C# で空の配列を初期化する
Fil Zjazel Romaeus Villegas
2023年10月12日
Csharp
Csharp Array
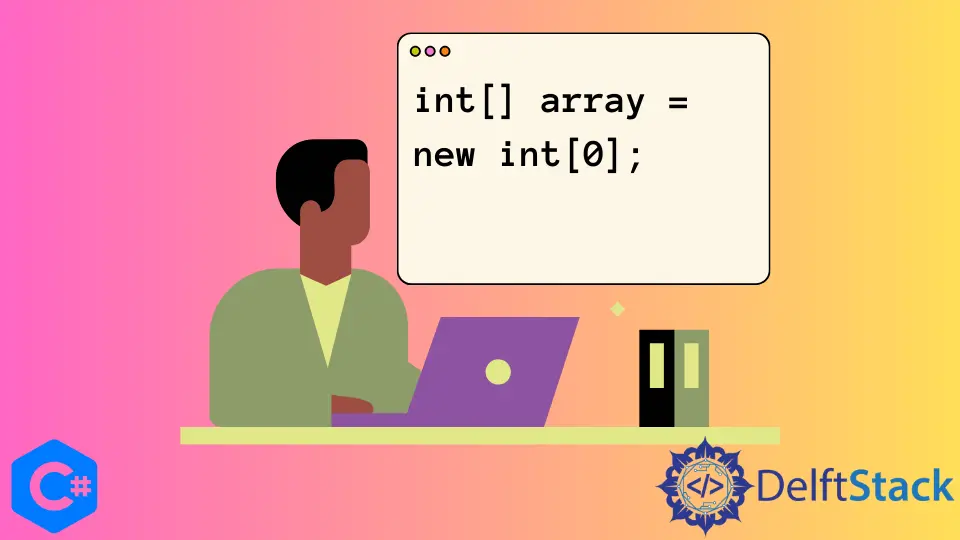
このチュートリアルでは、既知のサイズまたは空の配列で配列を初期化する方法を示します。
配列は、固定長の値のコレクションを表します。初期化時にサイズを設定すると、アレイを追加または縮小できなくなります。配列のサイズを変更することはできなくなりましたが、配列内のアイテムの値を変更することはできます。配列は、大量のデータを整理するときに役立ちます。
アレイを初期化するには、次のいずれかの例を使用できます。
// An array with a size of 5, all values are set to the default value. In this case, a 0 because its
// an integer
int[] array = new int[5];
// An array with all values set. When initializing the array in this way, there is no need to
// specify the size
int[] array_2 = new int[] { 10, 9, 8, 7, 6 };
配列の長さは固定されているため、保存するコレクションサイズがわからない場合は、必要な数のアイテムを追加できる List<T>
などの他のオプションを検討することをお勧めします。すべての項目が追加されたら、ToArray()
関数を使用してリストを配列に変換できます。
List<int> list = new List<int>();
int[] array = list.ToArray();
ただし、本当に空の配列が必要な場合は、配列のサイズを 0 に設定できます。
int[] array = new int[0];
例:
using System;
namespace StringArray_Example {
class Program {
static void Main(string[] args) {
// Initialize an empty array
int[] empty_array = new int[0];
// Join the values of the array to a single string separated by a comma
string item_str = string.Join(", ", empty_array);
// Print the length of the array
Console.WriteLine("The array has a length of " + empty_array.Length.ToString());
// Print the values of the array
Console.WriteLine("Items: " + item_str);
try {
// Attempt to add a new item to the empty array
empty_array[0] = 1;
} catch (Exception ex) {
Console.WriteLine("You cannot add new items to an array!");
Console.WriteLine("Exception: " + ex.ToString());
}
Console.ReadLine();
}
}
}
上記の例では、サイズを 0 に設定して空の整数配列を宣言しました。値と長さをコンソールに出力して実際に空であることを示した後、配列に新しい値を追加しようとしました。配列のサイズを変更することは許可されていないため、これは必然的に失敗し、Index was outside the bounds of the array
エラーが発生します。
出力:
The array has a length of 0
Items:
You cannot add new items to an array!
Exception: System.IndexOutOfRangeException: Index was outside the bounds of the array.
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe