C# でオブジェクトのプロパティでリストを並べ替える方法
Minahil Noor
2024年2月16日
Csharp
Csharp Method
Csharp Parameter
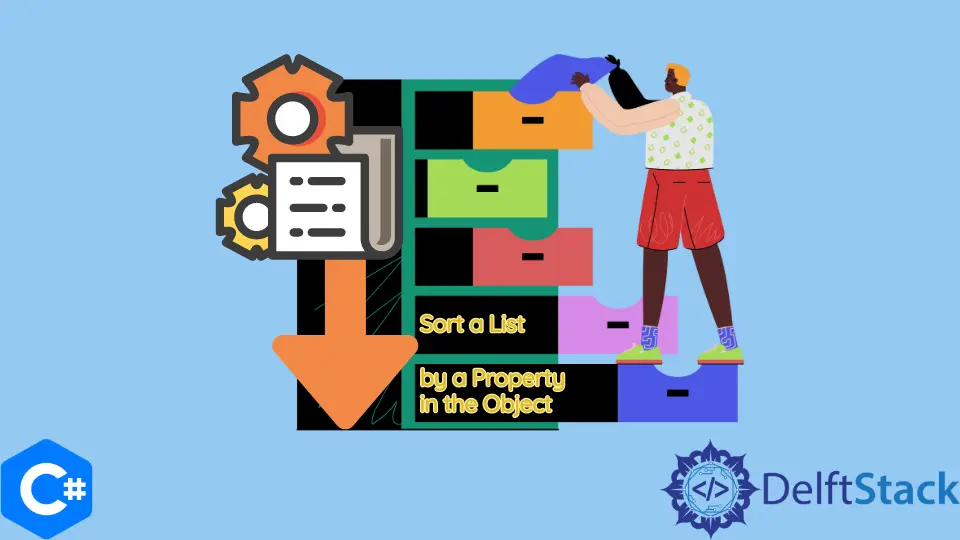
この記事では、C# のコードを使ってオブジェクト内のプロパティでリストを並べ替える方法を紹介します。
OrderBy
メソッドを使用するdelegate
の使用
C# でオブジェクトのプロパティでリストを並べ替えるには OrderBy
メソッドを使用する
オブジェクト内のプロパティでリストをソートするには、組み込み関数 OrderBy
を使用します。これは LINQ のメソッドです。このメソッドは、与えられたプロパティでソートされた新しいリストを作成します。リストをプロパティでソートするためにこのメソッドを使用する正しい構文は以下の通りです。
ListName.Orderby(varName => varName.property);
組み込み関数 OrderBy
は 2つのパラメータを持ちます。パラメータの詳細は以下の通りです。
パラメータ | 説明 | |
---|---|---|
varName |
必須 | これは与えられたリストの変数名です。 |
property |
必須 | これは、リストをソートするために使用するプロパティです。 |
以下のプログラムは、この関数を使用してオブジェクトのプロパティでリストを並べ替える方法を示しています。
using System;
using System.Linq;
using System.Collections.Generic;
public class Flowers {
public string name;
public int priority;
public Flowers(string name, int priority) {
this.name = name;
this.priority = priority;
}
public override string ToString() {
return "[" + name + "," + priority + "]";
}
}
public class Example {
public static void Main() {
Flowers hibiscus = new Flowers("Hibiscus", 3);
Flowers rose = new Flowers("Rose", 1);
Flowers lili = new Flowers("Lili", 4);
List<Flowers> mylist = new List<Flowers>() { hibiscus, rose, lili };
List<Flowers> sorted = mylist.OrderBy(x => x.priority).ToList();
Console.WriteLine(String.Join(Environment.NewLine, sorted));
}
}
出力:
[Rose,1]
[Hibiscus,3]
[Lili,4]
C# でオブジェクト内のプロパティでリストを並べ替えるために delegate
を使用する
また、カスタムデリゲートを使ってオブジェクトのプロパティでリストを並べ替えることもできます。delegate
を使用するための正しい構文は以下の通りです。
[access modifier]
delegate[return type][delegate name]([parameters])
以下のプログラムは、delegate
を使ってオブジェクトのプロパティでリストを並べ替える方法を示しています。
using System;
using System.Linq;
using System.Collections.Generic;
public class Flowers {
public string name;
public int priority;
public Flowers(string name, int priority) {
this.name = name;
this.priority = priority;
}
public override string ToString() {
return "[" + name + "," + priority + "]";
}
}
public class Example {
public static void Main() {
Flowers hibiscus = new Flowers("Hibiscus", 3);
Flowers rose = new Flowers("Rose", 1);
Flowers lili = new Flowers("Lili", 4);
List<Flowers> mylist = new List<Flowers>() { hibiscus, rose, lili };
mylist.Sort(delegate(Flowers x, Flowers y) { return x.priority.CompareTo(y.priority); });
Console.WriteLine(String.Join(Environment.NewLine, mylist));
}
}
出力:
[Rose,1]
[Hibiscus,3]
[Lili,4]
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
関連記事 - Csharp Method
- C# のオプションパラメータ
- C# 関数でメソッドをパラメータとして渡す方法
- C# は get メソッドに複数のパラメーターを渡する
- C# で静的メソッドをオーバーライドする
- C# のメソッドグループ