How to Override a Static Method in C#
- Understanding the Limitations of Static Methods in C#
- Alternate Ways to Override a Static Method in C#
-
Hiding a Static Method With the
new
Keyword in C# - Use a Singleton Pattern as an Alternate Way to Override a Static Method in C#
- Achieving Static Method Override With Dependency Injection in C#
- Conclusion
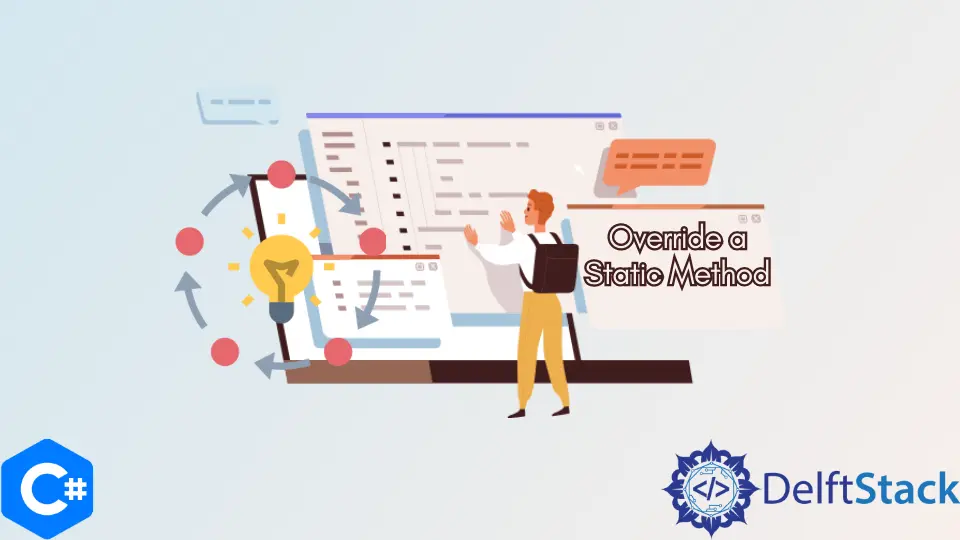
In object-oriented programming, polymorphism allows objects of different types to be treated as objects of a common base type. While method overriding is a fundamental aspect of polymorphism, it’s important to note that static methods do not participate in traditional method overriding in C#.
However, several techniques can be employed to achieve similar functionality. In this article, we will explore different methods for “overriding” static methods in C#, providing detailed explanations and example codes for each approach.
Understanding the Limitations of Static Methods in C#
Before diving into the solutions, it’s crucial to understand why traditional method overriding doesn’t apply to static methods in C#. Static methods are associated with the class rather than instances of the class.
Traditional method overriding is based on polymorphism, where the runtime type of an object determines which method implementation to execute. Since static methods are associated with the class itself, they are bound at compile-time, and their invocation is determined by the reference type, not the runtime type.
We cannot override a static method that belongs to an abstract base class. That is not something that can be done in C#.
This is because the phrase “static” refers to something that applies to everyone, while the overriding idea is what we utilize to change the implementation according to our requirements.
When we override a static method, we lose the static property that comes with it. Consequently, static methods in C# cannot be overridden.
However, we can break the rules and do the task in the opposite order to meet the requirements. Now, let’s explore alternative methods to achieve similar outcomes.
Alternate Ways to Override a Static Method in C#
Hiding is an idea that may be used, and it is possible to use. This idea is also significant to the Early Binding and Late Binding used when building virtual functions.
When virtual method implementations are chosen at runtime based on the instance, then this is called overriding. When more than one method with the same name is chosen at build time, it is known as overloading.
Overloading is more of a convenience than an essential component of object-oriented polymorphism.
Overloading static methods may first resemble overriding. The compiler searches in the inheritance structure until it finds a matching method if it cannot locate a static method in the supplied class.
The method is resolved and fixed at build time, which is a key distinction. Because this is not true overriding, you cannot label static methods as virtual
, override
, or new
.
The behavior of an overriding method should respect the Liskov Substitution Principle (LSP). More generally speaking, the behavior of an overriding method should adhere to the contract of the virtual method.
These constraints do not apply to overloaded static methods, which are more accurately conceived of as distinct methods with the same name but serving different functions. The new
keyword establishes members with the same name but different actions.
When the new
keyword is used, this warning will not appear as it usually would. In addition, bear in mind that you don’t even have to utilize delegates to call the parents’ hidden methods anytime you want; all you have to do is give them their full names.
Hiding a Static Method With the new
Keyword in C#
While the override
keyword is not applicable to static methods, the new
keyword can be used to shadow a base class’s static method in a derived class. This approach is often referred to as method hiding or shadowing.
Example code for shadowing with the new
keyword:
using System;
class BaseClass {
public static void DisplayMessage() {
Console.WriteLine("BaseClass DisplayMessage");
}
}
class DerivedClass : BaseClass {
public new static void DisplayMessage() { Console.WriteLine("DerivedClass DisplayMessage");
}
}
class Program {
static void Main() {
BaseClass.DisplayMessage(); // Output: BaseClass DisplayMessage
DerivedClass.DisplayMessage(); // Output: DerivedClass DisplayMessage
}
}
In this example, the DisplayMessage
method in the DerivedClass
is marked with the new
keyword. This indicates to the compiler that this method is intentionally hiding the method with the same name in the base class.
Output:
BaseClass DisplayMessage
DerivedClass DisplayMessage
The fact that static methods cannot be called on an instance and must be invoked by providing the name of the type to which they belong justifies the practice of hiding them, in our view. The most important decision to make about a method is whether it should be a static method, an instance method, or a virtual method.
The consequence of using the new
term is that the polymorphism cannot be maintained. While it might help reinterpret certain behaviors, we need to use it with great caution and be very cautious about how and where we use it.
Anyone who views the base class while invoking a redefined property will obtain the previous version of the property rather than the current one.
It is important to remember that partial classes cannot be specified across assemblies since this is a restriction imposed by the language.
In an ideal world, you should use them to partition an extensive class into many files (otherwise, you could be doing something incorrectly). You can sometimes use them to share code to facilitate cross-platform development (because not every code works on every platform).
If you are developing for several platforms, in that situation, you may be able to utilize this strategy; however, you should look into alternative ways of modeling your classes.
When partial classes are used, it is common for there to be an extended search for all of the components. This does nothing except make it more difficult for newcomers to comprehend what is happening.
By doing things this way, we can complete the work of overriding a static method of an abstract base class using another way.
Use a Singleton Pattern as an Alternate Way to Override a Static Method in C#
Another approach involves using a Singleton Pattern to encapsulate the static method within an instance. This allows for more dynamic behavior and facilitates unit testing.
The Singleton Pattern is a design pattern that ensures a class has only one instance and provides a global point of access to that instance. While it’s typically used for creating a single instance of a class, it can also be leveraged to achieve a form of static method “overriding”.
Here is an example of the basic syntax for implementing the Singleton Pattern in C#:
public class Singleton {
// Private static instance variable to hold the single instance of the class
private static Singleton _instance;
// Private constructor to prevent external instantiation
private Singleton() {}
// Public method to provide global access to the single instance
public static Singleton Instance {
get {
// Lazy initialization: create the instance if it doesn't exist
if (_instance == null)
_instance = new Singleton();
// Return the single instance
return _instance;
}
}
// Other members and methods of the class can be added here
// ...
// Example method
public void DisplayMessage() {
Console.WriteLine("Singleton Instance DisplayMessage");
}
}
Key elements in the Singleton Pattern:
- Private Static Instance Variable (
_instance
):- This variable holds the single instance of the class.
- It is declared as
private
to prevent external access.
- Private Constructor:
- The constructor is declared as
private
to prevent external instantiation of the class. - This ensures that the class can only be instantiated from within its code.
- The constructor is declared as
- Public Static Property (
Instance
):- This property provides global access to the single instance of the class.
- It uses lazy initialization, meaning the instance is only created if it doesn’t exist yet.
- Singleton Behavior:
- Clients access the singleton instance using the
Instance
property. - The first time it is accessed, the instance is created.
- Subsequent calls return the existing instance.
- Clients access the singleton instance using the
- Example Method (
DisplayMessage
):- This method is just an example to demonstrate that the singleton class can have other members and methods.
Let’s consider an example scenario where we have a base class with a static method, and we want to provide a different implementation of that static method in a derived class.
using System;
public class BaseClass {
public static void DisplayMessage() {
Console.WriteLine("BaseClass DisplayMessage");
}
}
public class SingletonOverride {
private static SingletonOverride _instance;
private SingletonOverride() {}
public static SingletonOverride Instance {
get {
if (_instance == null)
_instance = new SingletonOverride();
return _instance;
}
}
public void DisplayMessage() {
Console.WriteLine("SingletonOverride DisplayMessage");
}
}
class Program {
static void Main() {
BaseClass.DisplayMessage(); // Output: BaseClass DisplayMessage
SingletonOverride.Instance.DisplayMessage(); // Output: SingletonOverride DisplayMessage
}
}
In this example, the SingletonOverride
class serves as a singleton instance that encapsulates the desired behavior for the static method. By creating an instance of SingletonOverride
and calling its DisplayMessage
method, we effectively achieve an alternative form of static method “overriding”.
Output:
BaseClass DisplayMessage
SingletonOverride DisplayMessage
Achieving Static Method Override With Dependency Injection in C#
Consider using dependency injection to provide different implementations of a static-like behavior. This involves injecting a delegate or an interface to achieve a level of abstraction.
Dependency injection involves providing an external dependency to a class rather than letting the class create the dependency itself. In the context of the static method “override”, we can leverage interfaces or delegates to inject alternative implementations dynamically.
-
Define an Interface or Delegate
Create an interface or delegate representing the static-like behavior you want to override. This abstraction serves as a contract for different implementations.
-
Implement Concrete Classes
Create concrete classes that implement the interface or delegate, representing different behaviors.
-
Utilize Dependency Injection
Inject the interface or delegate into classes that need the static-like behavior. This allows you to switch implementations dynamically.
-
Configuration and Usage
Configure the dependency injection container to provide the desired implementation. This can be done at runtime, enabling dynamic behavior.
In this example,
MyClass
receives theIStaticLikeBehavior
interface through its constructor, allowing for flexible behavior based on the injected implementation.
Below is the full code demonstrating the use of dependency injection to achieve a form of “static method override” in C#:
using System;
using System.Collections.Generic;
// Step 1: Define an Interface
public interface IStaticLikeBehavior {
void PerformStaticLikeAction();
}
// Step 2: Implement Concrete Classes
public class OriginalImplementation : IStaticLikeBehavior {
public void PerformStaticLikeAction() {
Console.WriteLine("Original Implementation");
}
}
public class AlternativeImplementation : IStaticLikeBehavior {
public void PerformStaticLikeAction() {
Console.WriteLine("Alternative Implementation");
}
}
// Step 3: Utilize Dependency Injection
public class MyClass {
private readonly IStaticLikeBehavior _staticLikeBehavior;
public MyClass(IStaticLikeBehavior staticLikeBehavior) {
_staticLikeBehavior = staticLikeBehavior;
}
public void Execute() {
_staticLikeBehavior.PerformStaticLikeAction();
}
}
// Step 4: Configuration and Usage
public class DIContainer {
private readonly Dictionary<Type, Type> _registrations = new Dictionary<Type, Type>();
public void Register<TInterface, TImplementation>()
where TImplementation : TInterface {
_registrations[typeof(TInterface)] = typeof(TImplementation);
}
public TInterface Resolve<TInterface>() {
Type implementationType = _registrations[typeof(TInterface)];
return (TInterface)Activator.CreateInstance(implementationType);
}
}
class Program {
static void Main() {
// Configuration
var container = new DIContainer();
container.Register<IStaticLikeBehavior, AlternativeImplementation>();
// Usage
var myClass = new MyClass(container.Resolve<IStaticLikeBehavior>());
myClass.Execute(); // Output: Alternative Implementation
}
}
In this code:
- The
IStaticLikeBehavior
interface defines the contract for the static-like behavior. - Two classes,
OriginalImplementation
andAlternativeImplementation
, implement this interface, providing different behaviors. - The
MyClass
class takes an instance ofIStaticLikeBehavior
through its constructor and utilizes it to perform the static-like action in theExecute
method. - The
DIContainer
class is a simple dependency injection container responsible for registering and resolving types.
In the Main
method, an instance of DIContainer
is used to register AlternativeImplementation
as the implementation for IStaticLikeBehavior
. Then, an instance of MyClass
is created, and the Execute
method is called, resulting in the output "Alternative Implementation"
.
Output:
Alternative Implementation
This showcases the dynamic and flexible nature of dependency injection in achieving a form of “static method override” in C#.
Conclusion
In the realm of object-oriented programming in C#, where static methods do not adhere to traditional method overriding, this comprehensive article explores alternative methods to achieve similar functionality. After establishing the limitations of static methods, three main approaches are discussed:
- Hiding with the
new
Keyword:- Utilizes the
new
keyword to shadow a base class’s static method in a derived class. - Provides an alternative implementation but lacks true polymorphism.
- Utilizes the
- Singleton Pattern as an Alternative:
- Leverages the Singleton Pattern to encapsulate a static method within a singleton instance.
- Allows for dynamic behavior and different implementations.
- Static Method Override with Dependency Injection:
- Utilizes dependency injection to provide different implementations dynamically.
- Defines an interface, creates concrete classes with diverse behaviors, and injects implementations into classes.
- Provides a level of abstraction resembling the static method “override”.
Each method is illustrated with detailed examples and considerations. The article concludes by emphasizing the importance of choosing the appropriate approach based on project requirements and understanding the implications of each method.
Overall, these alternatives empower developers to design more flexible, maintainable, and extensible code in scenarios where true static method overriding is not possible.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn