在 C# 中初始化一個空陣列
Fil Zjazel Romaeus Villegas
2023年10月12日
Csharp
Csharp Array
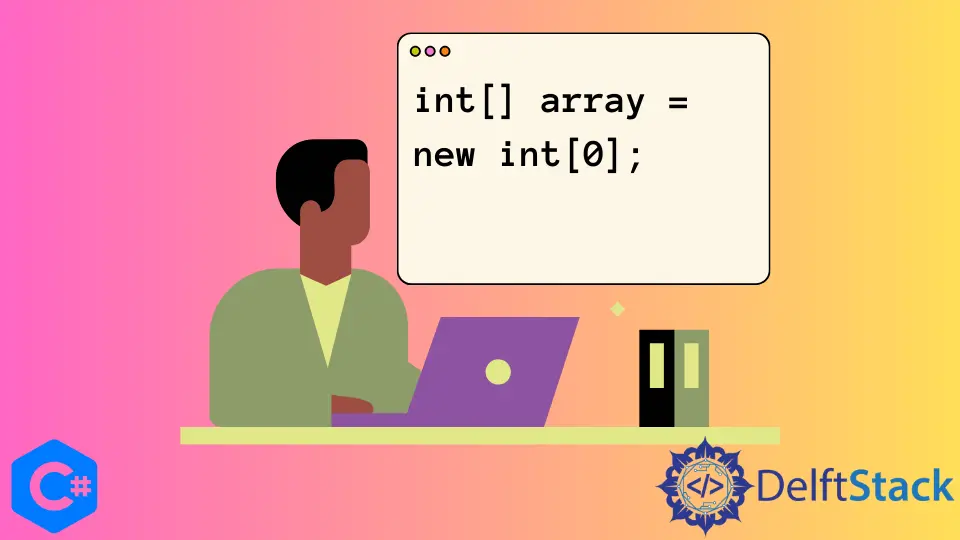
本教程將演示如何初始化一個已知大小的陣列或一個空陣列。
陣列表示具有固定長度的值的集合。在初始化時設定其大小後,你不能再新增或減少陣列。儘管你不能再更改陣列的大小,但你仍然可以更改陣列中各項的值。陣列在組織大量資料時很有用。
要初始化陣列,你可以使用以下任一示例。
// An array with a size of 5, all values are set to the default value. In this case, a 0 because its
// an integer
int[] array = new int[5];
// An array with all values set. When initializing the array in this way, there is no need to
// specify the size
int[] array_2 = new int[] { 10, 9, 8, 7, 6 };
由於陣列的長度是固定的,如果你不知道要儲存的集合大小,你可能需要考慮其他選項,例如 List<T>
,它允許你根據需要新增任意數量的元素。新增完所有元素後,你可以使用 ToArray()
函式將列表轉換為陣列。
List<int> list = new List<int>();
int[] array = list.ToArray();
但是,如果你確實需要一個空陣列,則可以將陣列的大小設定為 0。
int[] array = new int[0];
例子:
using System;
namespace StringArray_Example {
class Program {
static void Main(string[] args) {
// Initialize an empty array
int[] empty_array = new int[0];
// Join the values of the array to a single string separated by a comma
string item_str = string.Join(", ", empty_array);
// Print the length of the array
Console.WriteLine("The array has a length of " + empty_array.Length.ToString());
// Print the values of the array
Console.WriteLine("Items: " + item_str);
try {
// Attempt to add a new item to the empty array
empty_array[0] = 1;
} catch (Exception ex) {
Console.WriteLine("You cannot add new items to an array!");
Console.WriteLine("Exception: " + ex.ToString());
}
Console.ReadLine();
}
}
}
在上面的示例中,我們通過將其大小設定為 0 來宣告一個空整數陣列。在將其值和長度列印到控制檯以證明它確實為空之後,我們嘗試向該陣列新增一個新值。這不可避免地失敗了,因為你不允許修改陣列的大小,從而觸發 Index was outside the bounds of the array
錯誤。
輸出:
The array has a length of 0
Items:
You cannot add new items to an array!
Exception: System.IndexOutOfRangeException: Index was outside the bounds of the array.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe