How to Initialize an Empty Array in C#
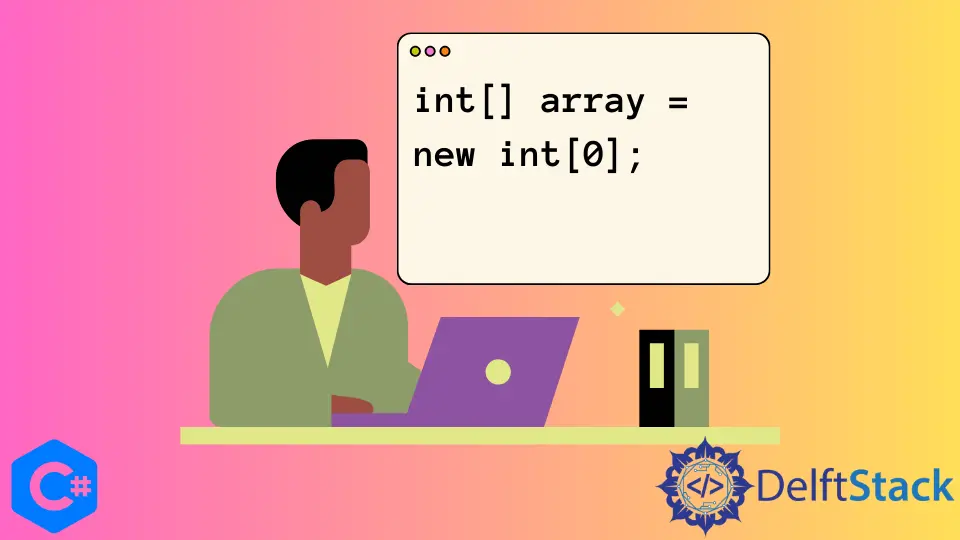
This tutorial will demonstrate how to initialize an array with a known size or an empty array.
An array represents a collection of values that has a fixed length. After setting its size on initialization, you can no longer add or lessen the array. Although you can no longer change the size of an array, you can still change the values of the items within the array. Arrays are beneficial when organizing large amounts of data.
To initialize an array, you can use either of the following examples.
// An array with a size of 5, all values are set to the default value. In this case, a 0 because its
// an integer
int[] array = new int[5];
// An array with all values set. When initializing the array in this way, there is no need to
// specify the size
int[] array_2 = new int[] { 10, 9, 8, 7, 6 };
Since arrays are fixed in length, if you do not know the collection size you’d like to store, you may want to consider other options like List<T>
, which allows you to add as many items as needed. After all items have been added, you can then convert the list to an array by using the ToArray()
function.
List<int> list = new List<int>();
int[] array = list.ToArray();
However, if you genuinely need an empty array, you can set the size of the array to 0.
int[] array = new int[0];
Example:
using System;
namespace StringArray_Example {
class Program {
static void Main(string[] args) {
// Initialize an empty array
int[] empty_array = new int[0];
// Join the values of the array to a single string separated by a comma
string item_str = string.Join(", ", empty_array);
// Print the length of the array
Console.WriteLine("The array has a length of " + empty_array.Length.ToString());
// Print the values of the array
Console.WriteLine("Items: " + item_str);
try {
// Attempt to add a new item to the empty array
empty_array[0] = 1;
} catch (Exception ex) {
Console.WriteLine("You cannot add new items to an array!");
Console.WriteLine("Exception: " + ex.ToString());
}
Console.ReadLine();
}
}
}
In the example above, we declared an empty integer array by setting its size to 0. After printing its values and length to the console to demonstrate that it is indeed empty, we attempted to add a new value to the array. This inevitably failed as you are not allowed to modify the size of an array, thus triggering an Index was outside the bounds of the array
error.
Output:
The array has a length of 0
Items:
You cannot add new items to an array!
Exception: System.IndexOutOfRangeException: Index was outside the bounds of the array.