How to Generate Random Alphanumeric Strings in C#
-
Generate Random Alphanumeric Strings With the
Random
Class inC#
-
Generate Random Alphanumeric Strings With the LINQ Method in
C#
-
Generate Random Alphanumeric Strings With the
RNGCryptoServiceProvider
Class inC#
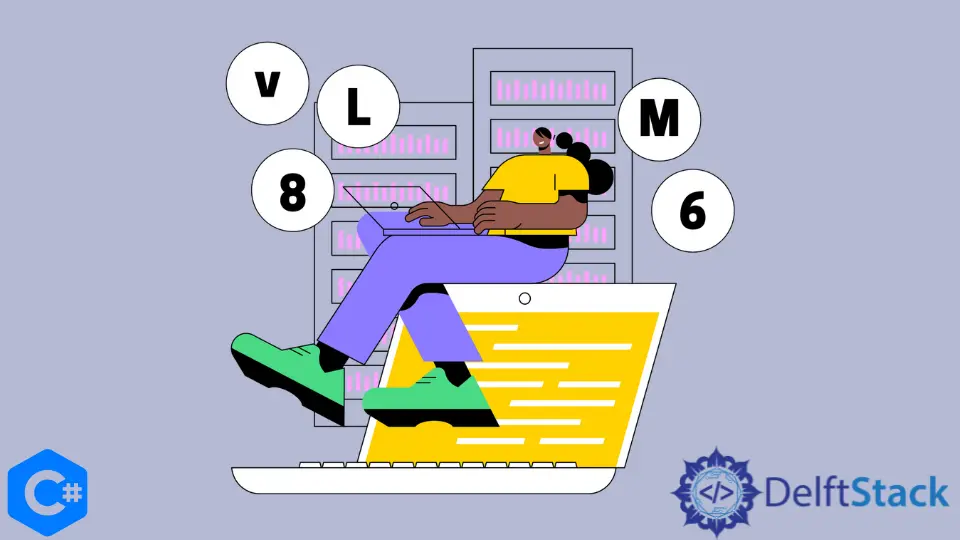
This tutorial will discuss the methods to generate a random alphanumeric string in C#.
Generate Random Alphanumeric Strings With the Random
Class in C#
The Random
class generates random numbers in C#. The Random.Next()
method generates a random integer value. We can declare a constant string variable containing all the alphanumeric characters and choose characters from the string variable based on the index generated by the Random.Next()
method.
The following code example shows us how to generate a random alphanumeric string with the Random
class in C#.
using System;
using System.Linq;
namespace random_alphanumeric_strings {
class Program {
static void Main(string[] args) {
var characters = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789";
var Charsarr = new char[8];
var random = new Random();
for (int i = 0; i < Charsarr.Length; i++) {
Charsarr[i] = characters[random.Next(characters.Length)];
}
var resultString = new String(Charsarr);
Console.WriteLine(resultString);
}
}
}
Output:
vL8M6BNr
We generated a random alphanumeric string of 8 characters length with the Random
class in C#. Then we initialized the string characters
containing all the desired alphanumeric characters. We created the array of characters Charsarr
with a length of 8
characters. We then initialized an instance of the Random
class random
. We iterated through the Charsarr
array and saved the character at a random index from the characters
string in Charsarr
each time. We then converted the array of characters Charsarr
to the string variable resultString
and displayed the result.
Generate Random Alphanumeric Strings With the LINQ Method in C#
The LINQ performs query functionality on data structures in C#. We can use the LINQ with the Random
class to improve the previous method’s performance. The following code example shows us how to generate a random alphanumeric string with the LINQ method in C#.
using System;
using System.Linq;
namespace random_alphanumeric_strings {
class Program {
private static Random random = new Random();
public static string method2(int length) {
const string characters = "ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789";
return new string(
Enumerable.Repeat(characters, length).Select(s => s[random.Next(s.Length)]).ToArray());
}
static void Main(string[] args) {
string finalString = method2(8);
Console.WriteLine(finalString);
}
}
}
Output:
SXUXK2P8
We created a random alphanumeric string with LINQ and the Random
class in C# in the above code. This method is more efficient than the method discussed above.
Generate Random Alphanumeric Strings With the RNGCryptoServiceProvider
Class in C#
The methods discussed above are not recommended for generating passwords because they are not really random and follow a linear pattern. For secure passwords, the RNGCryptoServiceProvider
class should be utilized. The RNGCryptoServiceProvider
class generates cryptographic random numbers in C#. The GetBytes()
method of the RNGCryptoServiceProvider
class fills a bytes array with random values. We can use the GetBytes()
method with the Convert.ToBase64String()
method to get a string out of the filled bytes array. The following code example shows us how to generate a secure random alphanumeric string with the RNGCryptoServiceProvider
class in C#.
using System;
using System.Linq;
using System.Security.Cryptography;
namespace random_alphanumeric_strings {
class Program {
static string method3(int length) {
using (var crypto = new RNGCryptoServiceProvider()) {
var bits = (length * 6);
var byte_size = ((bits + 7) / 8);
var bytesarray = new byte[byte_size];
crypto.GetBytes(bytesarray);
return Convert.ToBase64String(bytesarray);
}
}
static void Main(string[] args) {
string finalString = method3(8);
Console.WriteLine(finalString);
}
}
}
Output:
JGc42Ug3
We generated a secure random alphanumeric string with 8 characters with the RNGCryptoServiceProvider
class in C# in the above code. This method is recommended if we want to generate random passwords because it is relatively secure and unpredictable than the previous two methods.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn