Optional Parameter in C#
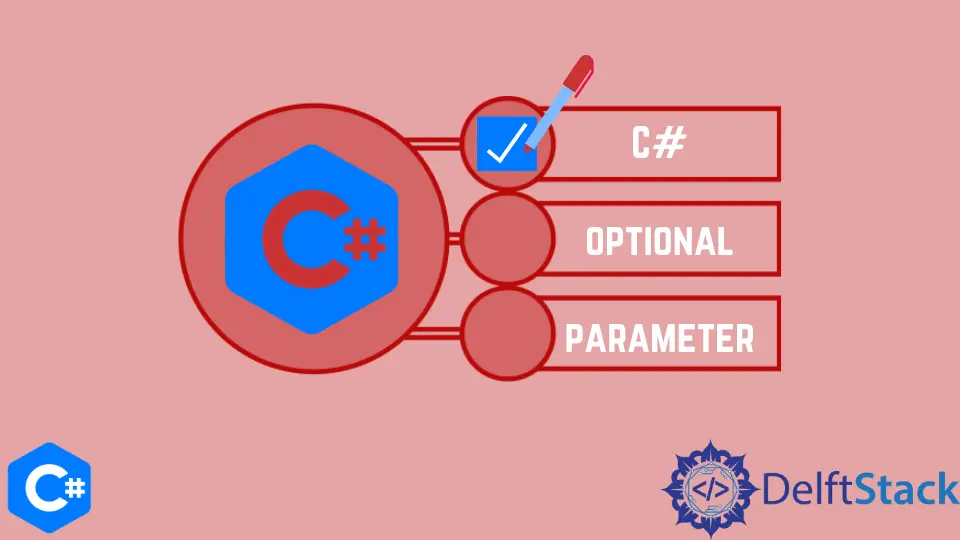
When programming in C#, developers often encounter situations where they need to create methods that can accept a varying number of parameters. This flexibility can significantly enhance the usability of your code.
In this article, we’ll explore how to make method parameters optional in C#. We will dive into three primary approaches: using default values, method overloading, and the Optional attribute. Each method has its unique advantages and use cases, making it essential to understand when to use each technique. By the end of this article, you’ll have a solid grasp of how to implement optional parameters effectively in your C# projects.
Default Value Method
One of the simplest ways to create optional parameters in C# is by using default values. This feature allows you to specify a default value for a parameter in a method signature. If the caller does not provide a value for that parameter, the method will use the default value instead. This approach is straightforward and enhances code readability.
Here’s an example of how to implement a method with default parameter values in C#:
using System;
class Program
{
static void Main()
{
Console.WriteLine(Add(5)); // Calls Add with default value for b
Console.WriteLine(Add(5, 10)); // Calls Add with specified value for b
}
static int Add(int a, int b = 3)
{
return a + b;
}
}
Output:
8
15
In this code, the Add
method takes two parameters: a
and b
. The parameter b
has a default value of 3. When we call Add(5)
, it uses the default value for b
, resulting in 8. If we call Add(5, 10)
, it uses the value of 10 for b
, resulting in 15. This method is particularly useful when you want to provide a sensible default while allowing for flexibility.
Method Overloading
Another approach to creating optional parameters is through method overloading. This technique involves defining multiple methods with the same name but different parameter lists. By providing different versions of a method, you can give users the option to call a method with varying numbers of parameters.
Here’s how you can achieve this in C#:
using System;
class Program
{
static void Main()
{
Console.WriteLine(Add(5)); // Calls the first Add method
Console.WriteLine(Add(5, 10)); // Calls the second Add method
}
static int Add(int a)
{
return a + 3; // Default behavior
}
static int Add(int a, int b)
{
return a + b; // Overloaded method
}
}
Output:
8
15
In the example above, we define two Add
methods: one that takes a single integer and another that takes two integers. The first method adds 3 to the input value, while the second method adds the two input values together. When calling Add(5)
, the first method is invoked, returning 8. When calling Add(5, 10)
, the second method is used, resulting in 15. Method overloading is particularly beneficial when you want to provide different functionalities based on the number of parameters.
Optional Attribute
C# also provides the Optional
attribute, which can be used to specify that a parameter is optional. This attribute allows you to define a method with optional parameters while also specifying their default values. It can be particularly useful in scenarios where you want to maintain backward compatibility.
Here’s an example of how to use the Optional
attribute in C#:
using System;
using System.Runtime.InteropServices;
class Program
{
static void Main()
{
Console.WriteLine(Greet("Alice")); // Calls Greet with default value for greeting
Console.WriteLine(Greet("Bob", "Hi")); // Calls Greet with specified greeting
}
static string Greet(string name, [Optional] string greeting = "Hello")
{
return $"{greeting}, {name}!";
}
}
Output:
Hello, Alice!
Hi, Bob!
In this example, the Greet
method takes two parameters: name
and greeting
, with greeting
being marked as optional. If the caller does not provide a value for greeting
, it defaults to “Hello”. When we call Greet("Alice")
, the method uses the default greeting, resulting in “Hello, Alice!”. If we specify a different greeting, like “Hi”, it uses that instead. The Optional
attribute is handy when you want to maintain flexibility while providing default behaviors.
Conclusion
Understanding how to implement optional parameters in C# can significantly improve the flexibility and usability of your methods. Whether you choose to use default values, method overloading, or the Optional
attribute, each approach offers unique advantages that can be tailored to fit your specific coding needs. By mastering these techniques, you can create cleaner, more efficient code that is easier to maintain and understand.
FAQ
-
What are optional parameters in C#?
Optional parameters allow methods to be called with fewer arguments than defined, using default values when necessary. -
How do default parameters work in C#?
Default parameters are specified in a method’s signature, allowing the method to use a predefined value if the caller does not provide one.
-
Can I overload methods with optional parameters?
Yes, method overloading allows you to define multiple versions of a method with different parameter lists, including optional ones. -
What is the purpose of the Optional attribute in C#?
The Optional attribute indicates that a parameter is optional and can have a default value, providing flexibility in method calls. -
Are there any limitations when using optional parameters in C#?
Optional parameters must be defined at the end of the parameter list, and you cannot have optional parameters before required ones.
#. Learn about default value methods, method overloading, and the Optional attribute. Enhance your C# programming skills by making your methods more flexible and user-friendly. Discover practical examples and detailed explanations to implement these techniques effectively in your projects.
Related Article - Csharp Method
- How to Pass a Method as a Parameter in C# Function
- How to Sort a List by a Property in the Object in C#
- How to Pass Multiple Parameters to a Get Method in C#
- How to Override a Static Method in C#
- Method Group in C#