsole Application
, and name it. -
Right-click on the
Solution Explorer
panel and SelectManage NuGet Packages
. -
Now click on the
Browse
option, search forTaskScheduler
and install it.
After adding the package, import the following libraries:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Microsoft.Win32.TaskScheduler;
We’ll initiate TaskService
to start the local machine’s task service.
using (TaskService ts = new TaskService()) {}
Now, create a new task definition as td
and give characteristics such as the author or description.
TaskDefinition td = ts.NewTask();
td.RegistrationInfo.Author = "Muhammad Zeeshan";
td.RegistrationInfo.Description = "Open Notepad++ for working";
We need to ensure that we have an action set up so that Notepad++ is launched once the trigger is triggered.
td.Actions.Add(new ExecAction(@"C:\Program Files\Notepad++\notepad++.exe"));
After that, we must register the job in the primary folder.
ts.RootFolder.RegisterTaskDefinition(@"My Scheduled Notepad++ Task", td).Run();
Lastly, we can insert an interval of the day shown below. Schedule the job to begin every other day at this time by setting a trigger.
td.Triggers.Add(new DailyTrigger { DaysInterval = 1 });
Complete Source Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Microsoft.Win32.TaskScheduler;
namespace ScheduledTasksbyZeeshan {
class Program {
static void Main(string[] args) {
using (TaskService ts = new TaskService()) {
TaskDefinition td = ts.NewTask();
td.RegistrationInfo.Author = "Muhammad Zeeshan";
td.RegistrationInfo.Description = "Open Notepad++ for working";
td.Actions.Add(new ExecAction(@"C:\Program Files\Notepad++\notepad++.exe"));
ts.RootFolder.RegisterTaskDefinition(@"My Scheduled Notepad++ Task", td).Run();
}
}
}
}
Output:
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn