How to Split a String on Newline in C#
-
Use
String.Split()
to Split a String on Newline in C# -
Use
Regex.Split()
to Split a String on Newline in C# -
Use
StringSplitOptions.RemoveEmptyEntries
WithString.Split()
to Split a String on Newline in C# -
Use
String.Join
andString.Split()
to Split a String on Newline in C# - Conclusion
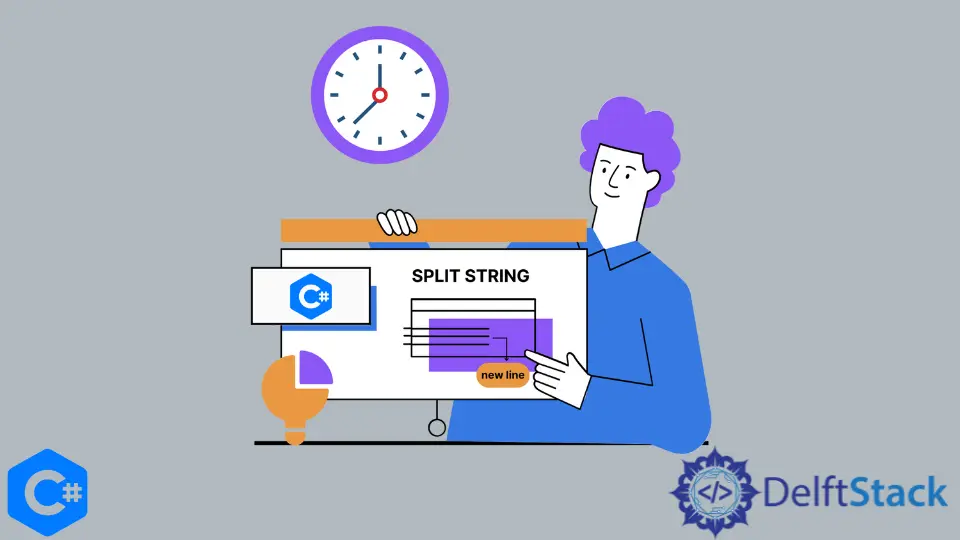
In C#, working with strings is a common task, and sometimes, you may need to split a string into multiple parts based on newline characters. This can be particularly useful when dealing with text data or files where each line represents a distinct piece of information.
In this article, we will explore how to split a string on newline using a specific character in C#.
Use String.Split()
to Split a String on Newline in C#
The String.Split()
method provides a convenient way to split a string into multiple parts based on a specific character.
The String.Split()
method is a member of the System.String
class in C#. It is designed to split a string into an array of substrings based on a specified delimiter or array of delimiters.
The basic syntax is as follows:
string[] result = inputString.Split(separator);
Here, inputString
is the string to be split, and separator
is the character or characters that determine where the string should be split. The result is an array of substrings.
The String.Split()
method works by scanning the input string from left to right and identifying occurrences of the specified separator. It then breaks the string into substrings at those points and returns an array containing these substrings.
Note that empty entries in the array may occur if there are consecutive occurrences of the separator or if the string starts or ends with the separator.
Let’s consider a practical example where we have a string and want to split it based on the space character. We will then print each part on a new line using a loop.
using System;
public class SplitStringExample {
public static void Main(string[] args) {
string inputString = "C# is a powerful programming language.";
string[] parts = inputString.Split(' ');
Console.WriteLine("Original String:");
Console.WriteLine(inputString);
Console.WriteLine("\nSplit String on Space Character:");
foreach (string part in parts) {
Console.WriteLine(part);
}
}
}
Here, inputString
is the original string, and pattern
is the regular expression pattern that defines where the string should be split.
Let’s consider a scenario where we have a string and want to split it based on spaces or commas. We will then print each part on a new line using a loop.
using System;
using System.Text.RegularExpressions;
public class RegexSplitExample {
public static void Main(string[] args) {
string inputString = "C#,Java,Python,C++";
string[] parts = Regex.Split(inputString, "[ ,]+");
Console.WriteLine("Original String:");
Console.WriteLine(inputString);
Console.WriteLine("\nSplit String on Spaces or Commas using Regex.Split:");
foreach (string part in parts) {
Console.WriteLine(part);
}
}
}
Here, inputString
is the original string, separator
is the character at which the string should be split, and StringSplitOptions.RemoveEmptyEntries
ensures that empty entries are removed from the resulting array.
Let’s consider an example where we have a string with multiple consecutive spaces, and we want to split it based on spaces.
using System;
public class SplitWithOptionsExample {
public static void Main(string[] args) {
string inputString = "C# is an amazing language.";
string[] parts = inputString.Split(new[] { ' ' }, StringSplitOptions.RemoveEmptyEntries);
Console.WriteLine("Original String:");
Console.WriteLine(inputString);
Console.WriteLine("\nSplit String on Spaces with StringSplitOptions.RemoveEmptyEntries:");
foreach (string part in parts) {
Console.WriteLine(part);
}
}
}
Here, inputString
is the original string, separator
is the character at which the string should be split, and Environment.NewLine
represents the newline character sequence.
Consider a scenario where we have a string with words separated by semicolons, and we want to split it based on semicolons. We will then print each part on a new line using String.Join
and String.Split()
.
using System;
public class JoinAndSplitExample {
public static void Main(string[] args) {
string inputString = "C#;Java;Python;C++";
string[] parts = inputString.Split(';');
string result = string.Join(Environment.NewLine, parts);
Console.WriteLine("Original String:");
Console.WriteLine(inputString);
Console.WriteLine("\nSplit String on Semicolons with String.Join and String.Split:");
Console.WriteLine(result);
}
}
Output:
Original String:
C#;Java;Python;C++
Split String on Semicolons with String.Join and String.Split:
C#
Java
Python
C++
Here, we also use the System
namespace and define a class named JoinAndSplitExample
with its Main
method.
Similar to the second code example above, the inputString
is set to C#;Java;Python;C++
to simulate a string with programming language names separated by semicolons.
The String.Split(';')
method is applied to split the string based on semicolons, resulting in an array of substrings stored in the parts
variable.
The subsequent steps involve using String.Join(Environment.NewLine, parts)
to concatenate the substrings with newline as the separator, forming a single string.
The original string is printed, along with a message indicating that we are about to display the split string on new lines.
Finally, the joined string is printed using Console.WriteLine(result)
.
This example showcases a concise and readable approach to splitting a string and printing each part on a new line using the combination of String.Join
and String.Split()
.
Conclusion
We’ve explored various techniques for splitting strings and printing each part on a new line in C#. The fundamental String.Split()
method allows for straightforward splitting based on a specific character.
For more intricate scenarios, Regex.Split()
offers flexibility through regular expression patterns. The combination of StringSplitOptions.RemoveEmptyEntries
with String.Split()
ensures cleaner results by excluding empty entries.
Additionally, the String.Join
and String.Split()
provides a concise and readable alternative for formatting output.
Whether handling simple delimiters or complex patterns, these methods allow us to efficiently manipulate strings, enhancing the versatility and expressiveness of string manipulation in C#.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn