How to Encrypt and Decrypt a String in C#
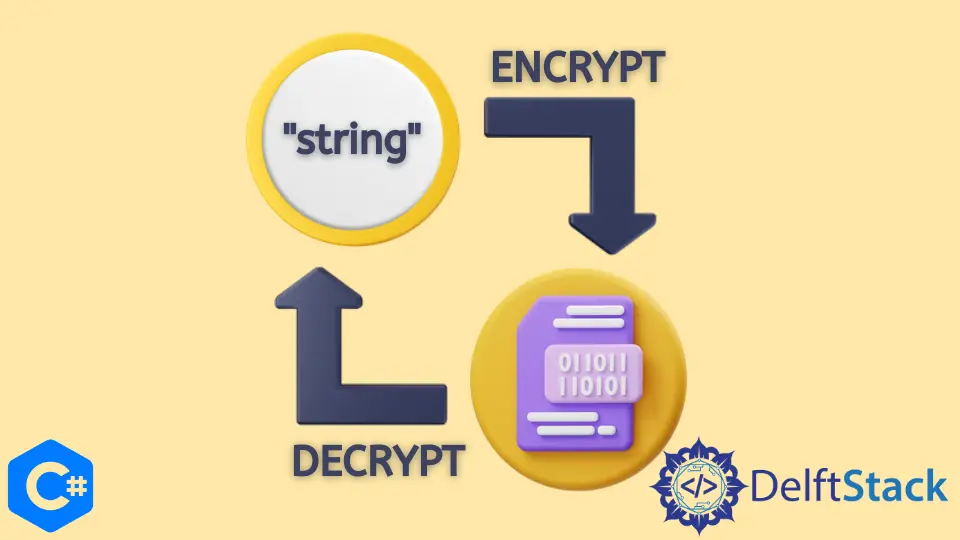
This tutorial will discuss methods to encrypt and decrypt a string in C#.
Encrypt a String With the AesManaged
Class in C#
Encryption is the process of converting data into ciphertext so that any unauthorized individuals cannot access the data. In this tutorial, we will use the Advanced Encryption Standard
(AES) algorithm to encrypt and decrypt a string in C#. The AesManaged
class provides methods to encrypt and decrypt our string using the AES algorithm. The CreateEncryptor()
function can encrypt data using a key. We have to pass our string keys to the CreateEncryptor()
function. The following code example shows us how to encrypt a string according to the AES algorithm with the CreateEncryptor()
function in C#.
using System;
using System.IO;
using System.Security.Cryptography;
using System.Text;
namespace encrypt_decrypt_string {
class Program {
static string Encrypt() {
try {
string textToEncrypt = "WaterWorld";
string ToReturn = "";
string publickey = "12345678";
string secretkey = "87654321";
byte[] secretkeyByte = {};
secretkeyByte = System.Text.Encoding.UTF8.GetBytes(secretkey);
byte[] publickeybyte = {};
publickeybyte = System.Text.Encoding.UTF8.GetBytes(publickey);
MemoryStream ms = null;
CryptoStream cs = null;
byte[] inputbyteArray = System.Text.Encoding.UTF8.GetBytes(textToEncrypt);
using (DESCryptoServiceProvider des = new DESCryptoServiceProvider()) {
ms = new MemoryStream();
cs = new CryptoStream(ms, des.CreateEncryptor(publickeybyte, secretkeyByte),
CryptoStreamMode.Write);
cs.Write(inputbyteArray, 0, inputbyteArray.Length);
cs.FlushFinalBlock();
ToReturn = Convert.ToBase64String(ms.ToArray());
}
return ToReturn;
} catch (Exception ex) {
throw new Exception(ex.Message, ex.InnerException);
}
}
static void Main(string[] args) {
string encrypted = Encrypt();
Console.WriteLine(encrypted);
}
}
}
Output:
6+PXxVWlBqcUnIdqsMyUHA==
In the above code, we encrypted the string WaterWorld
with the publickey
and secretkey
as keys and returned the 6+PXxVWlBqcUnIdqsMyUHA==
as an encrypted string. Both keys must be at least 8 characters in length.
Decrypt a String With the AesManaged
Class in C#
Decryption is the process of converting ciphertext back to the original data so that authorized individuals can access the data. The CreateDecryptor()
function can decrypt data using a key. We have to pass our string keys to the CreateEncryptor()
function. The keys must be the same as the ones used in the CreateDecryptor()
function. The following code example shows us how to encrypt a string according to the AES algorithm with the CreateDecryptor()
function in C#.
using System;
using System.IO;
using System.Security.Cryptography;
using System.Text;
namespace encrypt_decrypt_string {
class Program {
static string Decrypt() {
try {
string textToDecrypt = "6+PXxVWlBqcUnIdqsMyUHA==";
string ToReturn = "";
string publickey = "12345678";
string secretkey = "87654321";
byte[] privatekeyByte = {};
privatekeyByte = System.Text.Encoding.UTF8.GetBytes(secretkey);
byte[] publickeybyte = {};
publickeybyte = System.Text.Encoding.UTF8.GetBytes(publickey);
MemoryStream ms = null;
CryptoStream cs = null;
byte[] inputbyteArray = new byte[textToDecrypt.Replace(" ", "+").Length];
inputbyteArray = Convert.FromBase64String(textToDecrypt.Replace(" ", "+"));
using (DESCryptoServiceProvider des = new DESCryptoServiceProvider()) {
ms = new MemoryStream();
cs = new CryptoStream(ms, des.CreateDecryptor(publickeybyte, privatekeyByte),
CryptoStreamMode.Write);
cs.Write(inputbyteArray, 0, inputbyteArray.Length);
cs.FlushFinalBlock();
Encoding encoding = Encoding.UTF8;
ToReturn = encoding.GetString(ms.ToArray());
}
return ToReturn;
} catch (Exception ae) {
throw new Exception(ae.Message, ae.InnerException);
}
}
static void Main(string[] args) {
string decrypted = Decrypt();
Console.WriteLine(decrypted);
}
}
}
Output:
WaterWorld
In the above code, we converted the encrypted string 6+PXxVWlBqcUnIdqsMyUHA==
from the previous example back to its original form WaterWorld
with the publickey
and secretkey
as keys. The keys need to be the same as the keys used in the previous example for this method to work.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn