How to Escape Double Quotes in C#
- Escape Double Quotes in C# Using Verbatim String Literals
-
Escape Double Quotes in C# Using the Backslash (
\
) Escape Character - Escape Double Quotes in C# Using String Interpolation
- Escape Double Quotes in C# Using Character Escape Sequences
- Escape Double Quotes in C# Using a Single Quote Character Literal
- Conclusion
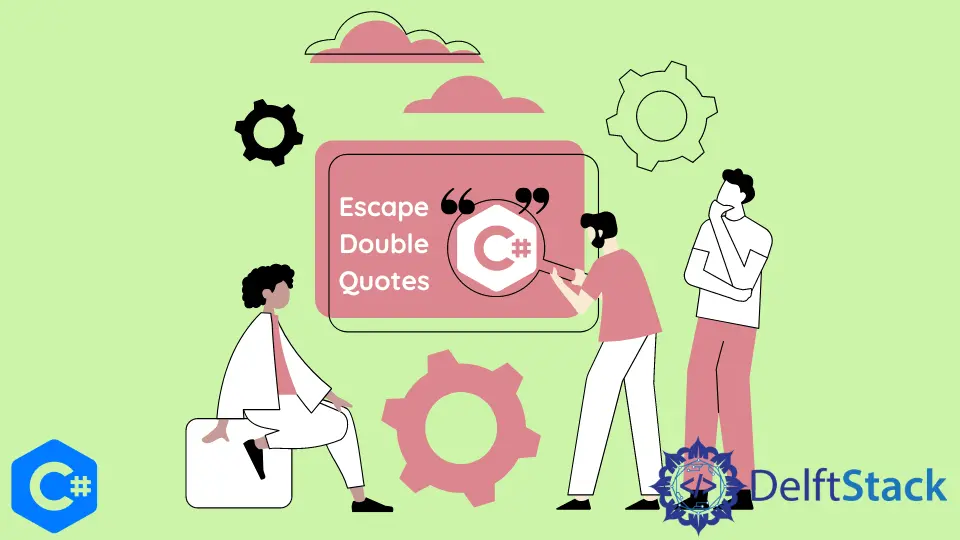
Double quotes are fundamental in C# programming, particularly when dealing with strings. However, incorporating double quotes within a string can pose challenges because double quotes are also used to define string literals.
In this article, we explore various techniques for escaping double quotes in C#, offering solutions that enhance code readability and simplify string handling. We’ll cover verbatim string literals, the backslash escape character, string interpolation, character escape sequences, and the innovative use of single-quote character literals.
Escape Double Quotes in C# Using Verbatim String Literals
In C#, verbatim string literals provide a convenient way to work with strings containing special characters like double quotes, backslashes, or line breaks. These literals are marked by the @
symbol before the string declaration.
Verbatim string literals are particularly handy when you need to escape double quotes. You just need to prefix the string with the @
symbol, which instructs the compiler to interpret the string exactly as it appears without the need for additional escaping.
Here’s an example:
string verbatimString = @"This is a ""quoted"" string.";
In the above example, the verbatim string @"This is a ""quoted"" string."
contains double quotes, and they don’t require any extra escaping.
This is different from regular string literals, where you would need to escape double quotes using a backslash:
string regularString = "This is a \"quoted\" string.";
Using verbatim string literals enhances code readability and helps prevent errors related to escaping double quotes. This feature is especially beneficial when working with strings that contain numerous special characters.
Here are some key points to remember:
- Opt for verbatim string literals when you want to maintain the exact formatting of a string, especially when it includes special characters.
- If you decide to use verbatim string literals in your code, maintain consistency to improve code readability. Either use them consistently throughout or avoid them altogether.
- Verbatim strings also preserve line breaks. While this can be useful in specific scenarios, be cautious as it might result in unintended line breaks if not managed properly.
- Keep in mind that if you use verbatim string literals within interpolated strings, you’ll still need to escape double quotes because interpolation expressions are evaluated within regular string contexts.
Escape Double Quotes in C# Using the Backslash (\
) Escape Character
Escape characters are special sequences that serve to represent characters that are challenging to input directly. In C#, the backslash (\
) is the designated escape character, indicating that the character following it should be treated differently.
When placed before a double quote, it instructs the compiler to interpret the double quote as a literal character, not as the opening or closing of a string.
Escaping double quotes in C# is as straightforward as adding a backslash (\
) before the double quote. Consider this example:
string myString = "This is a \"quoted\" string";
In this code, the double quotes within the string are prefixed with a backslash, explicitly telling the compiler to treat them as literal characters. Consequently, the string myString
will contain the text This is a "quoted" string
.
As demonstrated in the example above, escaping double quotes is essential when you want to incorporate double quotes within a string literal. This can be particularly handy when working with text that necessitates quoting, such as quoting a specific phrase or using quotations within a message.
The backslash (\
) escape character isn’t limited to double quotes; it can also be used to escape other special characters.
For instance, you can use it to escape backslashes themselves or to include newline characters (\n
) within a string.
string path = "C:\\MyDocuments\\File.txt";
string multiLineText = "This is a multi-line string.\nIt has two lines.";
Maintaining consistency in your use of the backslash escape character when dealing with double quotes is essential for code clarity. Once you start using it to escape double quotes, stick to that pattern throughout your code.
While escaping double quotes is vital, consider how it impacts the readability of your code, especially if your strings contain numerous double quotes. In cases where you have strings with multiple double quotes, you may want to contemplate using verbatim string literals (those prefixed with @
).
This eliminates the need for escaping double quotes and can enhance code readability.
Escape Double Quotes in C# Using String Interpolation
Escaping double quotes through the backslash (\
) escape character or using verbatim string literals are well-known methods in C#. However, there’s an alternative, more elegant approach that often eliminates the need for escaping double quotes: string interpolation.
String interpolation is a feature introduced in C# 6.0 that allows you to embed expressions within string literals. It simplifies the creation of formatted strings by replacing placeholders in the string with the values of expressions.
It’s not only efficient but also enhances the readability of your code.
String interpolation is denoted by the $
symbol preceding the string. Here’s a basic example to illustrate its simplicity:
string name = "John";
string greeting = $"Hello, {name}!";
In this example, the {name}
expression is replaced with the value of the name
variable, resulting in the string "Hello, John!"
.
String interpolation isn’t limited to variables; you can also incorporate double quotes directly within interpolated strings without the need for escaping. This feature is particularly beneficial when you want to create strings that contain quoted text or messages.
Here’s how you can include double quotes using string interpolation:
string quotedText = "This is a \"quoted\" string.";
string interpolatedString = $"This is an example of an {quotedText} using string interpolation.";
In the above code, the quotedText
variable contains a string with double quotes. When we use string interpolation to include it in another string, there’s no need to escape the double quotes.
The result is a well-structured string without any extra characters or escaping.
With string interpolation, you are liberated from the task of escaping double quotes or other special characters within the interpolated expressions. This not only simplifies your code but also reduces the likelihood of errors.
Another significant advantage is that making changes becomes more straightforward. When updates are needed, you only need to modify the expressions or variables within the string.
String interpolation proves to be a clean and efficient method to handle double quotes in C#, contributing to both code clarity and maintenance ease.
Escape Double Quotes in C# Using Character Escape Sequences
In C#, character escape sequences provide a straightforward method for representing special characters within strings. This is achieved by appending a backslash (\
) followed by a specific code to the character you want to include.
When it comes to double quotes, the character escape sequence to employ is \u0022
. This sequence enables you to seamlessly integrate double quotes within a string without triggering syntax errors.
Let’s dive into practical examples to illustrate the simplicity of using character escape sequences. To include a double quote character within a string, all you need to do is insert \u0022
at the desired location within the string.
string stringWithDoubleQuote = "This is a string containing a double quote: \u0022inside quotes\u0022.";
In this example, the string stringWithDoubleQuote
contains the text: This is a string containing a double quote: "inside quotes".
You can also employ character escape sequences when working with concatenated strings, as shown here:
string name = "Alice";
string message = "Hello, " + name + " said, \"Welcome to the party!\"";
In this case, the variable message
will hold the following text: `“Hello, Alice said, “Welcome to the party!”.”
Often, constructing JSON or XML strings necessitates the inclusion of double quotes around attribute values or object keys. Character escape sequences make this a breeze:
string json = "{\"name\": \"John\", \"age\": 30}";
The json
variable stores a JSON string: {"name": "John", "age": 30}
.
Utilizing character escape sequences to escape double quotes in C# strings offers several advantages:
- It enhances the readability of your code and conveys the explicit intention to include double quotes.
- Character escape sequences are a standardized approach to escaping special characters in C#, promoting consistency and facilitating code maintenance and collaboration.
- It ensures compatibility when working with data formats like JSON and XML, where double quotes hold significance.
- By adopting character escape sequences, you mitigate the risk of syntax errors and prevent unintended string termination, ensuring code reliability and robustness.
Escape Double Quotes in C# Using a Single Quote Character Literal
In C#, there’s a creative method to escape double quotes: by employing a single quote character literal. This technique entails defining a character literal that contains a double quote and subsequently converting it to a string.
In C#, character literals are enclosed within single quotes ('
). You can create a character literal that contains a double quote character and then easily concatenate it with other strings or transform it into a string.
Here’s how to execute this ingenious maneuver.
First, define a character literal with a double quote. To craft a character literal containing a double quote, enclose the double quote character with two single quotes:
char doubleQuoteChar = '"';
This line establishes a character literal named doubleQuoteChar
with a value of a double quote ("
).
Now that you possess this character literal, you can either combine it with other strings or convert it into a string by appending it to an empty string:
string stringWithDoubleQuote = "This is a " + doubleQuoteChar + "quoted" + doubleQuoteChar + " string.";
In this example, the variable stringWithDoubleQuote
holds the text: This is a "quoted" string.
Using a single quote character literal to escape double quotes in C# strings offers several benefits:
- This approach significantly enhances the readability of your code, making it abundantly clear that you intend to include double quotes within the string.
- It is a simple and intuitive method, free from complex escape sequences or additional characters.
- It seamlessly integrates with various string manipulation operations and concatenation techniques.
- You can deploy this approach across diverse scenarios, whether you’re constructing dynamic strings or handling user input.
This technique shines when you need to escape a limited number of double quotes within a string. However, if your string is replete with double quotes or you’re tackling intricate data formats like JSON or XML, alternative methods such as character escape sequences or verbatim string literals may be more suitable.
Conclusion
Escaping double quotes in C# is essential for smooth string manipulation and code clarity.
Whether you choose verbatim string literals for preserving formatting, the backslash escape character for simplicity, string interpolation for elegance, character escape sequences for precision or single quote character literals for creativity, you have an array of tools at your disposal.
Select the method that aligns best with your specific needs and enjoy more readable and error-free code. By mastering these techniques, you’ll handle double quotes in C# with confidence and finesse.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn