C#에서 디렉터리의 모든 파일 이름 가져 오기
-
C#에서
Directory.GetFiles()
메서드를 사용하여 디렉터리의 모든 파일 이름 가져 오기 -
C#에서
DirectoryInfo.GetFiles()
메서드를 사용하여 디렉터리의 모든 파일 이름 가져 오기
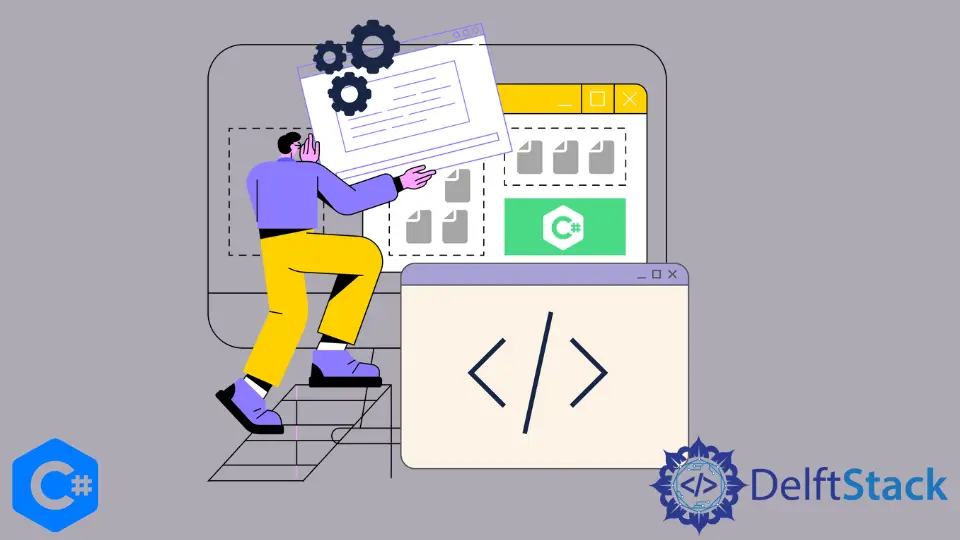
이 자습서에서는 C#의 특정 디렉터리에있는 모든 파일의 파일 이름을 가져 오는 방법에 대해 설명합니다.
C#에서Directory.GetFiles()
메서드를 사용하여 디렉터리의 모든 파일 이름 가져 오기
C#의 Directory.GetFiles()
메소드는 특정 디렉토리에있는 모든 파일의 이름을 가져옵니다. Directory.GetFiles()
메소드는 메소드 매개 변수에 지정된 디렉토리 내에있는 모든 파일의 절대 경로를 포함하는 문자열 배열을 리턴합니다. 다음 예를 참조하십시오.
using System;
using System.IO;
namespace get_all_files {
class Program {
static void Main(string[] args) {
string[] files = Directory.GetFiles(@"C:\File", "*.txt");
foreach (var file in files) {
Console.WriteLine(file);
}
}
}
}
출력:
C:\File\file.txt
C:\File\file1.txt
위의 코드에서 C#의Directory.GetFiles()
메소드를 사용하여C:\File
디렉토리 내에.txt
확장자를 가진 모든 파일의 이름을 추출했습니다.
Directory.GetFiles()
메소드는 다양한 용도로 사용할 수 있습니다. 예를 들어 다음 코드 예제는 C#의 파일 확장자에 관계없이 모든 파일의 이름을 제공합니다.
using System;
using System.IO;
namespace get_all_files {
class Program {
static void Main(string[] args) {
string[] files = Directory.GetFiles(@"C:\File");
foreach (var file in files) {
Console.WriteLine(file);
}
}
}
}
출력:
C:\File\file.txt
C:\File\file1.txt
C:\File\readme.md
Directory.GetFiles()
메소드를 사용하여 모든 하위 디렉토리에 포함 된 모든 파일의 파일 이름을 가져올 수도 있습니다. 다음 예를 참조하십시오.
using System;
using System.IO;
namespace get_all_files {
class Program {
static void Main(string[] args) {
string[] files = Directory.GetFiles(@"C:\File", "*.txt", SearchOption.AllDirectories);
foreach (var file in files) {
Console.WriteLine(file);
}
}
}
}
출력:
C:\File\file.txt
C:\File\file1.txt
C:\File\subFile\file3.txt
C:\File\subFile\file4.txt
위 코드에서 C#의Directory.GetFiles()
메소드를 사용하여C:\File
디렉토리의 모든 하위 디렉토리에서 확장자가.txt
인 모든 파일의 파일 이름을 추출했습니다.
C#에서DirectoryInfo.GetFiles()
메서드를 사용하여 디렉터리의 모든 파일 이름 가져 오기
DirectoryInfo.GetFiles()
메소드는 C#의 지정된 디렉토리에있는 파일을 가져옵니다. DirectoryInfo.GetFiles()
메소드는 메소드 매개 변수 내에 지정된 디렉토리에있는 모든 파일에 대한 정보를 포함하는FileInfo
클래스 오브젝트의 배열을 리턴합니다. FileInfo.Name
속성을 사용하여 파일 이름을 가져올 수 있습니다. 다음 코드 예제는 C#의DirectoryInfo.GetFiles()
메소드를 사용하여 지정된 디렉토리 내에서 파일의 파일 이름을 얻는 방법을 보여줍니다.
using System;
using System.IO;
namespace get_all_files {
class Program {
static void Main(string[] args) {
DirectoryInfo di = new DirectoryInfo(@"C:\File");
FileInfo[] files = di.GetFiles("*.txt");
string str = "";
foreach (FileInfo file in files) {
Console.WriteLine(file.Name);
}
}
}
}
출력:
file.txt
file1.txt
위 코드에서 C#의DirectoryInfo.GetFiles()
메소드를 사용하여C:\File
디렉토리 내에.txt
확장자를 가진 모든 파일의 파일 이름을 추출했습니다. 또한DirectoryInfo.GetFiles()
메소드 내에서SearchOption.AllDirectories
매개 변수를 사용하여 모든 하위 디렉토리에있는 모든 파일의 이름을 가져올 수 있습니다. 한편,DirectoryInfo.GetFiles()
메소드에 파일 확장자를 지정하지 않음으로써 파일 확장자에 관계없이 모든 파일의 이름을 가져올 수도 있습니다. 이전 접근법과이 접근법의 유일한 차이점은Directory.GetFiles()
메소드가 파일의 완전한 절대 경로를 제공한다는 것입니다. 반대로DirectoryInfo.GetFiles()
메소드는 모든 파일의 이름 만 제공합니다.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn