How to Add a Tab to a String in C#
- Add Tab Space Inside a String Using the Tab Escape Sequence in C#
-
Add Tab Space Inside a String Using the
StringBuilder
Class in C# - Add Tab Space Inside a String Using String Interpolation
-
Add Tab Space Inside a String Using the
String.Concat
Method -
Add Tab Space Inside a String Using the
+
Operator -
Add Tab Space Inside a String Using the
String.Format
Method - Conclusion
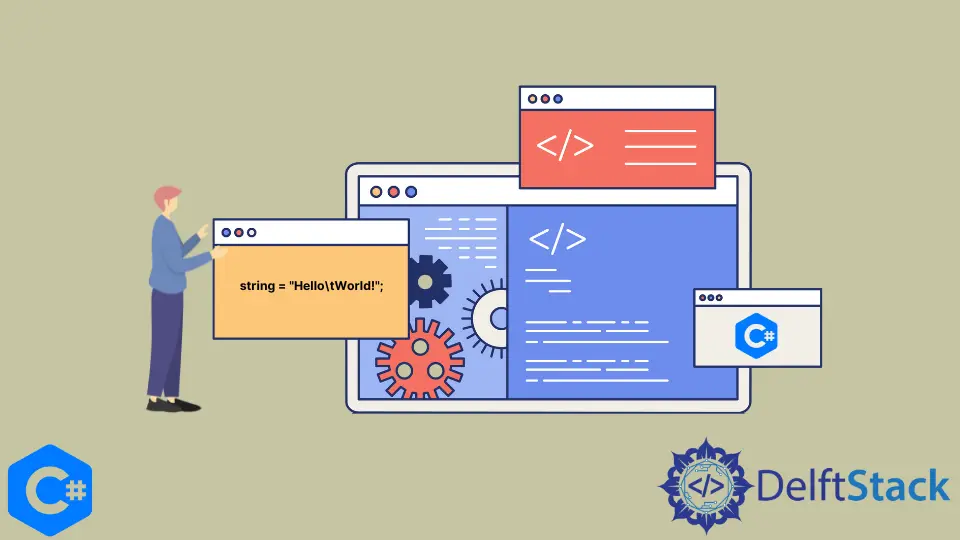
Adding tabs to strings in C# can be essential for formatting text or creating structured output. There are several methods to achieve this, each with its syntax and use cases.
In this article, we will explore these methods in detail and provide code examples for each.
Add Tab Space Inside a String Using the Tab Escape Sequence in C#
The simplest way to add a tab to a string in C# is by using the tab escape sequence, \t
. This is a special character sequence recognized by C# and many other programming languages.
When you include \t
in a string, it represents a horizontal tab character. When the string is displayed or printed, a tab character causes the text cursor to move to the next tab stop position, aligning the text accordingly.
For example, consider the following code:
string formattedText = "Name:\tJohn";
Console.WriteLine(formattedText);
Output:
Name: John
As you can see, the \t
escape sequence inserted a tab character between Name:
and John
, creating even spacing.
You can also use multiple tab escape sequences to add multiple tabs in a row. For instance:
// insert three tab characters
string formattedText = "Name:\t\t\tJohn";
Console.WriteLine(formattedText);
Output:
Name: John
Additionally, the tab escape sequence can be used in combination with regular text to format your strings as needed. Here’s an example:
string formattedText = "Name:\t\tJohn\tDoe";
Console.WriteLine(formattedText);
Output:
Name: John Doe
In this case, tabs were used to align John
and Doe
under Name
.
Add Tab Space Inside a String Using the StringBuilder
Class in C#
The StringBuilder
class is part of the System.Text
namespace and is designed to efficiently build and manipulate strings. Unlike regular strings, which are immutable in C#, StringBuilder
allows you to modify a string’s content without creating new string objects each time.
This makes it a suitable choice for tasks that involve frequent string manipulation, such as adding tabs.
Here’s the complete example of adding a tab to a string using the StringBuilder
class:
using System;
using System.Text;
class Program {
static void Main() {
// Create a new StringBuilder object to efficiently manipulate strings
StringBuilder stringBuilder = new StringBuilder();
// Define the first part of the string
string originalString = "Hello,";
stringBuilder.Append(originalString);
// Add a tab character to the string using the Append method
stringBuilder.Append('\t'); // Add a tab character
// Define and append the rest of the string
string restOfString = "World!";
stringBuilder.Append(restOfString);
// Convert the StringBuilder content to a regular string
string finalString = stringBuilder.ToString();
// Print the final string to the console
Console.WriteLine(finalString);
}
}
Output:
Hello, World!
Add Tab Space Inside a String Using String Interpolation
String interpolation simplifies the process of creating formatted strings by embedding expressions within string literals. It involves prefixing a string literal with a $
symbol and enclosing expressions within curly braces {}
.
The expressions within the curly braces are evaluated and replaced with their values at runtime.
Here’s a basic example of string interpolation:
string name = "John";
int age = 30;
string message = $"Hello, my name is {name} and I am {age} years old.";
Here, the values of name
and age
are inserted into the string, resulting in the message
variable containing the formatted string.
Now, to add a tab character to a string using string interpolation, you can include the \t
escape sequence within the curly braces. Here’s how you can do this:
using System;
class Program {
static void Main() {
string itemName = "Widget";
decimal price = 19.99M;
string formattedItem = $"Item: \t{itemName}\nPrice: \t${price}";
Console.WriteLine(formattedItem);
// Keep the console window open
Console.ReadLine();
}
}
Output:
Item: Widget
Price: $19.99
In this example, \t
is used to insert a tab character before the item name and the price, creating a neatly formatted output. The \n
character is used to add a newline for better readability.
If you need to customize the number of spaces a tab represents, you can do so by using additional \t
characters. Each \t
character typically represents one tab space, but you can use multiple \t
characters to increase the spacing.
For instance, if you want a double tab space, you can use \t\t
.
using System;
class Program {
static void Main() {
string itemName = "Widget";
decimal price = 19.99M;
string formattedItem = $"Item: \t\t{itemName}\nPrice: \t\t${price}";
Console.WriteLine(formattedItem);
Console.ReadLine();
}
}
Output:
Item: Widget
Price: $19.99
In this modified example, two \t
characters are used to create a double tab space before the item name and the price.
Add Tab Space Inside a String Using the String.Concat
Method
The String.Concat
method is a versatile way to concatenate multiple strings, including adding a tab character to an existing string. It is part of the System
namespace and is readily available in any C# application.
This method takes one or more string arguments and combines them into a single string.
Here’s the basic syntax of the String.Concat
method:
string result = string.Concat(string1, string2, string3, ...);
To add a tab character to a string using String.Concat
, include the tab character ("\t"
) within the concatenation:
using System;
class Program {
static void Main() {
string product1 = "Laptop";
string price1 = "$999";
string product2 = "Smartphone";
string price2 = "$499";
string formattedOutput = string.Concat(product1, "\t", price1, "\n", product2, "\t", price2);
Console.WriteLine(formattedOutput);
}
}
Output:
Laptop $999
Smartphone $499
As you can see, this code produced output with aligned columns.
Add Tab Space Inside a String Using the +
Operator
The +
operator in C# is primarily used for string concatenation. This means you can combine multiple strings into a single string using this operator.
To add tab spaces inside a string, you can concatenate the desired number of tab characters to the existing string.
Here’s an example:
using System;
class Program {
static void Main() {
string originalString = "This is a sample string.";
// Adding a single tab space (equivalent to four spaces)
string stringWithTab = originalString + "\tTabbed Text";
Console.WriteLine(stringWithTab);
}
}
Output:
This is a sample string. Tabbed Text
In this example, we started with the originalString
, and then we concatenated a tab character ("\t"
) and the text "Tabbed Text"
using the +
operator. The resulting stringWithTab
now has a tab space inserted before Tabbed Text
.
You can adjust the number of tab spaces by adding multiple tab characters. For instance, "\t\t"
would represent two tab spaces, equivalent to eight spaces.
Add Tab Space Inside a String Using the String.Format
Method
The String.Format
method allows you to create a formatted string by combining a template string with one or more values. The template string contains placeholders, which are enclosed in curly braces {}
and are replaced with the specified values at runtime.
Here’s the basic syntax of the String.Format
method:
string formattedString = string.Format(format, arg0, arg1, ...);
Parameters:
format
: The template string with placeholders.arg0
,arg1
, …: The values to replace the placeholders.
To add tab spaces, you can use the \t
escape sequence in your format string. Here’s how you can do it:
string name = "John";
int age = 30;
string formattedString = string.Format("Name:\t{0}\nAge:\t{1}", name, age);
In this example, we’ve used the \t
escape sequence to insert a tab space before the values of name
and age
.
The resulting formattedString
will look like this:
Name: John
Age: 30
Conclusion
In conclusion, adding tabs to strings in C# is a common task, and there are various methods to achieve it.
The choice of method depends on your specific requirements and coding style preferences. Whether you prefer simplicity, performance, or flexibility, C# provides multiple options to cater to your needs.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn