How to Remove First Character From String in C#
-
Use the
String.Remove()
Method to Remove the First Character From String in C# -
Use the
String.Substring()
Method to Remove the First Character From String in C# - Use String Interpolation to Remove the First Character From String in C#
- Use LINQ to Remove the First Character From String in C#
- Conclusion
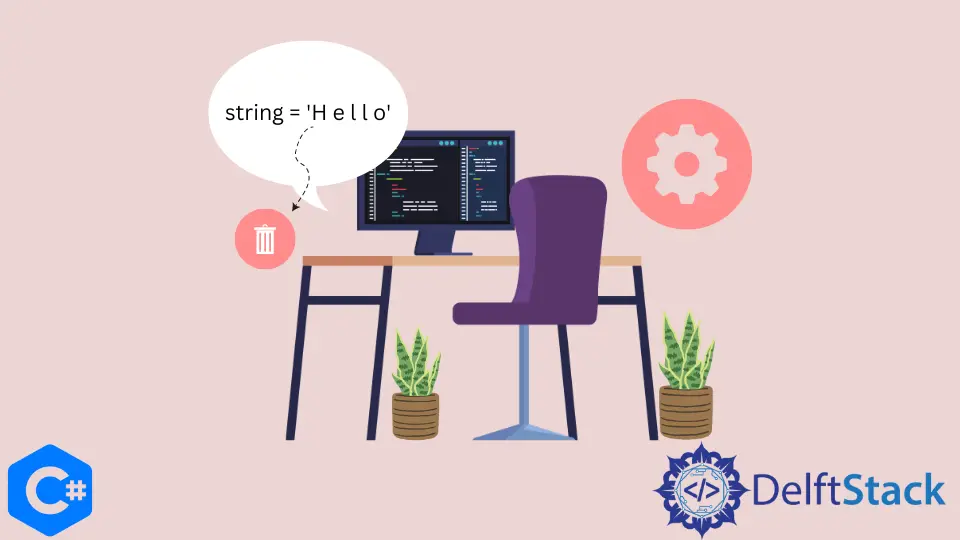
In C#, string manipulation is a common task, and sometimes, we may need to remove the first character from a string. Two commonly used methods for this purpose are String.Remove()
and String.Substring()
.
Additionally, we can achieve the same result using String Interpolation and LINQ. In this article, we’ll explore these methods.
Use the String.Remove()
Method to Remove the First Character From String in C#
The String.Remove(x, y)
method in C# removes a string value of a specified length and start index from the original string. It returns a new string in which the characters from index x
and having length y
are removed from the original string.
We can pass 0
as the starting index and 1
as the length to the String.Remove()
method to remove the string’s first character.
using System;
namespace remove_first_character {
class Program {
static void Main(string[] args) {
string s1 = "this is something";
s1 = s1.Remove(0, 1);
Console.WriteLine(s1);
}
}
}
Output:
his is something
In the code above, we declare and initialize a string variable s1
with the value "this is something"
. Then, we use the String.Remove()
method to remove the first character:
-
s1.Remove(0, 1)
removes characters starting from index0
(the first character) up to the specified length of1
. This effectively removes the first character ('t'
) from the string. -
The result is assigned back to the variable
s1
, overwriting the original string.
Lastly, the program displays the modified string s1
("his is something"
).
Use the String.Substring()
Method to Remove the First Character From String in C#
We can also use the String.Substring()
method to remove the string’s first character. The String.Substring(x)
method gets a smaller string from the original string that starts from the index x
in C#.
We can pass 1
as the starting index to remove the first character from a string.
using System;
namespace remove_first_character {
class Program {
static void Main(string[] args) {
string s1 = "this is something";
s1 = s1.Substring(1);
Console.WriteLine(s1);
}
}
}
Output:
his is something
In the code above, we declare and initialize a string variable s1
with the value "this is something"
. Then, we use the String.Substring()
method to remove the first character:
-
s1.Substring(1)
extracts a substring starting from the second character (index1
) to the end of the original string. -
The result is assigned back to the variable
s1
, removing the first character from the string.
Lastly, the program displays the modified string s1
("his is something"
).
Use String Interpolation to Remove the First Character From String in C#
String interpolation in C# involves creating a new string by combining string fragments, including variables and expressions, within a string literal. To remove the first character from a string using this method, you’ll construct a new string that excludes the initial character of the original string.
using System;
namespace remove_first_character {
class Program {
static void Main(string[] args) {
string s1 = "this is something";
s1 = $"{s1[0..1]}{s1[1..]}";
Console.WriteLine(s1);
}
}
}
Output:
his is something
In the code above, we initialize a string variable s1
with the value "this is something"
. Then, we use string interpolation to manipulate the string s1
as follows:
-
${s1[0..1]}
extracts the first character (index0
) and creates a substring containing only that character. -
${s1[1..]}
extracts a substring starting from the second character (index1
) to the end of the original string. These substrings are concatenated together to form a new string, effectively removing the first character. -
The modified string is stored back in the
s1
variable.
Lastly, the program displays the modified string s1
("his is something"
).
Use LINQ to Remove the First Character From String in C#
In C#, you can use Language-Integrated Query (LINQ) to remove the first character from a string. LINQ provides a convenient way to perform operations on sequences, including strings.
using System;
using System.Linq;
namespace remove_first_character {
class Program {
static void Main(string[] args) {
string s1 = "this is something";
s1 = new string(s1.Skip(1).ToArray());
Console.WriteLine(s1);
}
}
}
Output:
his is something
In the code above, we declare and initialize a string variable s1
with the value "this is something"
. We use LINQ to remove the first character from s1
:
-
s1.Skip(1)
skips the first character and returns the remaining characters as an enumerable. -
.ToArray()
converts the enumerable into an array of characters. -
new string(...)
constructs a new string from the character array, effectively removing the first character from the original string.
Lastly, the program displays the modified string s1
("his is something"
).
Conclusion
In this guide, we’ve covered various methods to remove the first character from a string in C#. We discussed using the String.Remove()
and String.Substring()
methods, as well as the String Interpolation and LINQ approaches.
These techniques provide you with flexibility and options for efficiently manipulating strings in C#. Whether you’re processing user input or modifying data, these methods will prove valuable in your string manipulation tasks in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn