How to Remove Quotes From String in C#
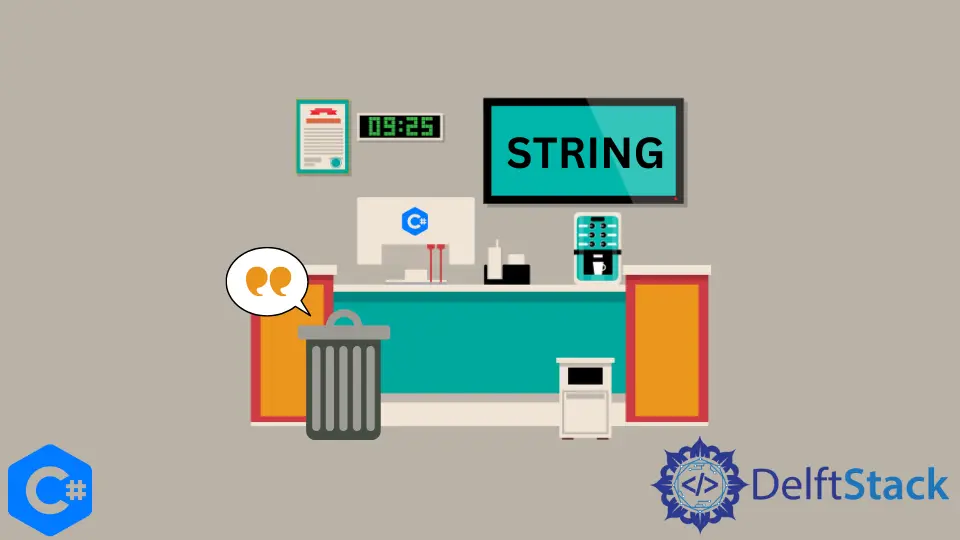
When working with strings in C#, you may often encounter situations where you need to remove quotes from a string variable. This is particularly common when dealing with user input, data parsing, or formatting strings for display. Fortunately, C# provides a straightforward method for this task using the String.Replace()
function. This method allows you to specify the character or substring you want to replace—in this case, the quotes—and replace them with an empty string.
In this article, we will explore various methods to effectively remove quotes from strings in C#, providing clear examples and explanations along the way.
Using String.Replace() Method
The String.Replace()
method is one of the simplest and most efficient ways to remove quotes from a string in C#. This method takes two parameters: the substring you want to replace and the string you want to replace it with. In our case, we will replace the double quotes with an empty string.
Here’s how you can do it:
string originalString = "\"Hello, World!\"";
string modifiedString = originalString.Replace("\"", "");
Console.WriteLine(modifiedString);
Output:
Hello, World!
In this example, we start with a string that contains quotes around “Hello, World!”. By using Replace("\"", "")
, we specify that we want to replace all occurrences of the double quote character with nothing. As a result, the output is a clean string without any quotes. This method is particularly useful because it operates on the entire string and replaces all instances of the specified character, making it efficient for larger strings.
Removing Single Quotes
In addition to removing double quotes, you may also need to remove single quotes from strings. The String.Replace()
method works just as effectively for single quotes. Here’s an example:
string originalString = "'Hello, World!'";
string modifiedString = originalString.Replace("'", "");
Console.WriteLine(modifiedString);
Output:
Hello, World!
As you can see, the process is identical to removing double quotes. By replacing the single quote character with an empty string, we achieve the desired result. This versatility makes String.Replace()
a powerful tool in your C# string manipulation toolkit. Whether you’re dealing with user-generated content or parsing data from external sources, this method can help you ensure that your strings are formatted correctly.
Using Regular Expressions
For more complex scenarios where you might want to remove both single and double quotes simultaneously, you can use the Regex
class from the System.Text.RegularExpressions
namespace. This method allows for more flexibility and can handle multiple characters or patterns in one go.
Here’s an example of how to use regular expressions to remove both types of quotes:
using System.Text.RegularExpressions;
string originalString = "\"Hello, 'World!'\"";
string modifiedString = Regex.Replace(originalString, "[\"']", "");
Console.WriteLine(modifiedString);
Output:
Hello, World!
In this code snippet, we import the System.Text.RegularExpressions
namespace to use the Regex.Replace()
method. The pattern ["']
denotes that we want to match both double and single quotes. By replacing them with an empty string, we effectively remove all quotes from the original string. Regular expressions offer greater control and can be particularly useful when dealing with more complex string manipulations, making them an excellent option for developers who need to handle various formatting scenarios.
Conclusion
Removing quotes from strings in C# is a straightforward process, thanks to the versatility of the String.Replace()
method and the power of regular expressions. Whether you’re dealing with double quotes, single quotes, or both, these methods provide you with the tools you need to ensure your strings are clean and properly formatted. By using these techniques, you can streamline your string manipulation tasks and improve your overall coding efficiency.
As you continue to work with strings in C#, remember that these methods can be easily adapted to suit your specific needs, making them invaluable for any developer.
FAQ
-
What is the best method to remove quotes from a string in C#?
The best method is to use the String.Replace() function, as it is simple and effective for removing quotes. -
Can I remove both single and double quotes at the same time?
Yes, you can use the Regex class to remove both types of quotes in one operation. -
What if I only want to remove quotes from the beginning and end of a string?
You can use theTrim()
method, which removes specified characters from the start and end of a string. -
Are there any performance considerations when using regular expressions?
Regular expressions can be slower than simple string replacements, especially on large strings or complex patterns. Use them judiciously.
- Can I remove quotes from a string in a case-insensitive manner?
The String.Replace() method is case-sensitive, but you can use Regex with the RegexOptions.IgnoreCase flag for case-insensitive replacements.
using the String.Replace() method and regular expressions. This guide provides clear examples and explanations to help you streamline your string manipulation tasks. Perfect for developers looking to enhance their coding efficiency.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn