How to Get Executable Path in C#
-
Get Executable Path With the
Assembly
Class inC#
-
Get Executable Path With the
AppDomain
Class inC#
-
Get Executable Path With the
Path
Class inC#
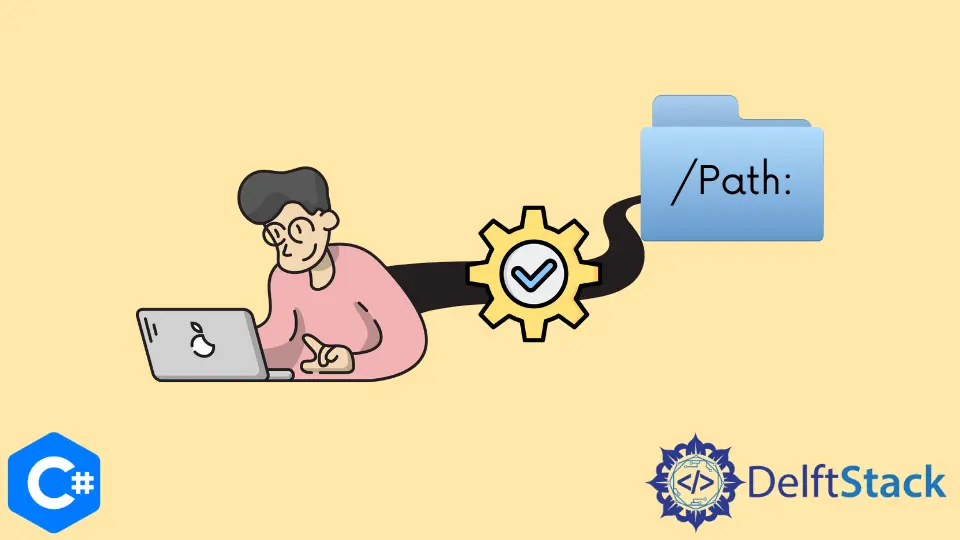
This tutorial will introduce the methods to get the executable path of our code in C#.
Get Executable Path With the Assembly
Class in C#
The Assembly
class represents an assembly that is a reusable building block of a common language runtime CLR application in C#. The Assembly.GetEntryAssembly()
function is used to get the assembly of the currently executing code. We can get the path of the currently executing code with the Assembly.GetEntryAssembly().Location
property which returns the executable path of the current in a string variable. See the following code example.
using System;
using System.IO;
using System.Reflection;
namespace executable_path {
class Program {
static void Main(string[] args) {
string execPath = Assembly.GetEntryAssembly().Location;
Console.WriteLine(execPath);
}
}
}
Output:
C:\Debug\destroy object.exe
In the above code, we displayed our current code’s executable path with the Assembly
class in C#. We stored the value returned by the Assembly.GetEntryAssembly().Location
property inside the string variable execPath
and displayed it to the user.
Get Executable Path With the AppDomain
Class in C#
The above method gives us the path along with the name of the executable file. If we want to get the name of the directory without the executable file’s name, we can use the AppDomain
class in C#. The AppDomain
class represents an application domain. An application domain is an isolated environment where applications execute. The AppDomain.CurrentDomain
property gets the application domain of the current application. We can get the executable path with the AppDomain.CurrentDomain.BaseDirectory
property, which returns the directory’s path containing the executable file of the current code file in a string variable. The following code example shows us how we can get our current code’s executable path with the AppDomain
class in C#.
using System;
using System.IO;
using System.Reflection;
namespace executable_path {
class Program {
static void Main(string[] args) {
string execPath = AppDomain.CurrentDomain.BaseDirectory;
Console.WriteLine(execPath);
}
}
}
Output:
C:\Debug\
In the above code, we displayed our current code’s executable path with the AppDomain
class in C#. We stored the value returned by the AppDomain.CurrentDomain.BaseDirectory
property inside the string variable execPath
and displayed it to the user. This approach is recommended because it is the easiest and requires less code than the other two approaches.
Get Executable Path With the Path
Class in C#
The Path
class performs various operations on strings containing file paths in C#. The Path.GetDirectoryName()
function can get information of the directory specified by the path. We can use the System.Reflection.Assembly.GetExecutingAssembly().CodeBase
property as an argument of the Path.GetDirectoryName()
function to get the name of the directory containing the current code. See the following code example.
using System;
using System.IO;
using System.Reflection;
namespace executable_path {
class Program {
static void Main(string[] args) {
string execPath =
Path.GetDirectoryName(System.Reflection.Assembly.GetExecutingAssembly().CodeBase);
Console.WriteLine(execPath);
}
}
}
Output:
file:\C:\Users\Maisam\source\repos\executable path\executable path\bin\Debug
In the above code, we displayed our current code’s executable path with the Path
class in C#. We stored the value returned by the Path.GetDirectoryName()
function inside the string variable execPath
and displayed it to the user. This approach returns the path with the file:\
string at the start of the path. This approach is not recommended because it takes more code than the previous two approaches.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn