How to Format a String to Currency in C#
-
Format a String to Currency With the
String.Format()
Method inC#
-
Format a String to Currency With the
ToString()
Method inC#
-
Comparing
String.Format()
andToString()
for Currency Formatting in C# - Conclusion
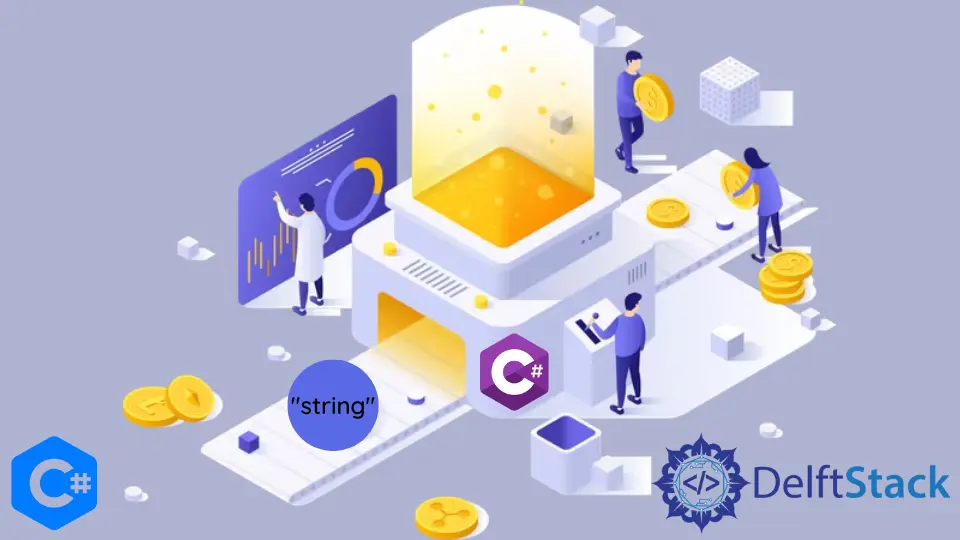
This tutorial will discuss the methods of formatting a string variable to currency in C#.
Format a String to Currency With the String.Format()
Method in C#
One of the most straightforward methods to format a string as currency in C# is by using the String.Format()
method. This method is versatile, allowing for the incorporation of format specifiers to tailor currency formatting according to specific requirements. It shines in its ability to provide both default and culture-specific currency representations. In this section, we explore how String.Format()
can be effectively utilized to produce precise and culturally aware currency formats in your C# applications.
Complete Working Code
Let’s start by examining a comprehensive example:
using System;
using System.Globalization;
class Program {
static void Main() {
decimal amount = 1234.56m; // Example amount
// Default currency formatting
string formattedCurrencyDefault = string.Format("{0:C}", amount);
Console.WriteLine("Default Format: " + formattedCurrencyDefault);
// US-specific currency formatting
CultureInfo usCulture = new CultureInfo("en-US");
string formattedCurrencyUS = string.Format(usCulture, "{0:C}", amount);
Console.WriteLine("US Format: " + formattedCurrencyUS);
}
}
Output:
$1,234.56
Step-by-Step Code Explanation
1. Setting Up the Environment
using System;
using System.Globalization;
This code imports essential namespaces: System
for basic functionality and System.Globalization
for culture-specific formatting.
2. Defining the Amount
decimal amount = 1234.56m;
We define a decimal variable amount
that represents the monetary value we wish to format.
3. Default Currency Formatting
string formattedCurrencyDefault = string.Format("{0:C}", amount);
Console.WriteLine("Default Format: " + formattedCurrencyDefault);
Here, String.Format
with {0:C}
formats amount
as currency using the system’s default cultural setting. The formatted string is then displayed.
4. Culture-Specific Currency Formatting
CultureInfo usCulture = new CultureInfo("en-US");
string formattedCurrencyUS = string.Format(usCulture, "{0:C}", amount);
Console.WriteLine("US Format: " + formattedCurrencyUS);
In this snippet, we instantiate a CultureInfo
object for the United States and pass it to String.Format
along with the same format specifier. This tailors the currency format to U.S. standards, demonstrating the method’s flexibility in adapting to different cultural norms.
