How to Pass Multiple Parameters to a Get Method in C#
-
Pass Multiple Parameters to a
Get
Method UsingController Action
inC#
-
Code Snippet to Pass Multiple Parameters to a
Get
Method UsingAttribute Routing
-
Code Snippet to Pass Multiple Parameters to a
Get
Method Using[FromQuery]
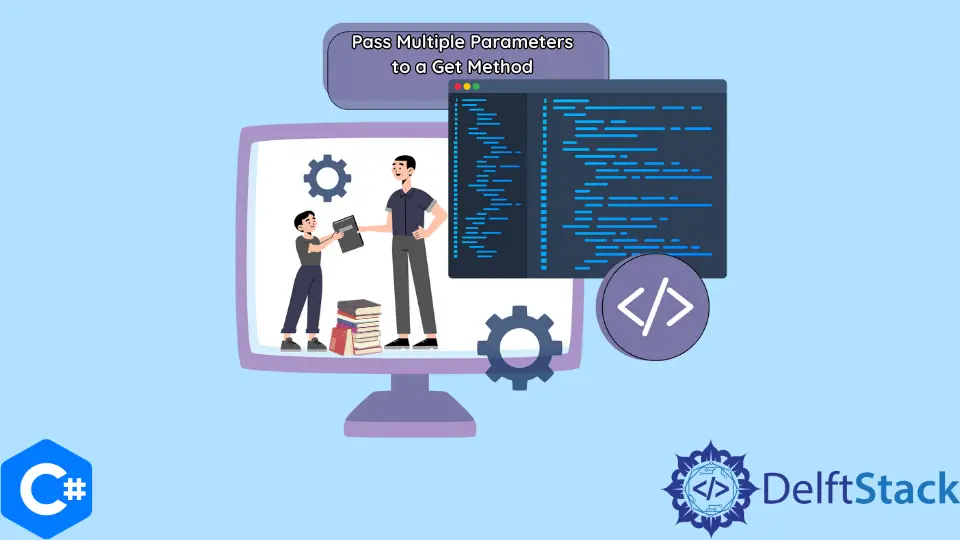
ASP.NET MVC
is used to develop web applications. MVC Web API
and Web pages
framework is now merged into one framework named MVC 6
. MVC
is a pattern that allows you to interact with the model, view, and controller via requests that are routed.
In this article, we are doing to discuss various methods that are used to pass multiple parameters to a Get
method in an MVC controller
.
Pass Multiple Parameters to a Get
Method Using Controller Action
in C#
A controller action
is a method that is used to handle incoming requests. And it returns the action result
. The action result
is the response to the incoming requests. In this case, we have used controller action to pass multiple parameters to a Get
method.
Example Code:
public string Get(int? id, string FirstName, string LastName, string Address) {
if (id.HasValue)
GetById(id);
else if (string.IsNullOrEmpty(Address))
GetByName(FirstName, LastName);
else
GetByNameAddress(FirstName, LastName, Address);
}
Code Snippet to Pass Multiple Parameters to a Get
Method Using Attribute Routing
In attribute routing
, we use attributes
to define routes
. It is a recommended method because it reduces the chances of error. It gives you more control over URLs
in your Web API
.
The correct syntax to use this method is as follows:
// Specify route
[Route("api/YOURCONTROLLER/{parameterOne}/{parameterTwo}")]
public string Get(int parameterOne, int parameterTwo) {
return $"{parameterOne}:{parameterTwo}";
}
}
Example Code:
// Specify route
[Route("api/yourControllerName/{FirstName}/{LastName}/{Address}")]
public string Get(string id, string FirstName, string LastName, string Address) {
return $"{FirstName}:{LastName}:{Address}";
}
Code Snippet to Pass Multiple Parameters to a Get
Method Using [FromQuery]
[FromQuery]
is used to specify that a parameter should be bound with the request query string
. It is the property of the FromQueryAttribute
class.
The correct syntax to use this property is as follows:
[HttpGet]
public string GetByAttribute([FromQuery] string parameterOne, [FromQuery] string parameterTwo) {}
Example Code:
[Route("api/person")]
public class PersonController : Controller {
[HttpGet]
// Passing one parameter to the Get method
public string GetById([FromQuery] int id) {}
[HttpGet]
// Passing two parameters to the Get method
public string GetByName([FromQuery] string firstName, [FromQuery] string lastName) {}
[HttpGet]
// Passing multiple parameters to the Get method
public string GetByNameAndAddress([FromQuery] string firstName, [FromQuery] string lastName,
[FromQuery] string address) {}
}