如何在 C# 中按对象中的属性对列表进行排序
Minahil Noor
2023年10月12日
Csharp
Csharp Method
Csharp Parameter
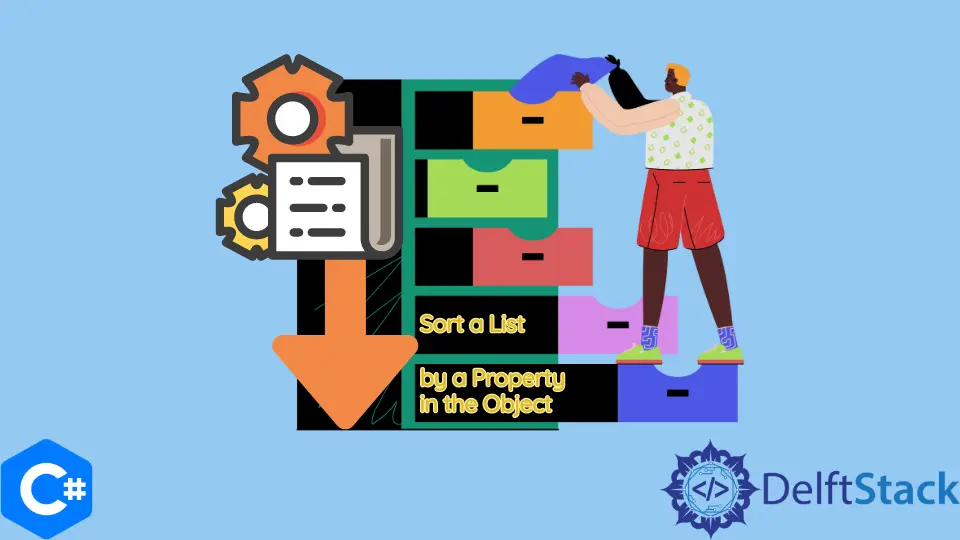
本文将用 C# 代码介绍用对象中的属性对列表进行排序的不同方法。
- 使用
OrderBy
方法 - 使用
delegate
在 C# 中使用 OrderBy
方法通过对象中的属性对列表进行排序
我们将使用内置函数 OrderBy
,通过对象中的一个属性对列表进行排序。这是 LINQ 的方法。这个方法创建一个新的列表,按照给定的属性排序。使用该方法按属性对一个列表进行排序的正确语法如下。
ListName.Orderby(varName => varName.property);
内置函数 OrderBy
有两个参数。它的详细参数如下。
参数 | 说明 | |
---|---|---|
varName |
强制 | 它是给定列表的变量名 |
property |
强制 | 这就是我们将用来给列表排序的属性 |
下面的程序显示了我们如何使用这个函数通过对象中的属性对列表进行排序。
using System;
using System.Linq;
using System.Collections.Generic;
public class Flowers {
public string name;
public int priority;
public Flowers(string name, int priority) {
this.name = name;
this.priority = priority;
}
public override string ToString() {
return "[" + name + "," + priority + "]";
}
}
public class Example {
public static void Main() {
Flowers hibiscus = new Flowers("Hibiscus", 3);
Flowers rose = new Flowers("Rose", 1);
Flowers lili = new Flowers("Lili", 4);
List<Flowers> mylist = new List<Flowers>() { hibiscus, rose, lili };
List<Flowers> sorted = mylist.OrderBy(x => x.priority).ToList();
Console.WriteLine(String.Join(Environment.NewLine, sorted));
}
}
输出:
[Rose,1]
[Hibiscus,3]
[Lili,4]
在 C# 中使用 delegate
通过对象中的一个属性对列表进行排序
我们也可以使用自定义 delegate
来对对象中的某个属性对列表进行排序。使用 delegate
的正确语法如下。
[access modifier]
delegate[return type][delegate name]([parameters])
下面的程序显示了我们如何使用委托来根据对象中的属性对列表进行排序。
using System;
using System.Linq;
using System.Collections.Generic;
public class Flowers {
public string name;
public int priority;
public Flowers(string name, int priority) {
this.name = name;
this.priority = priority;
}
public override string ToString() {
return "[" + name + "," + priority + "]";
}
}
public class Example {
public static void Main() {
Flowers hibiscus = new Flowers("Hibiscus", 3);
Flowers rose = new Flowers("Rose", 1);
Flowers lili = new Flowers("Lili", 4);
List<Flowers> mylist = new List<Flowers>() { hibiscus, rose, lili };
mylist.Sort(delegate(Flowers x, Flowers y) { return x.priority.CompareTo(y.priority); });
Console.WriteLine(String.Join(Environment.NewLine, mylist));
}
}
输出:
[Rose,1]
[Hibiscus,3]
[Lili,4]
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe