在 C# 中獲取可執行路徑
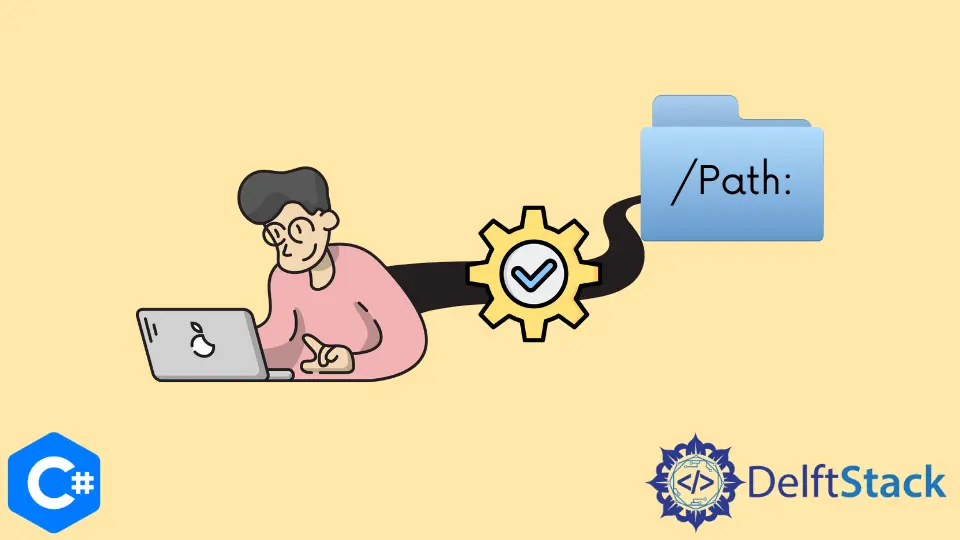
本教程將介紹獲取 C# 程式碼的可執行路徑的方法。
使用 C# 中的 Assembly
類獲取可執行路徑
Assembly
類表示一個程式集,它是 C# 中通用語言執行時 CLR 應用程式的可重用構建塊。Assembly.GetEntryAssembly()
函式用於獲取當前執行程式碼的彙編。我們可以使用 Assembly.GetEntryAssembly().Location
屬性獲取當前正在執行的程式碼的路徑,該屬性以字串變數的形式返回當前的可執行路徑。請參見以下程式碼示例。
using System;
using System.IO;
using System.Reflection;
namespace executable_path {
class Program {
static void Main(string[] args) {
string execPath = Assembly.GetEntryAssembly().Location;
Console.WriteLine(execPath);
}
}
}
輸出:
C:\Debug\destroy object.exe
在上面的程式碼中,我們使用 C# 中的 Assembly
類顯示了當前程式碼的可執行路徑。我們將 Assembly.GetEntryAssembly().Location
屬性返回的值儲存在字串變數 execPath
內,並顯示給使用者。
使用 C# 中的 AppDomain
類獲取可執行路徑
上面的方法為我們提供了路徑以及可執行檔案的名稱。如果要獲取沒有可執行檔名稱的目錄名稱,則可以使用 C# 中的 AppDomain
類。AppDomain
類表示一個應用程式域。應用程式域是在其中執行應用程式的隔離環境。AppDomain.CurrentDomain
屬性獲取當前應用程式的應用程式域。我們可以使用 AppDomain.CurrentDomain.BaseDirectory
屬性獲取可執行檔案路徑,該屬性返回目錄路徑,該路徑包含當前程式碼檔案的可執行檔案(字串變數)。以下程式碼示例向我們展示瞭如何使用 C# 中的 AppDomain
類獲取當前程式碼的可執行路徑。
using System;
using System.IO;
using System.Reflection;
namespace executable_path {
class Program {
static void Main(string[] args) {
string execPath = AppDomain.CurrentDomain.BaseDirectory;
Console.WriteLine(execPath);
}
}
}
輸出:
C:\Debug\
在上面的程式碼中,我們使用 C# 中的 AppDomain
類顯示了當前程式碼的可執行路徑。我們將 AppDomain.CurrentDomain.BaseDirectory
屬性返回的值儲存在字串變數 execPath
內,並將其顯示給使用者。建議使用此方法,因為與其他兩種方法相比,它最簡單且所需的程式碼更少。
使用 C# 中的 Path
類獲取可執行路徑
Path
類對包含 C# 中檔案路徑的字串執行各種操作。Path.GetDirectoryName()
函式可以獲取路徑指定的目錄資訊。我們可以將 System.Reflection.Assembly.GetExecutingAssembly().CodeBase
屬性用作 Path.GetDirectoryName()
函式的引數,以獲取包含當前程式碼的目錄的名稱。請參見以下程式碼示例。
using System;
using System.IO;
using System.Reflection;
namespace executable_path {
class Program {
static void Main(string[] args) {
string execPath =
Path.GetDirectoryName(System.Reflection.Assembly.GetExecutingAssembly().CodeBase);
Console.WriteLine(execPath);
}
}
}
輸出:
file:\C:\Users\Maisam\source\repos\executable path\executable path\bin\Debug
在上面的程式碼中,我們使用 C# 中的 Path
類顯示了當前程式碼的可執行路徑。我們將 Path.GetDirectoryName()
函式返回的值儲存在字串變數 execPath
內,並顯示給使用者。此方法以路徑開頭的字串 file:\
返回路徑。不建議使用此方法,因為與前兩種方法相比,它需要更多的程式碼。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn