How to Encode and Decode a Base64 String in C#
-
Encode a String to a Base64 String With the
Convert.ToBase64String()
Method inC#
-
Decode a String From a Base64 String With the
Convert.FromBase64String()
Method inC#
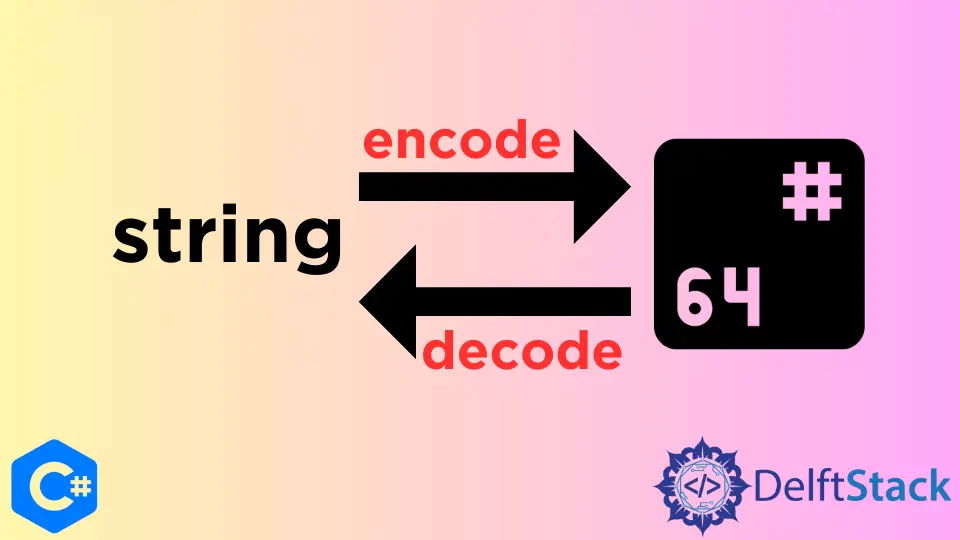
This tutorial will discuss methods to encode and decode a base64 string in C#.
Encode a String to a Base64 String With the Convert.ToBase64String()
Method in C#
The Convert
class can convert one base data type to another base data type in C#. The Convert.ToBase64String()
method converts an array of bytes to a base64 string variable in C#. To convert a string variable to a base64 string, we must first convert that string to an array of bytes. The Encoding.UTF8.GetBytes()
method is converts a string variable to an array of bytes in C#. The following code example shows us how we can encode a string variable to a base64 string with the Convert.ToBase64String()
function in C#.
using System;
using System.Text;
namespace encode_and_decode_base64_string {
class Program {
public static string Base64Encode(string plainText) {
var plainTextBytes = Encoding.UTF8.GetBytes(plainText);
return System.Convert.ToBase64String(plainTextBytes);
}
static void Main(string[] args) {
string original = "This is a string";
Console.WriteLine("Original String = " + original);
string base64 = Base64Encode(original);
Console.WriteLine("Encoded String = " + base64);
}
}
}
Output:
Original String = This is a string
Encoded String = VGhpcyBpcyBhIHN0cmluZw==
We encoded the string variable original
with the value This is a string
to the base64 string base64
with the value VGhpcyBpcyBhIHN0cmluZw==
. We first converted the string plainText
to the bytes array plainTextBytes
with the Encoding.UTF8.GetBytes(plainText)
function. We encoded the bytes array plainTextBytes
to the base64
string with the Convert.ToBase64String(plainTextBytes)
function.
Decode a String From a Base64 String With the Convert.FromBase64String()
Method in C#
The Convert.FromBase64String()
method can convert a base64 string variable to an array of bytes in C#. To convert a base64 encoded string to a standard string variable, we have to convert the array of bytes returned by the Convert.FromBase64String()
method to a string variable using the Encoding.UTF8.GetString()
method in C#. The following code example shows us how to decode a base64 string to a string variable with the Convert.FromBase64String()
function in C#.
using System;
using System.Text;
namespace encode_and_decode_base64_string {
class Program {
public static string Base64Decode(string base64EncodedData) {
var base64EncodedBytes = System.Convert.FromBase64String(base64EncodedData);
return System.Text.Encoding.UTF8.GetString(base64EncodedBytes);
}
static void Main(string[] args) {
string base64 = "VGhpcyBpcyBhIHN0cmluZw==";
Console.WriteLine("Encoded String = " + base64);
string original = Base64Decode(base64);
Console.WriteLine("Decoded String = " + original);
}
}
}
Output:
Encoded String = VGhpcyBpcyBhIHN0cmluZw==
Decoded String = This is a string
We decoded the base64 string base64
with the value VGhpcyBpcyBhIHN0cmluZw==
to the string variable original
with the value This is a string
. We first converted the base64EncodedData
string to the bytes array base64EncodedBytes
with the Convert.FromBase64String(base64EncodedData)
function. We converted the bytes array base64EncodedBytes
to the string variable original
with the Encoding.UTF8.GetString(base64EncodedBytes)
function.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn