用 C# 讀寫檔案
-
在 C# 中使用
File.WriteAllText()
方法將資料寫入檔案 -
使用 C# 中的
File.ReadAllText()
方法從檔案讀取資料 -
在 C# 中使用
StreamWriter
類將資料寫入檔案 -
在 C# 中使用
StreamReader
類從檔案中讀取資料
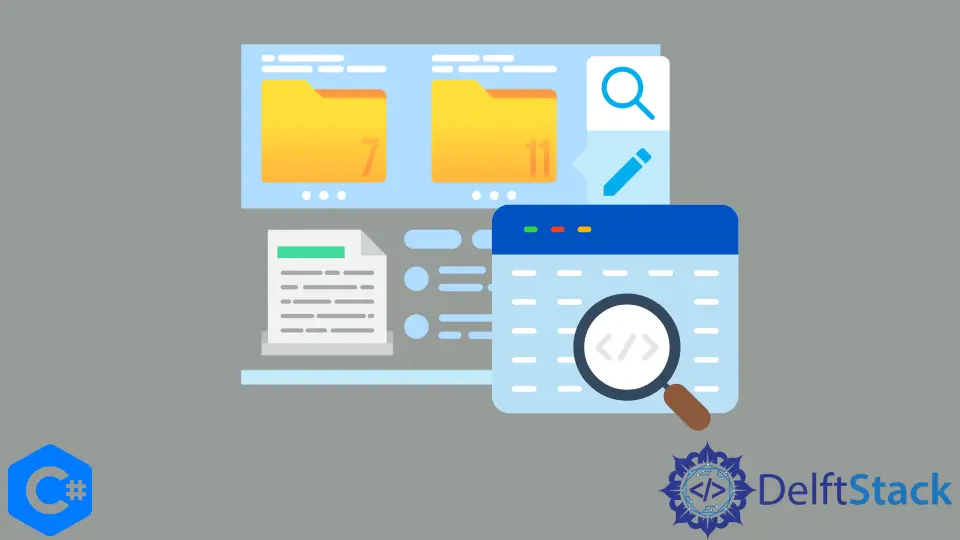
本教程將討論使用 C# 從檔案讀取資料或將資料寫入檔案的方法。
在 C# 中使用 File.WriteAllText()
方法將資料寫入檔案
File
類提供使用 C# 處理檔案的功能。File.WriteAllText(path)
方法可用於在路徑 path
中向檔案中寫入一些字串。以下程式碼示例向我們展示瞭如何使用 C# 中的 File.WriteAllText()
函式將資料寫入檔案。
using System;
using System.IO;
namespace write_to_a_file {
class Program {
static void Main(string[] args) {
string path = "C:\\File\\file.txt";
string Text = "Hello, Hi, ByeBye";
File.WriteAllText(path, Text);
}
}
}
file.txt
內容:
Hello, Hi, ByeBye
在上面的程式碼中,我們在路徑 C:\File
內建立了一個文字檔案 file.txt
,並使用 File.WriteAllText(path, Text)
函式在 C# 中的功能。
使用 C# 中的 File.ReadAllText()
方法從檔案讀取資料
File.ReadAllText()
方法可用於以字串變數的形式從檔案中讀取資料。File.ReadAllText()
方法將檔案的路徑作為引數,並以字串資料型別返回檔案的內容。以下程式碼示例向我們展示瞭如何使用 C# 中的 File.ReadAllText()
方法從檔案讀取資料。
using System;
using System.IO;
namespace write_to_a_file {
class Program {
static void Main(string[] args) {
string path = "C:\\File\\file.txt";
string readText = File.ReadAllText(path);
Console.WriteLine(readText);
}
}
}
輸出:
Hello, Hi, ByeBye
在上面的程式碼中,我們使用 File.ReadAllText(path)
方法讀取了先前寫入 C:\File
目錄內的 file.txt
檔案的所有資料,並將其顯示給使用者。
在 C# 中使用 StreamWriter
類將資料寫入檔案
StreamWriter
類用於以 C# 的特定編碼將資料寫入流。StreamWrite.WriteLine()
方法可用於將字串變數寫入檔案。以下程式碼示例向我們展示瞭如何使用 C# 中的 StreamWriter.WriteLine()
方法將資料寫入檔案。
using System;
using System.IO;
namespace write_to_a_file {
class Program {
static void Main(string[] args) {
string path = "C:\\File\\file.txt";
string Text = "Hello and Welcome";
using (StreamWriter writetext = new StreamWriter(path)) {
writetext.WriteLine(Text);
}
}
}
}
file.txt 內容:
Hello and Welcome
在上面的程式碼中,我們建立了一個 StreamWriter
類的物件,並將字串變數 Text
與 writetext.WriteLine(Text)
一起寫入了 C:\File
目錄內的 file.txt
檔案中。C# 中的函式。
在 C# 中使用 StreamReader
類從檔案中讀取資料
StreamReader
類用於以 C# 的特定編碼從流中讀取資料。StreamReader.ReadLine()
方法可用於從檔案中讀取字串資料。以下程式碼示例向我們展示瞭如何使用 C# 中的 StreamReader.ReadLine()
方法從檔案中讀取資料。
using System;
using System.IO;
namespace write_to_a_file {
class Program {
static void Main(string[] args) {
string path = "C:\\File\\file.txt";
using (StreamReader readtext = new StreamReader(path)) {
string readText = readtext.ReadLine();
Console.WriteLine(readText);
}
}
}
}
輸出:
Hello and Welcome
在上面的程式碼中,我們建立了 StreamReader
類的物件,並使用 readtext.ReadLine()
函式讀取了先前寫入 C:\File
目錄內的 file.txt
檔案的所有資料,並向使用者顯示了它。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn