How to Get Dictionary Key by Value in C#
-
Get a Dictionary Key by Value Using the
foreach
Loop in C# - Get a Dictionary Key by Value Using LINQ in C#
- Get a Dictionary Key by Value Using a Reverse Dictionary in C#
-
Get a Dictionary Key by Value Using
EqualityComparer<>.Default.Equals()
in C# - Conclusion
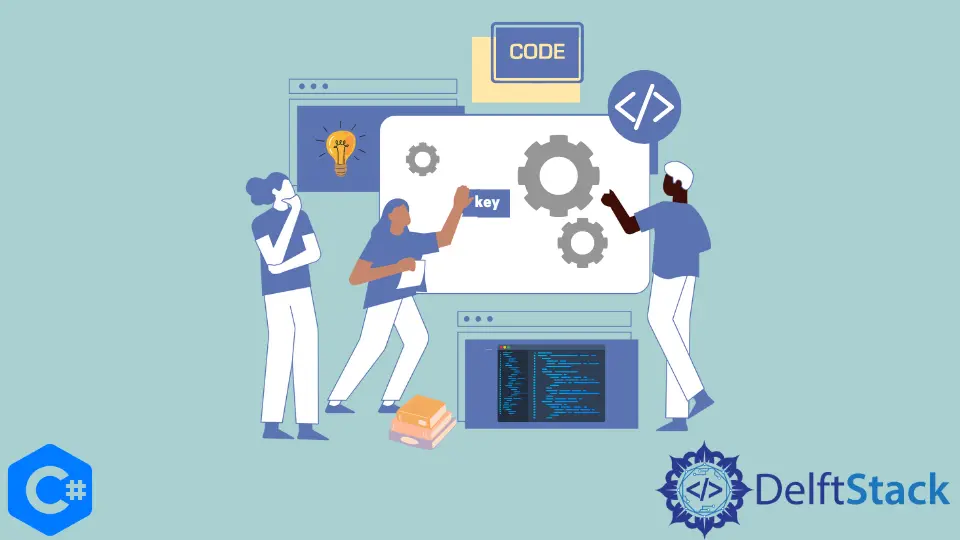
Dictionaries in C# are versatile data structures, providing a quick and efficient way to store key-value pairs. Occasionally, you may find yourself needing to retrieve a dictionary key based on its corresponding value.
In this article, we’ll explore various approaches to accomplish this task, offering you different methods to choose from based on your specific needs.
Get a Dictionary Key by Value Using the foreach
Loop in C#
While dictionaries provide an efficient way to store key-value pairs, there is no built-in method for directly obtaining a dictionary key based on its associated value. However, a common and straightforward approach to achieve this is by utilizing the foreach
loop.
The foreach
loop simplifies the process of iterating through each element in a collection, making it well-suited for dictionary traversal. In the context of obtaining a key by value, we iterate through each key-value pair in the dictionary.
By using an if
statement within the loop, we can compare the values until a match is found, allowing us to capture the corresponding key.
The following code example shows us how to get a dictionary key by value using the foreach
loop in C#.
using System;
using System.Collections.Generic;
class Program {
static void Main() {
Dictionary<string, string> types =
new Dictionary<string, string> { { "1", "one" }, { "2", "two" }, { "3", "three" } };
string targetValue = "two";
string foundKey = "";
foreach (var pair in types) {
if (pair.Value == targetValue) {
foundKey = pair.Key;
break;
}
}
Console.WriteLine("Key: " + foundKey);
}
}
In this example, we start by creating a dictionary named types
with string keys and values, and we specify the targetValue
we want to find the key for, in this case, two
. We initialize an empty string variable foundKey
to store the result.
The foreach
loop iterates through each key-value pair in the types
dictionary. Inside the loop, the if
statement checks if the current pair’s value matches the targetValue
.
If a match is found, we assign the key (pair.Key
) to the foundKey
variable and exit the loop using break
. Finally, we print the found key to the console.
Output:
Key: 2
In this output, we successfully obtained the key 2
corresponding to the value two
using the foreach
loop. This method is effective for scenarios where a simple iteration is sufficient, providing a clear and concise way to get a dictionary key by its value in C#.
Get a Dictionary Key by Value Using LINQ in C#
While the foreach
loop provides a practical method for obtaining a dictionary key by its corresponding value, LINQ (Language Integrated Query) in C# offers a more concise and expressive solution. LINQ allows us to integrate SQL-like queries directly into the language, simplifying the process of searching for a key based on a specific value in a dictionary.
In this approach, we can leverage the FirstOrDefault()
method provided by LINQ to find the first key-value pair in the dictionary that satisfies a given condition. Here is the syntax of the FirstOrDefault
method:
public static TSource FirstOrDefault<TSource>(this IEnumerable<TSource> source,
Func<TSource, bool> predicate);
Where:
source
: TheIEnumerable<TSource>
to return the first element from.predicate
: A function to test each element for a condition.
Specifically, we can use a lambda expression within FirstOrDefault()
to check if the value of the current pair matches our target value. This method is not only concise but also ensures that the search stops as soon as a matching pair is found, improving efficiency.
See the following code example:
using System;
using System.Collections.Generic;
using System.Linq;
class Program {
static void Main() {
Dictionary<string, string> types =
new Dictionary<string, string> { { "1", "one" }, { "2", "two" }, { "3", "three" } };
string targetValue = "three";
var foundKey = types.FirstOrDefault(x => x.Value == targetValue).Key;
Console.WriteLine("Key: " + foundKey);
}
}
Here, we create the same dictionary, types
, with string keys and values. Similar to the foreach
loop approach, we define the targetValue
we want to find the key for, in this case, three
.
The LINQ expression FirstOrDefault(x => x.Value == targetValue)
is employed to find the first key-value pair in the types
dictionary where the value matches the specified targetValue
. The result is stored in the foundKey
variable.
Output:
Key: 3
In this output, we successfully obtained the key 3
corresponding to the value three
using LINQ. The LINQ approach provides a more concise and expressive solution for scenarios where you prefer a declarative and query-like syntax.
Get a Dictionary Key by Value Using a Reverse Dictionary in C#
In addition to the foreach
loop and LINQ methods, another interesting approach for obtaining a dictionary key by its corresponding value is by creating a reverse dictionary. This method involves creating a new dictionary where the values become keys, and the keys become values.
The idea behind the reverse dictionary approach is to trade memory for faster lookup times. By creating a dictionary where values become keys, searching for a key based on a value becomes a constant time operation.
This can be particularly useful if such lookups are frequent and performance is a critical factor.
Let’s illustrate this with an example:
using System;
using System.Collections.Generic;
using System.Linq;
class Program {
static void Main() {
Dictionary<string, string> types =
new Dictionary<string, string> { { "1", "one" }, { "2", "two" }, { "3", "three" } };
Dictionary<string, string> reverseDictionary = types.ToDictionary(x => x.Value, x => x.Key);
string targetValue = "two";
string foundKey = reverseDictionary[targetValue];
Console.WriteLine("Key: " + foundKey);
}
}
To implement the reverse dictionary approach, we use the LINQ ToDictionary()
method to create a new dictionary named reverseDictionary
. This new dictionary swaps the keys and values of the original dictionary, where the original values become keys, and the original keys become values.
This reverse dictionary essentially allows for direct lookups based on values.
We then specify the targetValue
we want to find the key for, in this case, two
. Using the reverseDictionary
, we perform a direct lookup by accessing the value as a key, and the result is stored in the foundKey
variable.
Output:
Key: 2
In this output, the reverse dictionary approach successfully obtained the key 2
corresponding to the value two
. While this method may consume more memory due to the creation of the reverse dictionary, it provides a swift and efficient solution for frequent lookups based on values in a dictionary.
Get a Dictionary Key by Value Using EqualityComparer<>.Default.Equals()
in C#
Another approach for obtaining a dictionary key based on its corresponding value involves using the EqualityComparer<>.Default.Equals()
method. This method is particularly useful when dealing with complex types or situations where the default equality comparison might not suffice.
The EqualityComparer<>.Default.Equals()
method is a generic method provided by the EqualityComparer<T>
class in C#. It allows for comparing two values for equality, utilizing the default equality comparer for the type.
This method is often used in scenarios where custom equality logic is required. Its syntax is as follows:
bool Equals(T x, T y);
Here, x
and y
are the values to compare for equality.
See the following code example:
using System;
using System.Collections.Generic;
class Program {
static void Main() {
Dictionary<string, string> types =
new Dictionary<string, string> { { "1", "one" }, { "2", "two" }, { "3", "three" } };
string targetValue = "one";
string foundKey = GetKeyByValue(types, targetValue);
Console.WriteLine("Key: " + foundKey);
}
static TKey GetKeyByValue<TKey, TValue>(Dictionary<TKey, TValue> dictionary, TValue targetValue) {
foreach (var pair in dictionary) {
if (EqualityComparer<TValue>.Default.Equals(pair.Value, targetValue)) {
return pair.Key;
}
}
throw new KeyNotFoundException($"Key not found for value: {targetValue}");
}
}
In this example, a Dictionary<string, string>
named types
is created, containing key-value pairs representing numerical strings and their corresponding word representations. The target value to search for is set to one
.
The method GetKeyByValue
is introduced to encapsulate the key retrieval logic. This method takes a generic dictionary and a target value as parameters.
Within a foreach
loop, each key-value pair is checked using EqualityComparer<TValue>.Default.Equals()
to determine if the current value matches the target value. If a match is found, the corresponding key is returned.
If the loop completes without finding a matching key, a KeyNotFoundException
is thrown, indicating that the specified value was not found in the dictionary.
Output:
Key: 1
In this output, the code successfully obtained the key 1
corresponding to the value one
using the EqualityComparer<>.Default.Equals()
method. This approach is valuable when dealing with custom types or scenarios where a more nuanced equality comparison is required.
Conclusion
In summary, the choice of method depends on factors such as simplicity, performance, and the nature of your data. The foreach
loop is straightforward, LINQ provides expressive querying, a reverse dictionary offers efficient lookups, and EqualityComparer<>.Default.Equals()
accommodates custom equality comparisons.
Choose the method that aligns with your requirements and coding style, ensuring a seamless experience in retrieving dictionary keys by values in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn