How to Get the Current Folder Path in C#
-
C# Program to Get Current Folder Path Using
GetCurrentDirectory()
Method -
C# Program to Get Current Folder Path Using
GetDirectoryName()
Method -
C# Program to Get Current Folder Path Using
CurrentDirectory
Property
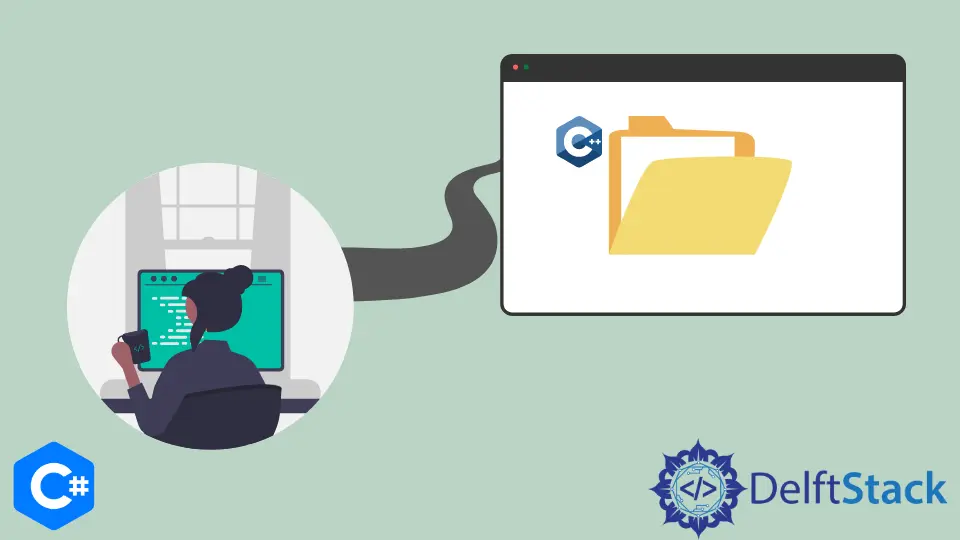
In C# we can use Directory
class to work with directories. A directory or a folder is used to store files.
In this article, we are going to discuss various methods that are used to get the current folder path.
C# Program to Get Current Folder Path Using GetCurrentDirectory()
Method
The method GetCurrentDirectory()
is used to fetch the current folder path in which your working application is stored. In this case, it will fetch the directory from where our program runs.
The correct syntax to use this method is as follows:
Directory.GetCurrentDirectory();
Example Code:
using System;
using System.IO;
namespace CurrentFolder {
class Folder {
static void Main(string[] args) {
var CurrentDirectory = Directory.GetCurrentDirectory();
Console.WriteLine(CurrentDirectory);
}
}
}
Output:
C:\Users\Cv\source\repos\ClassLibrary1\ClassLibrary1\bin\Debug\netstandard2.0
//Directory where the program is saved i.e current folder path
C# Program to Get Current Folder Path Using GetDirectoryName()
Method
The method GetDirectoryName()
is used to get the current directory. It accepts a string
as a parameter that tells about the path of a file.
But if we don’t know the path of the file then we pass Assembly.GetEntryAssembly().Location
as a parameter to this method. Assembly.GetEntryAssembly().Location
fetches the file path with the file name. Using it, GetDirectoryName()
fetches the current directory.
The correct syntax to use this method is as follows:
GetDirectoryName(PathString);
System.IO.Path.GetDirectoryName(Assembly.GetEntryAssembly().Location);
Example Code:
using System;
using System.Reflection;
namespace CurrentFolder {
class Folder {
static void Main(string[] args) {
var CurrentDirectory = System.IO.Path.GetDirectoryName(Assembly.GetEntryAssembly().Location);
Console.WriteLine(CurrentDirectory);
}
}
}
Output:
C:\Users\Cv\source\repos\ClassLibrary1\ClassLibrary1\bin\Debug\netstandard2.0
//Directory where the program is saved i.e current folder path
C# Program to Get Current Folder Path Using CurrentDirectory
Property
The property CurrentDirectory
is used to get the full path of the current working directory. The property CurrentDirectory
is defined in System.Environment
class, that’s why it is used as Environment.CurrentDirectory
.
The correct syntax to use this property is as follows:
var CurrentDirectory = Environment.CurrentDirectory;
Example Code:
using System;
namespace CurrentFolder {
class Folder {
static void Main() {
var CurrentDirectory = Environment.CurrentDirectory;
Console.WriteLine(CurrentDirectory);
}
}
}
Output:
C:\Users\Cv\source\repos\ClassLibrary1\ClassLibrary1\bin\Debug\netstandard2.0
//Directory where the program is saved i.e current folder path