How to Check Palindrome String in C#
-
Check Palindrome String With the
String.Substring()
Method inC#
-
Check Palindrome String With the LINQ Method in
C#
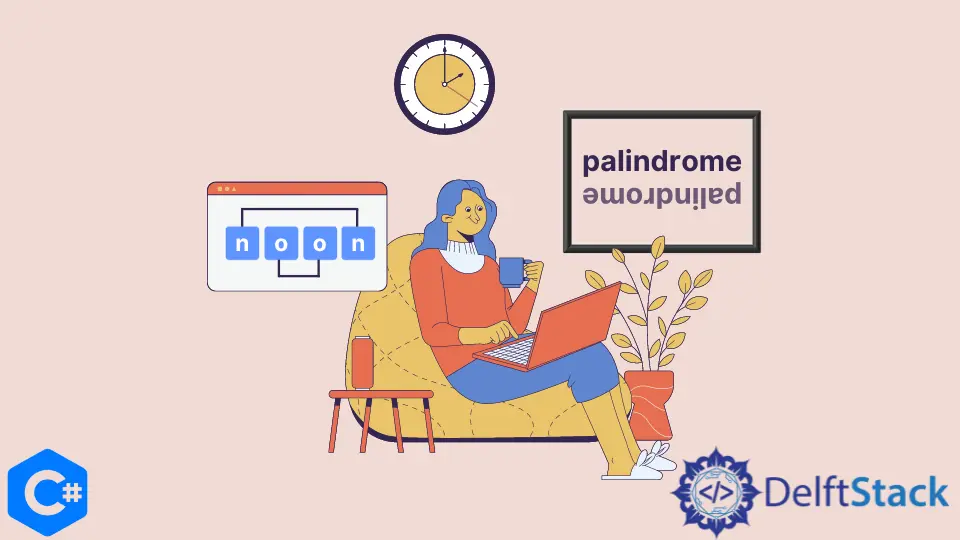
This tutorial will introduce the methods to check if a string is palindrome or not in C#.
Check Palindrome String With the String.Substring()
Method in C#
A string is considered palindrome if it is read the same forward and backward. Unfortunately, there is no built-in method to check whether a string is a palindrome or not in C#. But we can use the String.Substring()
method to split the string from the middle to get the first half. We can get the reverse of the second half by converting the string to an array of characters, reversing the sequence of characters with the Array.Reverse()
method, converting the reverse array to a string, and then split the new string from the middle with the String.Substring()
method. If the first half is equal to the reverse of the second half, the string is a palindrome. If the first half is not equal to the second half, the string is not a palindrome. The following code example shows us how we can check whether a string is a palindrome or not with the String.Substring()
method in C#.
using System;
using System.Linq;
namespace palindrome {
class Program {
public static bool checkPalindrome(string mainString) {
string firstHalf = mainString.Substring(0, mainString.Length / 2);
char[] arr = mainString.ToCharArray();
Array.Reverse(arr);
string temp = new string(arr);
string secondHalf = temp.Substring(0, temp.Length / 2);
return firstHalf.Equals(secondHalf);
}
static void Main(string[] args) {
bool palindrome = checkPalindrome("12321");
Console.WriteLine(palindrome);
}
}
}
Output:
True
In the above code, we checked whether the string 12321
is a palindrome or not with the String.Substring()
method in C#.
Check Palindrome String With the LINQ Method in C#
The SequenceEqual()
method inside the LINQ compares two sequences of elements in C#. The Reverse()
method inside the LINQ reverses the elements of a sequence in C#. We can use the Reverse()
method to reverse our string and compare it with the original string using the SequenceEqual()
method. The following code example shows us how we can check whether a string is a palindrome or not with the LINQ method in C#.
using System;
using System.Linq;
namespace palindrome {
class Program {
public static bool checkPalindrome2(string mainString) {
return mainString.SequenceEqual(mainString.Reverse());
}
static void Main(string[] args) {
bool palindrome = checkPalindrome2("12321");
Console.WriteLine(palindrome);
}
}
}
Output:
True
In the above code, we checked whether the string 12321
is a palindrome or not with the SequenceEqual()
and Reverse()
methods in C#. This approach is preferred over the previous approach because it is simpler.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn