C#에서 개체의 속성별로 목록을 정렬하는 방법
Minahil Noor
2024년2월16일
Csharp
Csharp Method
Csharp Parameter
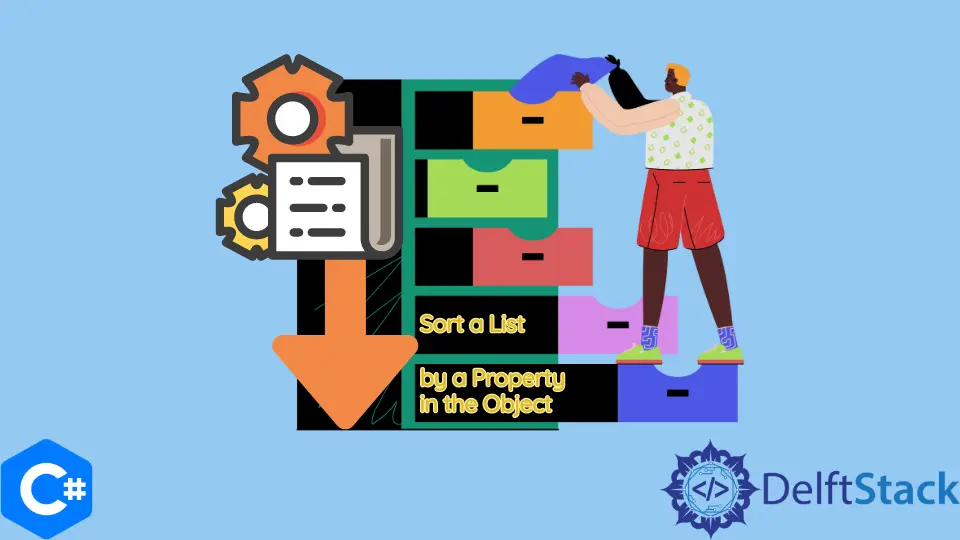
이 문서에서는 C# 코드를 사용하여 개체의 속성별로 목록을 정렬하는 다양한 방법을 소개합니다.
OrderBy
방법 사용- 델리게이트 사용
OrderBy
메서드를 사용하여 C#의 개체에서 속성별로 목록 정렬
기본 제공 함수 OrderBy
를 사용하여 목록을 정렬합니다. 객체의 속성에 의해. LINQ의 방법입니다. 이 메서드는 지정된 속성별로 정렬 된 새 목록을 만듭니다. 속성별로 목록을 정렬하는 데이 방법을 사용하는 올바른 구문은 다음과 같습니다.
ListName.Orderby(varName => varName.property);
내장 함수 OrderBy
에는 두 개의 매개 변수가 있습니다. 매개 변수의 세부 사항은 다음과 같습니다.
매개 변수 | 기술 | |
---|---|---|
varName |
필수 | 주어진 목록의 변수 이름입니다. |
property |
필수 | 목록을 정렬하는 데 사용할 속성입니다. |
아래 프로그램은이 함수를 사용하여 객체의 속성별로 목록을 정렬하는 방법을 보여줍니다.
using System;
using System.Linq;
using System.Collections.Generic;
public class Flowers {
public string name;
public int priority;
public Flowers(string name, int priority) {
this.name = name;
this.priority = priority;
}
public override string ToString() {
return "[" + name + "," + priority + "]";
}
}
public class Example {
public static void Main() {
Flowers hibiscus = new Flowers("Hibiscus", 3);
Flowers rose = new Flowers("Rose", 1);
Flowers lili = new Flowers("Lili", 4);
List<Flowers> mylist = new List<Flowers>() { hibiscus, rose, lili };
List<Flowers> sorted = mylist.OrderBy(x => x.priority).ToList();
Console.WriteLine(String.Join(Environment.NewLine, sorted));
}
}
출력:
[Rose,1]
[Hibiscus,3]
[Lili,4]
대리인을 사용하여 C#에서 개체의 속성별로 목록 정렬
사용자 지정 대리자를 사용하여 개체의 속성별로 목록을 정렬 할 수도 있습니다. delegate
를 사용하는 올바른 구문은 다음과 같습니다.
[access modifier]
delegate[return type][delegate name]([parameters])
아래 프로그램은 델리게이트를 사용하여 객체의 속성별로 목록을 정렬하는 방법을 보여줍니다.
using System;
using System.Linq;
using System.Collections.Generic;
public class Flowers {
public string name;
public int priority;
public Flowers(string name, int priority) {
this.name = name;
this.priority = priority;
}
public override string ToString() {
return "[" + name + "," + priority + "]";
}
}
public class Example {
public static void Main() {
Flowers hibiscus = new Flowers("Hibiscus", 3);
Flowers rose = new Flowers("Rose", 1);
Flowers lili = new Flowers("Lili", 4);
List<Flowers> mylist = new List<Flowers>() { hibiscus, rose, lili };
mylist.Sort(delegate(Flowers x, Flowers y) { return x.priority.CompareTo(y.priority); });
Console.WriteLine(String.Join(Environment.NewLine, mylist));
}
}
출력:
[Rose,1]
[Hibiscus,3]
[Lili,4]
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다