How to Sort Dictionary by Value in C#
-
Sort Dictionary by Value With the List Method in
C#
-
Sort Dictionary by Value With the Linq Method in
C#
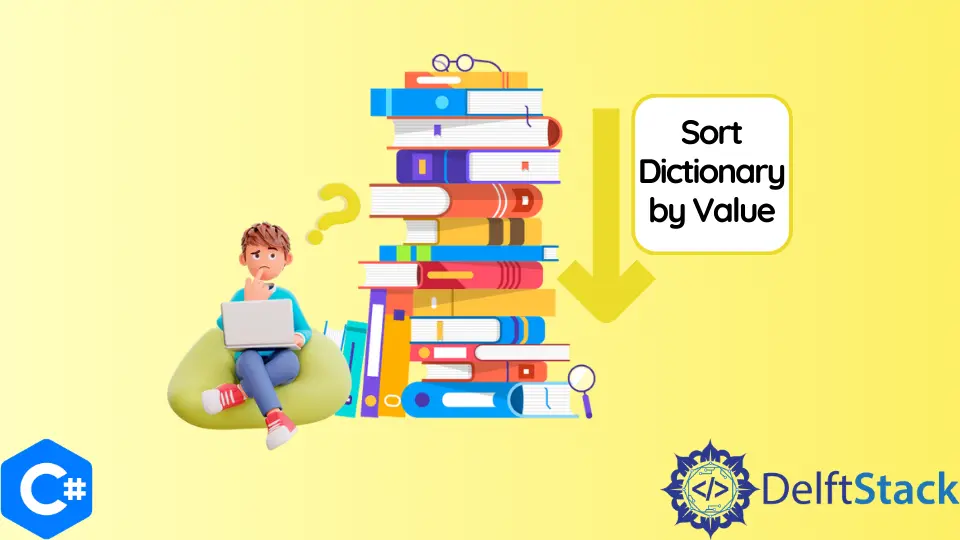
This tutorial will introduce methods to sort a dictionary by value in C#.
Sort Dictionary by Value With the List Method in C#
The C# dictionary data structure stores data in the form of key:value
pairs. Unfortunately, there is no built-in method to sort a dictionary by value in C#. We have to convert the dictionary to a list of tuples and then sort the list. The following code example shows us how to sort a dictionary by value with a list in C#.
using System;
using System.Collections.Generic;
using System.Linq;
namespace sort_dictionary_by_value {
class Program {
static void Main(string[] args) {
Dictionary<string, int> myDict = new Dictionary<string, int>();
myDict.Add("one", 1);
myDict.Add("four", 4);
myDict.Add("two", 2);
myDict.Add("three", 3);
var myList = myDict.ToList();
myList.Sort((pair1, pair2) => pair1.Value.CompareTo(pair2.Value));
foreach (var value in myList) {
Console.WriteLine(value);
}
}
}
}
Output:
[one, 1]
[two, 2]
[three, 3]
[four, 4]
We created the dictionary myDict
and sorted it by the integer value. We first converted the myDict
to the list of tuples myList
with the ToList()
function in C#. We then sorted the myList
with Linq and displayed the values.
Sort Dictionary by Value With the Linq Method in C#
We can also directly sort a dictionary by value without converting it to a list first. The Linq or language integrated query is used to perform SQL-like queries in C#. We can use Linq to sort a dictionary by value. The following code example shows us how to sort a dictionary by value with Linq in C#.
using System;
using System.Collections.Generic;
using System.Linq;
namespace sort_dictionary_by_value {
class Program {
static void Main(string[] args) {
Dictionary<string, int> myDict = new Dictionary<string, int>();
myDict.Add("one", 1);
myDict.Add("four", 4);
myDict.Add("two", 2);
myDict.Add("three", 3);
var sortedDict = from entry in myDict orderby entry.Value ascending select entry;
foreach (var value in sortedDict) {
Console.WriteLine(value);
}
}
}
}
Output:
[one, 1]
[two, 2]
[three, 3]
[four, 4]
We created the dictionary myDict
and sorted it by the integer value with Linq in C#. We stored the sorted dictionary inside sortedDict
and displayed the values.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn