How to Remove String From String in C#
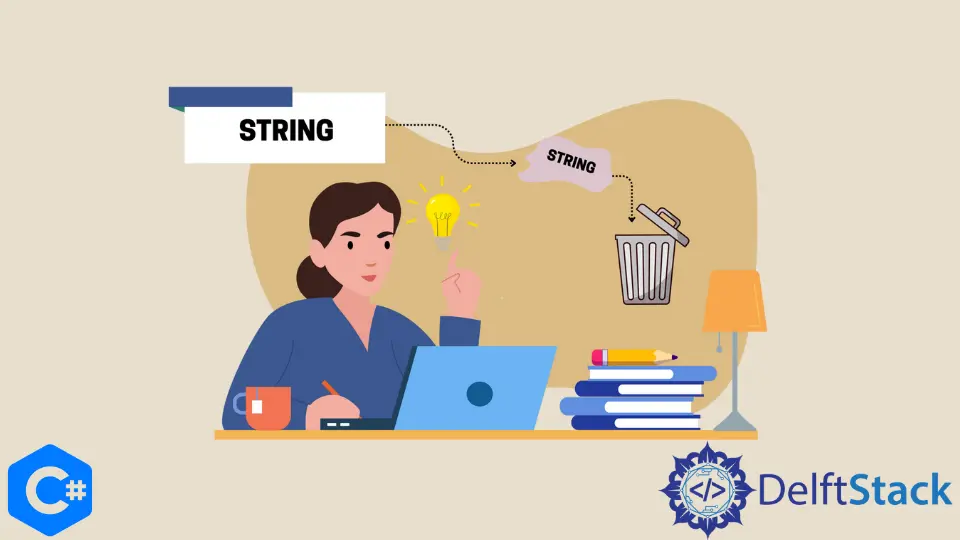
This article illustrates how to remove characters from a string using the C# programming language beginning from a specific character forward or at a particular location within the string.
Use the String.Remove()
Method to Remove String Characters in a String
It is used to delete all the characters in a string starting at a certain point already set. For example, if we do not supply the length, all characters after the specified position will be removed.
Changing the total amount of parameters sent to this method is one way to override its behavior.
The following example demonstrates how we will use four separate removal functions, each of which to remove individual characters or strings.
First things first, let us look at the library’s imports.
using System;
Create a class named StringRemoveBySaad
that contains the Main()
function.
class StringRemoveBySaad {
static void Main(string[] args) {}
}
Now we will create a variable in which we’ll save a string.
string sampleString = "Saad Aslam is a programming article writer at DelftStack";
We will use the .Remove()
method, giving one parameter to remove any characters that come after the first 20 characters, and then we will show the result on the console.
string remove20 = sampleString.Remove(20);
Console.WriteLine(remove20);
Now, by providing the function with two parameters, we will remove characters beginning at the 10th place and continuing through the following 12 characters.
String removeSelected = sampleString.Remove(10, 12);
Console.WriteLine(removeSelected);
Let us imagine you want to remove everything in a string that comes after or before a specific substring. We can locate the location of the substring by using the String.IndexOf()
function, and we can also use the beginning index and the number of characters to delete.
After that, we will construct a position variable named posString
and then use the IndexOf()
method to pass a string index article
as a parameter.
int posString = sampleString.IndexOf("article");
The following two code examples will delete everything in a string before and after the substring article
.
We shall use the .Remove()
method to remove the characters after the specified index by passing the posString
variable to the function.
string afterString = sampleString.Remove(posString);
Console.WriteLine(afterString);
After that, we will use the .Remove()
function to eliminate the characters before the article
substring while keeping the founder substring.
string beforeString = sampleString.Remove(0, posString);
Console.Write(beforeString);
Source Code
using System;
namespace RemoveStringCSharp {
class StringRemoveBySaad {
static void Main(string[] args) {
string sampleString = "Saad Aslam is a programming article writer at DelftStack";
string remove20 = sampleString.Remove(20);
Console.WriteLine(remove20);
String removeSelected = sampleString.Remove(10, 12);
Console.WriteLine(removeSelected);
int posString = sampleString.IndexOf("article");
if (posString > 0) {
string afterString = sampleString.Remove(posString);
Console.WriteLine(afterString);
string beforeString = sampleString.Remove(0, posString);
Console.Write(beforeString);
}
}
}
}
Output:
Saad Aslam is a prog
Saad Aslammming article writer at DelftStack
Saad Aslam is a programming
article writer at DelftStack
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn