How to Sort a List by a Property in the Object in C#
-
Use the
OrderBy
Method to Sort a List by a Property in the Object inC#
-
Use a Delegate to Sort a List by a Property in the Object in
C#
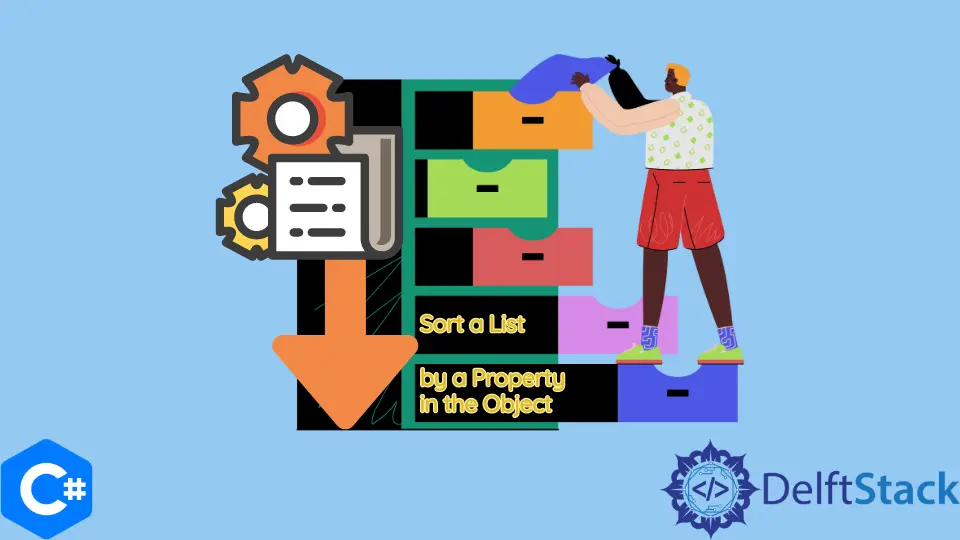
This article will introduce different methods to sort a list by a property in the object using C# code.
- Using the
OrderBy
method - Using
delegate
Use the OrderBy
Method to Sort a List by a Property in the Object in C#
We will use the built-in function OrderBy
to sort a list by a property in the object. It is LINQ’s method. This method creates a new list sorted by the given property. The correct syntax to use this method for sorting a list by a property is as follows.
ListName.Orderby(varName => varName.property);
The built-in function OrderBy
has two parameters. The details of its parameters are as follows.
Parameters | Description | |
---|---|---|
varName |
mandatory | It is the variable name for the given list. |
property |
mandatory | It is the property that we will use to sort our list. |
The program below shows how we can use this function to sort a list by a property in the object.
using System;
using System.Linq;
using System.Collections.Generic;
public class Flowers {
public string name;
public int priority;
public Flowers(string name, int priority) {
this.name = name;
this.priority = priority;
}
public override string ToString() {
return "[" + name + "," + priority + "]";
}
}
public class Example {
public static void Main() {
Flowers hibiscus = new Flowers("Hibiscus", 3);
Flowers rose = new Flowers("Rose", 1);
Flowers lili = new Flowers("Lili", 4);
List<Flowers> mylist = new List<Flowers>() { hibiscus, rose, lili };
List<Flowers> sorted = mylist.OrderBy(x => x.priority).ToList();
Console.WriteLine(String.Join(Environment.NewLine, sorted));
}
}
Output:
[Rose,1]
[Hibiscus,3]
[Lili,4]
Use a Delegate to Sort a List by a Property in the Object in C#
We can also use the custom delegate to sort a list by a property in the object. The correct syntax to use a delegate
is as follows.
[access modifier]
delegate[return type][delegate name]([parameters])
The program below shows how we can use a delegate to sort a list by a property in the object.
using System;
using System.Linq;
using System.Collections.Generic;
public class Flowers {
public string name;
public int priority;
public Flowers(string name, int priority) {
this.name = name;
this.priority = priority;
}
public override string ToString() {
return "[" + name + "," + priority + "]";
}
}
public class Example {
public static void Main() {
Flowers hibiscus = new Flowers("Hibiscus", 3);
Flowers rose = new Flowers("Rose", 1);
Flowers lili = new Flowers("Lili", 4);
List<Flowers> mylist = new List<Flowers>() { hibiscus, rose, lili };
mylist.Sort(delegate(Flowers x, Flowers y) { return x.priority.CompareTo(y.priority); });
Console.WriteLine(String.Join(Environment.NewLine, mylist));
}
}
Output:
[Rose,1]
[Hibiscus,3]
[Lili,4]
Related Article - Csharp Method
- Optional Parameter in C#
- How to Pass a Method as a Parameter in C# Function
- How to Pass Multiple Parameters to a Get Method in C#
- How to Override a Static Method in C#
- Method Group in C#