How to Delete All Files in a Directory in C#
-
Delete All the Files Inside a Directory With the
DirectoryInfo.GetFiles()
Method in C# -
Delete All the Files Inside a Directory With the
DirectoryInfo.GetDirectories()
Method in C# -
Delete All the Files Inside a Directory With the
DirectoryInfo.EnumerateFiles()
Method in C# -
Delete All the Directories Inside a Directory With the
DirectoryInfo.EnumerateDirectories()
Method in C# - Conclusion
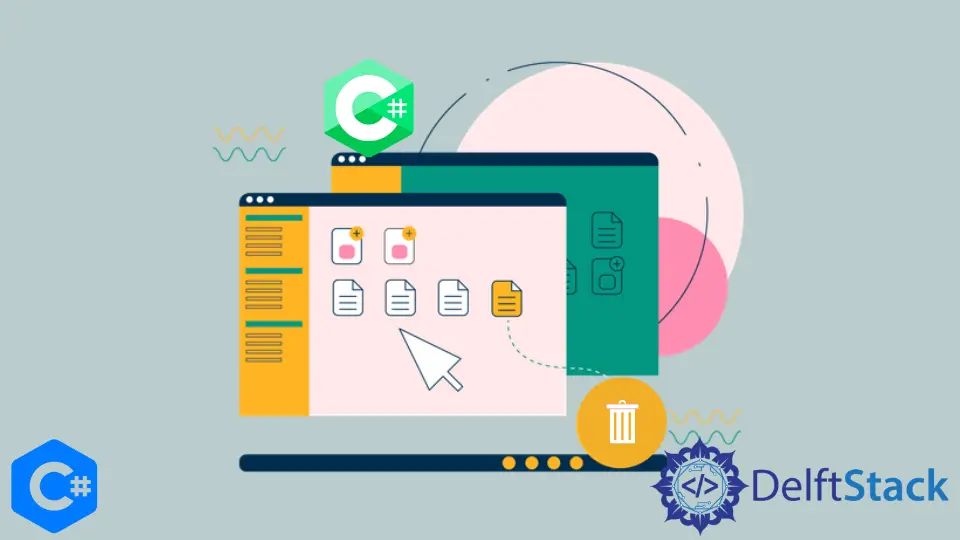
Efficient file management is pivotal in programming, often necessitating the deletion of all files in a directory. This may be essential for data cleanup, preparing for new data, or maintaining an organized file structure.
In C#, accomplishing this task is streamlined through the use of different methods, providing a clean and effective solution. This tutorial will discuss the methods to delete all the files inside a directory in C#.
Delete All the Files Inside a Directory With the DirectoryInfo.GetFiles()
Method in C#
The DirectoryInfo.GetFiles()
method in C# gets all the files inside a specified directory. The DirectoryInfo.GetFiles()
method takes the path as an argument and returns an array of the FileInfo
class objects that contain information about each file in that directory.
We can get all the files inside the specified directory with the DirectoryInfo.GetFiles()
method and then delete the files with the FileInfo.Delete()
method.
The following code example shows us how we can delete all the files inside a directory with the DirectoryInfo.GetFiles()
and FileInfo.Delete()
methods in C#.
Code Input:
using System.IO;
using System;
class Program {
public static void Main(string[] args) {
// Specify the directory path
string directoryPath = @"Directory";
// Create a DirectoryInfo object for the specified directory
DirectoryInfo directory = new DirectoryInfo(directoryPath);
// Delete all files in the directory
foreach (FileInfo file in directory.GetFiles()) {
file.Delete();
}
// Output message indicating successful deletion
Console.WriteLine("Files deleted successfully");
}
}
Firstly, we define a variable directoryPath
to hold the path of the directory we wish to operate on. This establishes the target location for deleting all files.
Subsequently, we create a DirectoryInfo
object named directory
. This object encapsulates information about the specified directory, laying the foundation for our subsequent operations.
The crux of the operation lies within a foreach
loop. Iterating through each file in the directory obtained by invoking GetFiles
on our directory
object, we utilize the Delete
method to remove each file individually.
After successfully deleting all files in the directory, a simple Console.WriteLine
statement communicates this accomplishment. The output message, Files deleted successfully
, serves as a reassuring confirmation of the completed task.
Code Output:
Files deleted successfully
Executing this script ensures the removal of all files within the specified directory, providing a clean slate for subsequent operations. The output message serves as a clear indication of the successful completion of the file deletion process.
Delete All the Files Inside a Directory With the DirectoryInfo.GetDirectories()
Method in C#
If we also want to delete all the sub-directories inside the C:\Sample\
directory, we can use the DirectoryInfo.GetDirectories()
method to get all the sub-directories inside the C:\Sample\
directory. The DirectoryInfo.GetDirectories()
method returns an array of the DirectoryInfo
class objects that contain information about all the sub-directories.
We can delete the sub-directories with the DirectoryInfo.Delete()
method. We can also delete all the sub-directories inside each sub-directory by specifying true
inside the arguments of the DirectoryInfo.Delete()
method.
The following code example shows us how we can delete all the files and sub-directories inside a directory with the DirectoryInfo.GetDirectories()
method in C#.
Code Input:
using System;
using System.IO;
class Program {
public static void Main(string[] args) {
// Specify the directory path
string directoryPath = @"Directory";
// Create a DirectoryInfo object for the specified directory
DirectoryInfo directory = new DirectoryInfo(directoryPath);
// Delete all files in the directory
foreach (FileInfo file in directory.GetFiles()) {
file.Delete();
}
// Delete all subdirectories and their contents
foreach (DirectoryInfo subdirectory in directory.GetDirectories()) {
subdirectory.Delete(true);
}
// Output message indicating successful deletion
Console.WriteLine("Files and sub-directories deleted successfully");
}
}
The journey begins with the definition of directoryPath
, serving as the target path where we intend to delete all files and directories.
Next, we instantiate a DirectoryInfo
object named directory
based on the specified path. This object becomes the focal point for interacting with the contents of the directory.
The initial loop focuses on deleting all files within the directory. It traverses the files using directory.GetFiles()
, and for each file encountered, the Delete
method is invoked.
Building on the momentum, the subsequent loop addresses subdirectories. Utilizing directory.GetDirectories()
, it iterates through each subdirectory, invoking Delete(true)
to ensure recursive deletion of the directory and its contents.
Upon successful completion of the deletion process, a reassuring message is outputted using Console.WriteLine
, stating Files and sub-directories deleted successfully
.
Code Output:
Files and Sub-directories deleted successfully
Executing this script guarantees the removal of all files and directories within the specified directory, leaving a clean slate for subsequent operations. The output message serves as a clear affirmation of the successful completion of the file and directory deletion process.
Delete All the Files Inside a Directory With the DirectoryInfo.EnumerateFiles()
Method in C#
The methods discussed in the previous section do the job well, but there is a more robust way to do the same thing. The DirectoryInfo.EnumerateFiles()
method iterates through each file inside the directory.
This approach is much faster than the previous approach. The DirectoryInfo.EnumerateFiles()
method can be used inside the foreach
loop to get an object of the FileInfo
class in each iteration.
We can delete each file with the FileInfo.Delete()
method.
The following code example shows us how we can delete all the files inside a directory with the DirectoryInfo.EnumerateFiles()
method in C#.
Code Input:
using System;
using System.IO;
class Program {
public static void Main(string[] args) {
// Specify the directory path
string directoryPath = @"Directory";
// Create a DirectoryInfo object for the specified directory
DirectoryInfo directory = new DirectoryInfo(directoryPath);
// Delete all files in the directory
foreach (FileInfo file in directory.EnumerateFiles()) {
file.Delete();
}
// Output message indicating successful deletion
Console.WriteLine("Files deleted successfully");
}
}
Our journey begins by setting the variable directoryPath
to the path of the directory where we want to perform the file deletion.
Next, a DirectoryInfo
object named directory
is instantiated, representing the specified directory. This object becomes our interface to interact with the files within the directory.
The pivotal moment occurs within the foreach
loop. Utilizing directory.EnumerateFiles()
, it retrieves an enumerable collection of FileInfo
objects, each representing a file in the directory.
For each file encountered, the Delete
method is invoked, seamlessly removing the file from the directory.
Upon the successful completion of the file deletion process, a reassuring message is printed to the console using Console.WriteLine
, stating Files deleted successfully
.
Code Output:
Files deleted successfully
Executing this script ensures the removal of all files within the specified directory, concluding with a confirmation message affirming the successful completion of the file deletion process.
Delete All the Directories Inside a Directory With the DirectoryInfo.EnumerateDirectories()
Method in C#
If we also want to delete all the subdirectories inside our main directory, we can also use the DirectoryInfo.EnumerateDirectories()
method in C#. The DirectoryInfo.EnumerateDirectories()
method is used to iterate through each sub-directory inside the main directory.
The following code example shows us how we can delete all the files and sub-directories inside a directory with the DirectoryInfo.EnumerateFiles()
method in C#.
Code Input:
using System;
using System.IO;
class Program {
public static void Main(string[] args) {
// Specify the directory path
string directoryPath = @"Directory";
// Create a DirectoryInfo object for the specified directory
DirectoryInfo directory = new DirectoryInfo(directoryPath);
// Delete all files in the directory
foreach (FileInfo file in directory.EnumerateFiles()) {
file.Delete();
}
// Delete all subdirectories and their contents
foreach (DirectoryInfo subdirectory in directory.EnumerateDirectories()) {
subdirectory.Delete(true);
}
// Output message indicating successful deletion
Console.WriteLine("Files and sub-directories deleted successfully");
}
}
We start by defining the variable directoryPath
, representing the target directory where we want to delete all files and directories.
Next, we instantiate a DirectoryInfo
object named directory
based on the specified path. This object becomes our gateway to interacting with the files and directories within the target directory.
The initial loop focuses on deleting all files within the directory. Leveraging directory.EnumerateFiles()
, it retrieves an enumerable collection of file paths.
For each file encountered, the Delete
method is invoked, removing the file.
Building on the momentum, the subsequent loop addresses subdirectories. Utilizing directory.EnumerateDirectories()
, it iterates through each subdirectory.
The Delete(true)
method ensures the recursive deletion of the directory and its contents.
Upon successful completion of the deletion process, a confirmation message is displayed using Console.WriteLine
, stating Files and sub-directories deleted successfully
.
Code Output:
Files and sub-directories deleted successfully
Executing this script guarantees the removal of all files and directories within the specified directory, leaving a clean slate for subsequent operations. The output message serves as a clear affirmation of the successful completion of the file and directory deletion process.
Conclusion
In conclusion, the presented methods for deleting files in a directory using C# demonstrate the language’s versatility and efficiency in file management. Whether employing DirectoryInfo.GetFiles
and DirectoryInfo.GetDirectories
or DirectoryInfo.EnumerateDirectories()
, these techniques offer accessible solutions for developers at various skill levels.
The simplicity of the code snippets underscores the elegance of C#’s file manipulation capabilities, allowing for effortless deletion of files and directories. These methods are invaluable in scenarios requiring systematic cleanup, contributing to the overall robustness, efficiency, and maintainability of C# applications.
Developers can confidently apply these approaches to ensure a thorough and effective file management process in their projects.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Csharp File
- How to Get File Name From the Path in C#
- How to Rename a File in C#
- How to Download a File From a URL in C#
- How to Read a Text File Line by Line in C#
- How to Read PDF File in C#
- How to Read Embedded Resource Text File in C#