By using String.Format()
, developers can seamlessly integrate both standard and localized currency formats into their C# applications, ensuring a user-friendly and culturally sensitive display of monetary values.
Format a String to Currency With the ToString()
Method in C#
The ToString()
method in C# stands as a versatile and essential tool for formatting strings, especially for currency conversion. It offers a straightforward approach to transforming numeric values into currency formats. In this section, we explore the ToString()
method’s capacity for both standard and culture-specific currency formatting, highlighting its role in presenting financial data with precision and adaptability in C# applications.
Complete Working Code
Here’s a complete example to illustrate the use of ToString()
for currency formatting:
using System;
using System.Globalization;
class Program {
static void Main() {
decimal amount = 1234.56m; // Example amount
// Default currency formatting
string formattedCurrency = amount.ToString("C");
Console.WriteLine("Default Format: " + formattedCurrency);
// US-specific currency formatting
CultureInfo usCulture = new CultureInfo("en-US");
string formattedCurrencyUS = amount.ToString("C", usCulture);
Console.WriteLine("US Format: " + formattedCurrencyUS);
}
}
Output:
$1,234.56
Step-by-Step Code Explanation
1. Importing Required Namespaces
using System;
using System.Globalization;
The System
namespace is included for fundamental types, while System.Globalization
is used for accessing culture-specific information, critical for currency formatting.
2. Specifying the Amount
decimal amount = 1234.56m;
A decimal variable, amount
, is declared to represent the monetary value to be formatted.
3. Applying Default Currency Formatting
string formattedCurrency = amount.ToString("C");
Console.WriteLine("Default Format: " + formattedCurrency);
The ToString
method with the "C"
format specifier is used to convert amount
into a currency string using the default cultural settings. The result is output to the console.
4. Implementing Culture-Specific Currency Formatting
CultureInfo usCulture = new CultureInfo("en-US");
string formattedCurrencyUS = amount.ToString("C", usCulture);
Console.WriteLine("US Format: " + formattedCurrencyUS);
A CultureInfo
object for the U.S. is instantiated to apply U.S.-specific currency formatting. The ToString
method is then used with this culture object to format the currency accordingly, demonstrating the method’s flexibility in adapting to various cultural norms.
The ToString()
method in C# simplifies the task of formatting numbers as currency, offering developers a reliable tool to handle both general and culture-specific requirements. This functionality is essential for applications dealing with financial data, ensuring accurate and user-friendly displays of currency values.
Comparing String.Format()
and ToString()
for Currency Formatting in C#
In C# programming, both String.Format()
and ToString()
are valuable for formatting currency, but they differ in application and convenience. Here’s a concise comparison:
Flexibility and Application
String.Format()
excels in scenarios requiring multiple variables or complex string constructions. It’s particularly useful when formatting several values within a single string or when integrating additional text alongside the formatted currency. This method allows for a clear separation of format and data, which can enhance code maintainability.ToString()
is more straightforward, ideal for converting a single numeric value. Its simplicity and direct approach make it a preferred choice for simple currency formatting tasks, especially when dealing with individual values.
Culture-Specific Formatting
Both methods support culture-specific formatting, essential for applications targeting global audiences. String.Format()
takes the CultureInfo
object as the first argument, conveniently applying the same cultural format to multiple values. In contrast, ToString()
applies culture as a secondary argument, intuitive for single-value conversions.
Readability and Performance
String.Format()
might seem complex initially but provides a clear distinction between data and its presentation, a boon for code updates and readability.ToString()
is more readable due to its simplicity, making it a go-to for quick formatting needs. Performance-wise, it’s marginally more efficient for straightforward tasks, whereasString.Format()
could be slightly more demanding due to its parsing mechanism.
Conclusion
In this article, we’ve explored the String.Format()
and ToString()
methods in C# for currency formatting. String.Format()
is ideal for complex scenarios involving multiple variables, offering flexibility and detailed control. In contrast, ToString()
excels in straightforward, single-value conversions with its simplicity and direct approach. Both methods cater to different needs, ensuring accurate and culturally appropriate currency representations in C# applications.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn