How to Format a String as a Telephone Number in C#
-
Format a String as a Telephone Number in C# Using a
formatPhNumber()
Custom Method -
Format a String as a Telephone Number in C# Using
String.Format
- Format a String as a Telephone Number in C# Using String Interpolation
- Conclusion
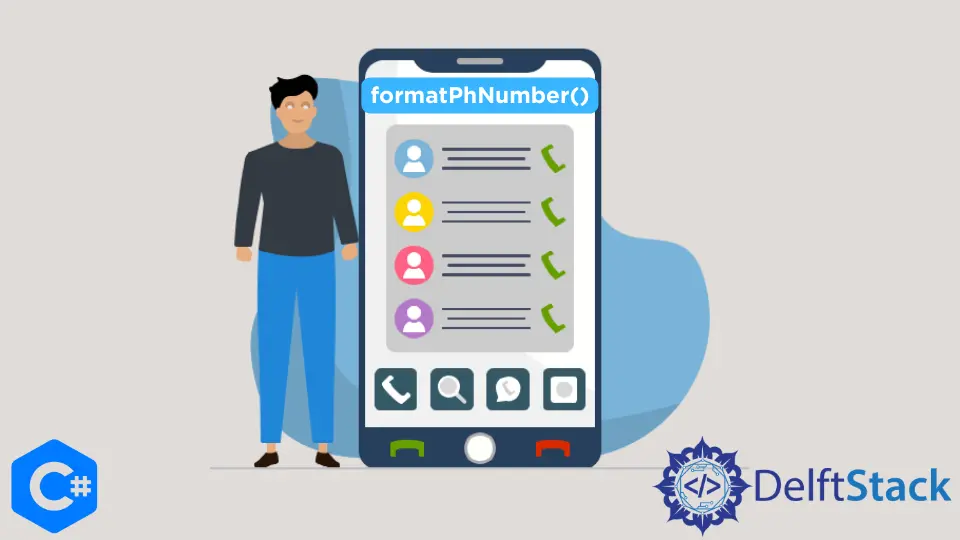
Formatting a string as a telephone number is a common task in programming, often required for displaying user-friendly information or validating user inputs. In C#, there are multiple methods to achieve this, each with its syntax and advantages.
In this article, we’ll explore three different methods: using the String.Format
function, employing string interpolation, and implementing a custom method (formatPhNumber()
).
Format a String as a Telephone Number in C# Using a formatPhNumber()
Custom Method
In this section, we will discuss how to format a string as a telephone number in C# by using the formatPhNumber()
custom method. This method will take a phone number and a format string as input, remove non-numeric characters, and apply the specified format.
We begin by importing the necessary libraries, including System
and System.Text.RegularExpressions
. The formatPhNumber()
method is then defined within a class named PhoneFormatter
.
This method takes two string parameters: phoneNum
(representing the phone number to be formatted) and phoneFormat
(indicating the desired format).
using System;
using System.Text.RegularExpressions;
class PhoneFormatter {
public static string formatPhNumber(string phoneNum, string phoneFormat) {
// Method implementation goes here
return phoneNum;
}
static void Main() {
// Main method content
}
}
Inside the formatPhNumber()
method, we first check if the phoneFormat
parameter is empty. If so, we assign it a default phone number format, which is (##) ###-####
.
We then utilize a regular expression (Regex
) to remove any non-numeric characters from the provided phoneNum
.
if (phoneFormat == "") {
phoneFormat = "(##) ###-####";
}
Regex regex = new Regex(@"[^\d]");
phoneNum = regex.Replace(phoneNum, "");
Following this, we check if the cleaned phoneNum
has a length greater than zero. If true, we convert the phone number to a 64-bit integer and then format it using the specified or default format.
if (phoneNum.Length > 0) {
phoneNum = Convert.ToInt64(phoneNum).ToString(phoneFormat);
}
return phoneNum;
In the Main()
method, we initialize a string variable, phNumber
, representing a sample phone number ("123456789"
). We then call the formatPhNumber()
method, passing the phone number and an empty string as parameters (allowing the default format to be applied).
string phNumber = "123456789";
Console.WriteLine(formatPhNumber(phNumber, ""));
Here is the entire source code for formatting a phone number in C#:
using System;
using System.Text.RegularExpressions;
class PhoneFormatter {
public static string formatPhNumber(string phoneNum, string phoneFormat) {
if (phoneFormat == "") {
phoneFormat = "(##) ###-####";
}
Regex regex = new Regex(@"[^\d]");
phoneNum = regex.Replace(phoneNum, "");
if (phoneNum.Length > 0) {
phoneNum = Convert.ToInt64(phoneNum).ToString(phoneFormat);
}
return phoneNum;
}
static void Main() {
string phNumber = "123456789";
Console.WriteLine(formatPhNumber(phNumber, ""));
}
}
The output of the provided test case would be:
(12) 345-6789
This demonstrates the formatted version of the phone number according to the default format (##) ###-####
. Feel free to customize the input phone number and format as needed for your specific use case.
Format a String as a Telephone Number in C# Using String.Format
An alternative method for formatting a string as a telephone number is using the String.Format
function. The String.Format
method allows us to format strings using a format specifier.
In the context of phone number formatting, we can define a format string that includes placeholders for different parts of the phone number.
using System;
class PhoneFormatter {
public static string FormatPhoneNumber(string areaCode, string firstPart, string secondPart) {
string format = "({0}) {1}-{2}";
return string.Format(format, areaCode, firstPart, secondPart);
}
static void Main() {
// Main method content
}
}
We define a method named FormatPhoneNumber
within the PhoneFormatter
class. This method takes three string parameters: areaCode
, firstPart
, and secondPart
, representing different segments of the phone number.
Inside the method, we create a format string where {0}
, {1}
, and {2}
are placeholders for the area code, first part, and second part, respectively.
public static string FormatPhoneNumber(string areaCode, string firstPart, string secondPart) {
string format = "({0}) {1}-{2}";
return string.Format(format, areaCode, firstPart, secondPart);
}
In the Main()
method, we initialize variables representing the area code, first part, and second part of a sample phone number. We then call the FormatPhoneNumber
method with these values and print the result to the console.
static void Main() {
string areaCode = "123";
string firstPart = "456";
string secondPart = "7890";
Console.WriteLine(FormatPhoneNumber(areaCode, firstPart, secondPart));
}
Here is the complete source code for formatting a phone number using String.Format
:
using System;
class PhoneFormatter {
public static string FormatPhoneNumber(string areaCode, string firstPart, string secondPart) {
string format = "({0}) {1}-{2}";
return string.Format(format, areaCode, firstPart, secondPart);
}
static void Main() {
string areaCode = "123";
string firstPart = "456";
string secondPart = "7890";
Console.WriteLine(FormatPhoneNumber(areaCode, firstPart, secondPart));
}
}
The output of the provided test case would be:
(123) 456-7890
This result showcases the formatted version of the phone number using the specified format string. Customize the area code, first part, and second part as needed for your specific scenarios.
Format a String as a Telephone Number in C# Using String Interpolation
String interpolation is a convenient and readable way to format strings in C#. This approach offers a concise syntax that is both expressive and easy to understand.
To utilize string interpolation, we don’t need additional libraries, as it’s a feature inherent in C#. Let’s see how we can apply string interpolation to format a phone number.
using System;
class PhoneFormatter {
public static string FormatPhoneNumber(string areaCode, string firstPart, string secondPart) {
return $"({areaCode}) {firstPart}-{secondPart}";
}
static void Main() {
// Main method content
}
}
In the PhoneFormatter
class, the FormatPhoneNumber
method is defined. This method takes three string parameters: areaCode
, firstPart
, and secondPart
.
Inside this method, string interpolation is applied using the $
symbol followed by curly braces {}
to embed the variables directly into the string.
public static string FormatPhoneNumber(string areaCode, string firstPart, string secondPart) {
return $"({areaCode}) {firstPart}-{secondPart}";
}
In the Main()
method, variables representing the area code, first part, and second part of a sample phone number are initialized. The FormatPhoneNumber
method is then called with these values, and the result is printed to the console.
static void Main() {
string areaCode = "123";
string firstPart = "456";
string secondPart = "7890";
Console.WriteLine(FormatPhoneNumber(areaCode, firstPart, secondPart));
}
Here is the complete source code for formatting a phone number using string interpolation in C#:
using System;
class PhoneFormatter {
public static string FormatPhoneNumber(string areaCode, string firstPart, string secondPart) {
return $"({areaCode}) {firstPart}-{secondPart}";
}
static void Main() {
string areaCode = "123";
string firstPart = "456";
string secondPart = "7890";
Console.WriteLine(FormatPhoneNumber(areaCode, firstPart, secondPart));
}
}
The output of the provided code would be:
(123) 456-7890
Conclusion
Formatting a string as a telephone number in C# can be accomplished through various methods, each with its strengths. Whether you prefer the simplicity of String.Format
, the conciseness of string interpolation, or the control of a custom method, C# provides the tools to meet your formatting needs.
Choose the method that aligns with your coding style and project requirements.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn