How to Get Relative Path of a File in C#
-
Get Relative Path of a File With the
Path
Class inC#
-
Get Relative Path of a File With the
Resources.resx
File inC#
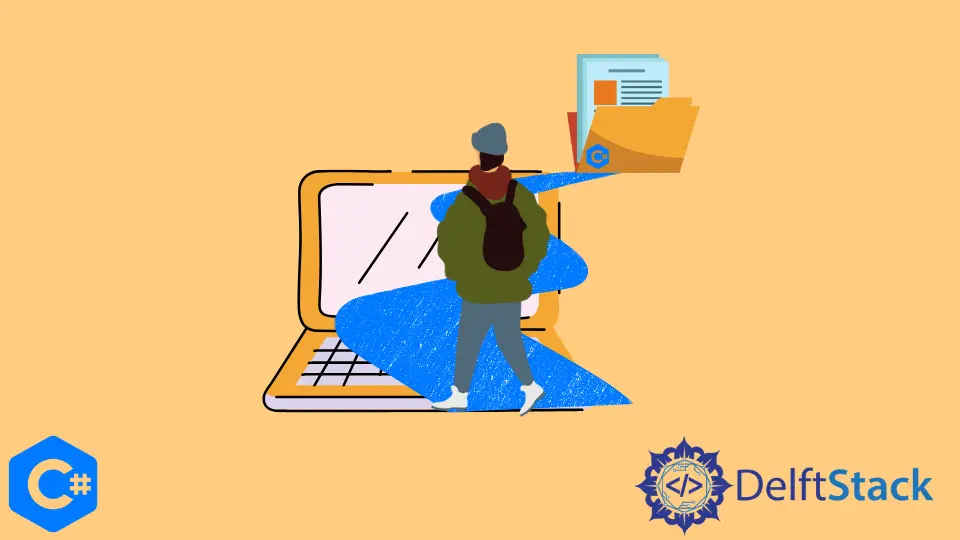
This tutorial will discuss methods to get the relative path of a file in C#.
Get Relative Path of a File With the Path
Class in C#
For this tutorial, I will be talking about the following directory structure.
/ MyProject / Bin Main.exe / Folder Img.jpg Main.cs
When our program executes, it does so from the Main.exe
file inside the Bin
directory. We want to write our code starting from or relative to the Bin
directory. We want to access the Img.jpg
file inside the Folder
directory. This problem can be easily handled by one of the following methods.
The Path
class performs operations on strings variables containing file paths in C#. The Path.GetDirectoryName()
function gets the information about the current directory. We can pass the Assembly.GetExecutingAssembly().CodeBase
as a parameter to the Path.GetDirectoryName()
function to get information about the output directory of our project.
var outPutDirectory = Path.GetDirectoryName(Assembly.GetExecutingAssembly().CodeBase);
We can then combine the resultant path with the short relative path to our Img.jpg
file to create a relative path to our Img.jpg
file.
var iconPath = Path.Combine(outPutDirectory, "Folder\\Img.jpg");
We can then convert this new path to our relative path string with the Uri.LocalPath
property.
string icon_path = new Uri(iconPath).LocalPath;
The following code example shows us how we can perform all the above-mentioned steps in C#.
var outPutDirectory = Path.GetDirectoryName(Assembly.GetExecutingAssembly().CodeBase);
var iconPath = Path.Combine(outPutDirectory, "Folder\\Img.jpg");
string icon_path = new Uri(iconPath).LocalPath;
The string variable icon_path
is now the relative path to the file Img.jpg
and can be used to reference the image throughout our code’s execution.
Get Relative Path of a File With the Resources.resx
File in C#
When we have some external resources that we want to use in our application, such as an image icon, we have to utilize a resources.resx
file in C#. The resources.resx
file works as a record of all the resources our application uses. Once we have added a resources.resx
file in our project and referenced all the required resources, we can easily access all the resources with Resources.Resource1
command. The following code example shows us how we can get a file’s relative path with the resources.resx
file in C#.
using mynamespace.Properties var imgpath = Resources.img;
The imgpath
variable can be used as the reference to the Img.jpg
file throughout our code’s execution in C#. This method should be preferred over the previous method because it is easier to understand, simpler to write, and keeps our code organized.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn