How to Get All File Names in a Directory in C#
-
Get All File Names in a Directory With the
Directory.GetFiles()
Method in C# -
Get All File Names in a Directory With the
DirectoryInfo.GetFiles()
Method in C# -
Get All File Names in a Directory With the
Directory.EnumerateFiles
Method in C# - Conclusion
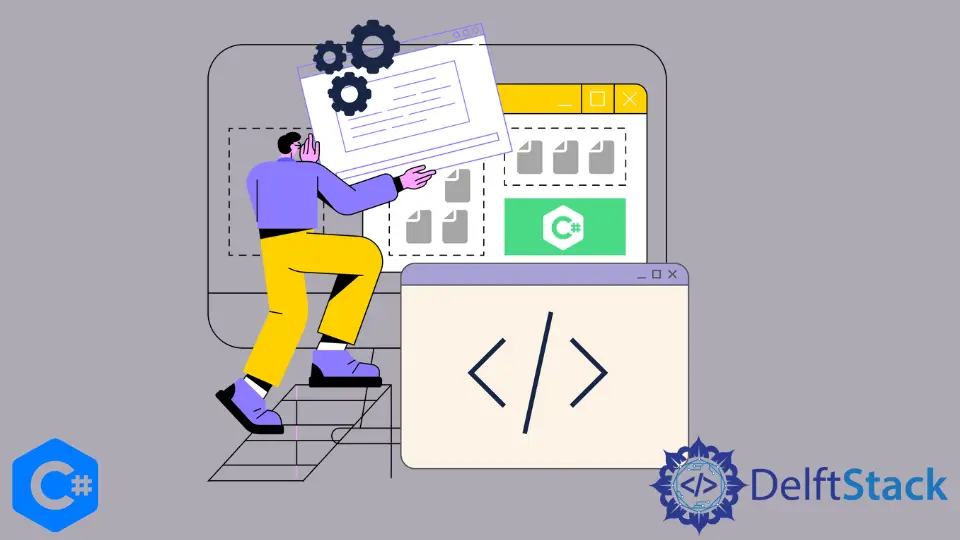
Retrieving file names from a directory is a common task in C# programming, essential for various file management and processing tasks. Among the different methods available, the Directory.GetFiles()
method provides a straightforward approach to accomplish this task efficiently.
This article explores different methods for getting file names in a directory using C#, focusing on the Directory.GetFiles()
method and alternative approaches such as DirectoryInfo.GetFiles()
and Directory.EnumerateFiles()
.
Get All File Names in a Directory With the Directory.GetFiles()
Method in C#
The Directory.GetFiles()
method in C# gets the names of all the files inside a specific directory. The Directory.GetFiles()
method returns an array of strings that contains the absolute paths of all the files inside the directory specified in the method parameters.
Example:
using System;
using System.IO;
class Program {
static void Main() {
string directoryPath = @"Folder";
string[] fileNames = Directory.GetFiles(directoryPath);
Console.WriteLine("Files in directory:");
foreach (string fileName in fileNames) {
Console.WriteLine(fileName);
}
}
}
In the provided code example, we specify the directory path where we want to retrieve the file names using the directoryPath
variable. We then call the Directory.GetFiles()
method, passing the directory path as an argument.
This method returns an array of strings containing the names of all files in the specified directory. Once we have the array of file names, we iterate over it using a foreach
loop to print each file name to the console.
Output:
Get All File Names With Specific File Extension in a Directory With the Directory.GetFiles()
Method in C#
In C#, the Directory.GetFiles()
method offers a convenient way to retrieve file names from a directory based on specific criteria, such as file extensions. This method allows developers to filter files within a directory by providing a search pattern parameter, enabling the retrieval of files that match the specified pattern.
Example:
using System;
using System.IO;
namespace get_all_files {
class Program {
static void Main(string[] args) {
string[] files = Directory.GetFiles(@"Folder", "*.txt");
foreach (var file in files) {
Console.WriteLine(file);
}
}
}
}
In the provided code example, we utilize the Directory.GetFiles()
method to retrieve file names from the Folder
directory with the *.txt
search pattern, indicating that we only want to retrieve files with a .txt
extension.
The method returns an array of strings containing the names of all matching files, which we then iterate over using a foreach
loop to print each file name to the console. This approach efficiently filters the files based on the specified criteria, ensuring that only files with the desired extension are included in the result.
Output:
Get All File Names Including Subdirectories in a Directory With the Directory.GetFiles()
Method in C#
In C#, the Directory.GetFiles()
method offers a powerful capability to not only retrieve file names from a specific directory but also search for files recursively within all subdirectories. This feature is invaluable when dealing with complex directory structures where files may be nested within multiple levels of subdirectories.
By incorporating the SearchOption.AllDirectories
parameter, developers can efficiently gather file names from both the specified directory and all its subdirectories, streamlining file management and exploration tasks within C# applications.
Example:
using System;
using System.IO;
namespace get_all_files {
class Program {
static void Main(string[] args) {
string[] files = Directory.GetFiles(@"Folder", "*.txt", SearchOption.AllDirectories);
foreach (var file in files) {
Console.WriteLine(file);
}
}
}
}
In the provided code example, we utilize the Directory.GetFiles()
method with the SearchOption.AllDirectories
parameter to retrieve file names from the Folder
directory and all its subdirectories. This enables us to gather a comprehensive list of all text files (*.txt
) present within the directory hierarchy.
By iterating over the array of file names using a foreach
loop, we print each file name to the console, allowing us to visualize the entire file structure encompassing the specified directory and its subdirectories.
Output:
Get All File Names in a Directory With the DirectoryInfo.GetFiles()
Method in C#
The DirectoryInfo.GetFiles()
method gets the files inside a specified directory in C#. The DirectoryInfo.GetFiles()
method returns an array of the FileInfo
class objects that contain information about all the files in the directory specified inside the method parameters.
Example:
using System;
using System.IO;
namespace get_all_files {
class Program {
static void Main(string[] args) {
DirectoryInfo di = new DirectoryInfo(@"Folder");
FileInfo[] files = di.GetFiles("*.txt");
foreach (FileInfo file in files) {
Console.WriteLine(file.Name);
}
}
}
}
In the provided code example, we instantiate a DirectoryInfo
object di
with the directory path Folder
. We then use the GetFiles()
method of the DirectoryInfo
class to retrieve an array of FileInfo
objects representing all text files (*.txt
) within the directory.
By iterating over the array of FileInfo
objects using a foreach
loop, we print each file name (file.Name
) to the console. This approach provides a structured and object-oriented way to obtain file names within a directory, offering access to additional file metadata if needed.
Output:
Get All File Names in a Directory With the Directory.EnumerateFiles
Method in C#
In C#, the Directory.EnumerateFiles()
method provides a convenient and efficient way to retrieve file names within a directory. Unlike the Directory.GetFiles()
method, which immediately returns an array of file names, Directory.EnumerateFiles()
returns an enumerable collection of file names as needed, which can be iterated over using a foreach
loop.
Example:
using System;
using System.IO;
using System.Collections.Generic;
class Program {
static void Main(string[] args) {
string directoryPath = @"Folder";
IEnumerable<string> fileNames = Directory.EnumerateFiles(directoryPath);
Console.WriteLine("Files in directory:");
foreach (string fileName in fileNames) {
Console.WriteLine(fileName);
}
}
}
In the provided code example, we use the Directory.EnumerateFiles()
method to retrieve an enumerable collection of file names from the Folder
directory. This method returns file names as needed rather than loading all file names into memory at once, resulting in better performance and memory efficiency, especially for directories with a large number of files.
By iterating over the enumerable collection of file names using a foreach
loop, we print each file name to the console, providing a comprehensive list of files in the directory.
Output:
Conclusion
Understanding the diverse methods for retrieving file names in a directory is pivotal for effective file management in C# applications. While the Directory.GetFiles()
method serves as a reliable choice for straightforward file retrieval, alternatives like DirectoryInfo.GetFiles()
and Directory.EnumerateFiles()
provide additional capabilities, such as accessing file metadata and improving memory efficiency.
By strategically choosing the most suitable method for each scenario, developers can optimize their code for performance and scalability. Additionally, familiarity with these methods empowers developers to handle various file-related tasks efficiently, from simple file enumeration to complex directory traversals.
Ultimately, mastering these techniques enhances the overall robustness and efficiency of C# applications, ensuring smooth file handling and streamlined operations in diverse software projects.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn