How to Read a JSON File in C#
- Understanding JSON and Its Importance
- Setting Up Your C# Environment
- Reading JSON Files Using JsonConvert
- Handling Complex JSON Structures
- Error Handling While Reading JSON
- Conclusion
- FAQ
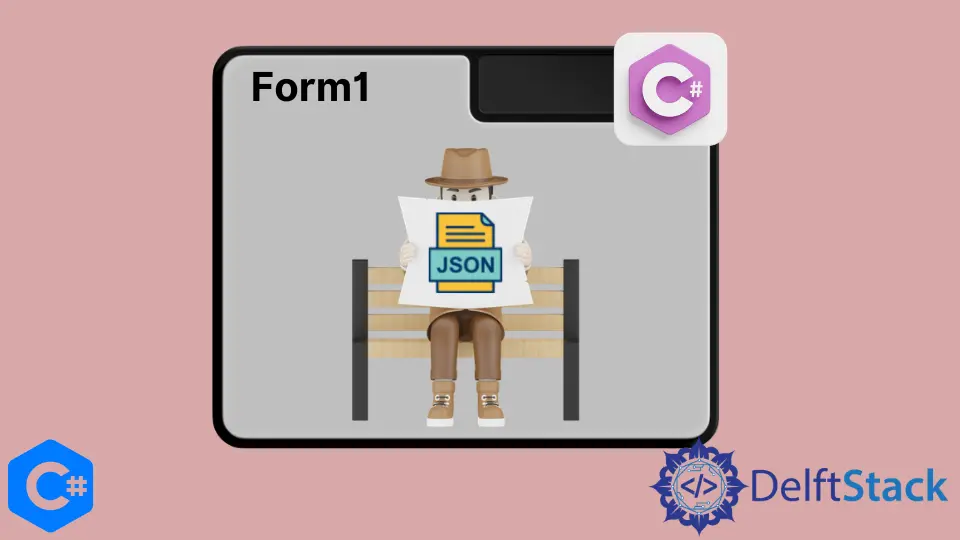
In today’s digital world, data interchange formats like JSON (JavaScript Object Notation) have become essential. If you’re working with C#, knowing how to read JSON files can be a game changer. The JsonConvert class in the Json.NET namespace is a powerful tool that simplifies the process of reading and writing JSON files. Whether you’re developing a web application, a desktop app, or a mobile solution, handling JSON data efficiently can enhance your application’s functionality.
In this article, we’ll explore various methods to read a JSON file in C#, complete with code examples and explanations. By the end, you’ll be well-equipped to handle JSON data in your C# projects.
Understanding JSON and Its Importance
JSON is a lightweight data format that is easy for humans to read and write and easy for machines to parse and generate. It is widely used for APIs and data storage, making it crucial for developers to know how to manipulate it. In C#, the Json.NET library is the most popular choice for working with JSON. It provides a simple way to convert between .NET objects and JSON, making your development process smoother and more efficient.
Setting Up Your C# Environment
Before diving into the code, ensure you have the Json.NET library installed. You can easily add it to your project using NuGet Package Manager. Open your Visual Studio, go to Tools > NuGet Package Manager > Manage NuGet Packages for Solution, and search for “Newtonsoft.Json”. Install it, and you’re ready to start reading JSON files.
Reading JSON Files Using JsonConvert
One of the most straightforward ways to read a JSON file in C# is by using the JsonConvert class. This class provides methods to deserialize JSON strings into .NET objects. Here’s a simple example to demonstrate how to read a JSON file.
using System;
using System.IO;
using Newtonsoft.Json;
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
class Program
{
static void Main()
{
string jsonFilePath = "path/to/your/file.json";
string jsonData = File.ReadAllText(jsonFilePath);
Person person = JsonConvert.DeserializeObject<Person>(jsonData);
Console.WriteLine($"Name: {person.Name}");
Console.WriteLine($"Age: {person.Age}");
}
}
Output:
Name: John Doe
Age: 30
In this example, we first define a Person
class with properties that match the JSON structure. We then read the JSON file using File.ReadAllText()
and deserialize it into a Person
object using JsonConvert.DeserializeObject<T>()
. This method converts the JSON data into a C# object seamlessly, allowing you to access the properties directly.
Handling Complex JSON Structures
Sometimes, you might encounter JSON files with more complex structures, such as arrays or nested objects. Json.NET can handle these scenarios as well. Let’s see how to read a JSON file that contains an array of objects.
using System;
using System.Collections.Generic;
using System.IO;
using Newtonsoft.Json;
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
class Program
{
static void Main()
{
string jsonFilePath = "path/to/your/file.json";
string jsonData = File.ReadAllText(jsonFilePath);
List<Person> people = JsonConvert.DeserializeObject<List<Person>>(jsonData);
foreach (var person in people)
{
Console.WriteLine($"Name: {person.Name}, Age: {person.Age}");
}
}
}
Output:
Name: John Doe, Age: 30
Name: Jane Smith, Age: 25
In this example, the JSON file contains an array of Person
objects. By deserializing it into a List<Person>
, we can easily iterate through the collection and access each person’s details. This flexibility makes Json.NET a powerful tool for handling various JSON formats.
Error Handling While Reading JSON
When working with JSON files, it’s essential to implement error handling to manage potential issues. This can include file not found exceptions or deserialization errors. Here’s how you can incorporate error handling into your JSON reading process.
using System;
using System.Collections.Generic;
using System.IO;
using Newtonsoft.Json;
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
class Program
{
static void Main()
{
string jsonFilePath = "path/to/your/file.json";
try
{
string jsonData = File.ReadAllText(jsonFilePath);
List<Person> people = JsonConvert.DeserializeObject<List<Person>>(jsonData);
foreach (var person in people)
{
Console.WriteLine($"Name: {person.Name}, Age: {person.Age}");
}
}
catch (FileNotFoundException)
{
Console.WriteLine("The specified file was not found.");
}
catch (JsonException)
{
Console.WriteLine("Error parsing JSON data.");
}
}
}
Output:
Name: John Doe, Age: 30
Name: Jane Smith, Age: 25
In this code, we use a try-catch block to handle potential exceptions. If the file is not found, a FileNotFoundException
is caught, and an appropriate message is displayed. Similarly, if there’s an issue with the JSON structure, a JsonException
will be caught, allowing you to handle errors gracefully.
Conclusion
Reading JSON files in C# is a straightforward task, especially with the help of the Json.NET library. By understanding the various methods to deserialize JSON data, including handling complex structures and implementing error handling, you can effectively manage JSON data in your applications. Whether you’re dealing with simple objects or intricate data structures, Json.NET provides the tools you need to work with JSON seamlessly. With these skills, you’re now better equipped to integrate JSON data into your C# projects, enhancing their functionality and user experience.
FAQ
-
How do I install Json.NET in my C# project?
You can install Json.NET via NuGet Package Manager in Visual Studio by searching for “Newtonsoft.Json”. -
Can I read JSON data directly from a URL in C#?
Yes, you can useHttpClient
to fetch JSON data from a URL and then deserialize it using JsonConvert. -
What happens if the JSON structure does not match my C# class?
If the JSON structure does not match the C# class, you may encounter exceptions or null values for properties that do not exist in the JSON. -
Is Json.NET the only library for handling JSON in C#?
No, while Json.NET is the most popular, there are other libraries like System.Text.Json that you can use for handling JSON data. -
Can I serialize C# objects back to JSON using Json.NET?
Yes, Json.NET provides methods to convert C# objects to JSON strings usingJsonConvert.SerializeObject()
.
using the JsonConvert class from the Json.NET namespace. This article provides step-by-step methods, code examples, and error handling techniques to help you efficiently manage JSON data in your applications. Discover the best practices for working with JSON in C# and enhance your programming skills today.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn