How to Read and Write to a File in C#
-
Write Data to a File With the
File.WriteAllText()
Method inC#
-
Read Data From a File With the
File.ReadAllText()
Method inC#
-
Write Data to a File With the
StreamWriter
Class inC#
-
Read Data From a File With the
StreamReader
Class inC#
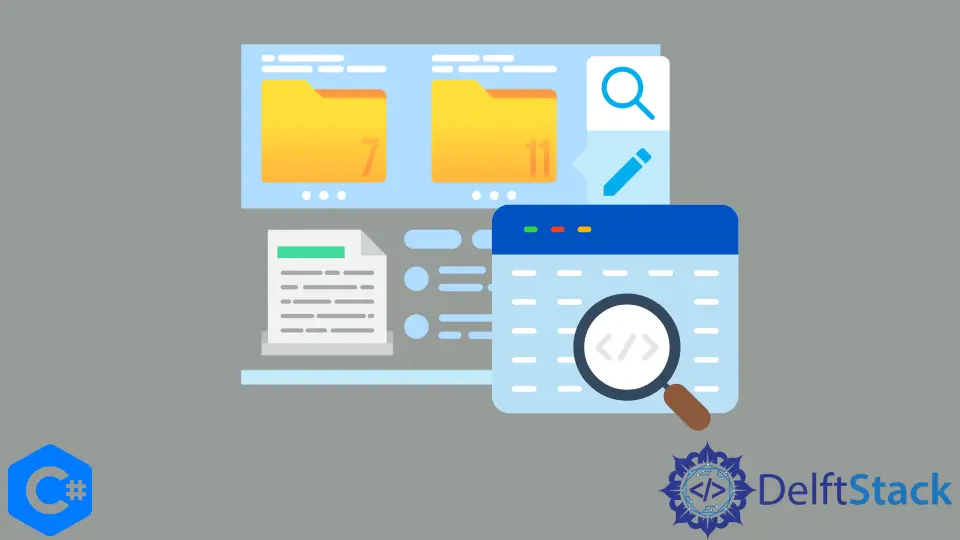
This tutorial will discuss methods to read from and write data to a file in C#.
Write Data to a File With the File.WriteAllText()
Method in C#
The File
class provides functionality for file handling in C#. The File.WriteAllText(path)
method can be used to write some string to a file in the path path
. The following code example shows us how to write data to a file with the File.WriteAllText()
function in C#.
using System;
using System.IO;
namespace write_to_a_file {
class Program {
static void Main(string[] args) {
string path = "C:\\File\\file.txt";
string Text = "Hello, Hi, ByeBye";
File.WriteAllText(path, Text);
}
}
}
file.txt
contents:
Hello, Hi, ByeBye
In the above code, we created a text file file.txt
inside the path C:\File
and wrote the string variable Text
to the file.txt
file with the File.WriteAllText(path, Text)
function in C#.
Read Data From a File With the File.ReadAllText()
Method in C#
The File.ReadAllText()
method can be used to read data from a file in the form of a string variable. The File.ReadAllText()
method takes the path of the file as a parameter and returns the contents of the file in string data type. The following code example shows us how to read data from a file with the File.ReadAllText()
method in C#.
using System;
using System.IO;
namespace write_to_a_file {
class Program {
static void Main(string[] args) {
string path = "C:\\File\\file.txt";
string readText = File.ReadAllText(path);
Console.WriteLine(readText);
}
}
}
Output:
Hello, Hi, ByeBye
In the above code, we read all the data previously written to the file.txt
file inside the C:\File
directory with the File.ReadAllText(path)
method and displayed it to the user.
Write Data to a File With the StreamWriter
Class in C#
The StreamWriter
class is used to write data to a stream in a particular encoding in C#. The StreamWrite.WriteLine()
method can be used to write a string variable to a file. The following code example shows us how to write data to a file with the StreamWriter.WriteLine()
method in C#.
using System;
using System.IO;
namespace write_to_a_file {
class Program {
static void Main(string[] args) {
string path = "C:\\File\\file.txt";
string Text = "Hello and Welcome";
using (StreamWriter writetext = new StreamWriter(path)) {
writetext.WriteLine(Text);
}
}
}
}
file.txt contents:
Hello and Welcome
In the above code, we created an object of the StreamWriter
class and wrote the string variable Text
to the file.txt
file inside the C:\File
directory with the writetext.WriteLine(Text)
function in C#.
Read Data From a File With the StreamReader
Class in C#
The StreamReader
class is used to read data from a stream in a particular encoding in C#. The StreamReader.ReadLine()
method can be used to read string data from a file. The following code example shows us how to read data from a file with the StreamReader.ReadLine()
method in C#.
using System;
using System.IO;
namespace write_to_a_file {
class Program {
static void Main(string[] args) {
string path = "C:\\File\\file.txt";
using (StreamReader readtext = new StreamReader(path)) {
string readText = readtext.ReadLine();
Console.WriteLine(readText);
}
}
}
}
Output:
Hello and Welcome
In the above code, we created an object of the StreamReader
class and read all the data previously written to the file.txt
file inside the C:\File
directory with the readtext.ReadLine()
function and displayed it to the user.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn