How to Count the List Elements in C#
-
Use the
List.Count
Property to Count the Elements of a List inC#
-
Use the
List.Count()
Method to Count the Elements of a List inC#
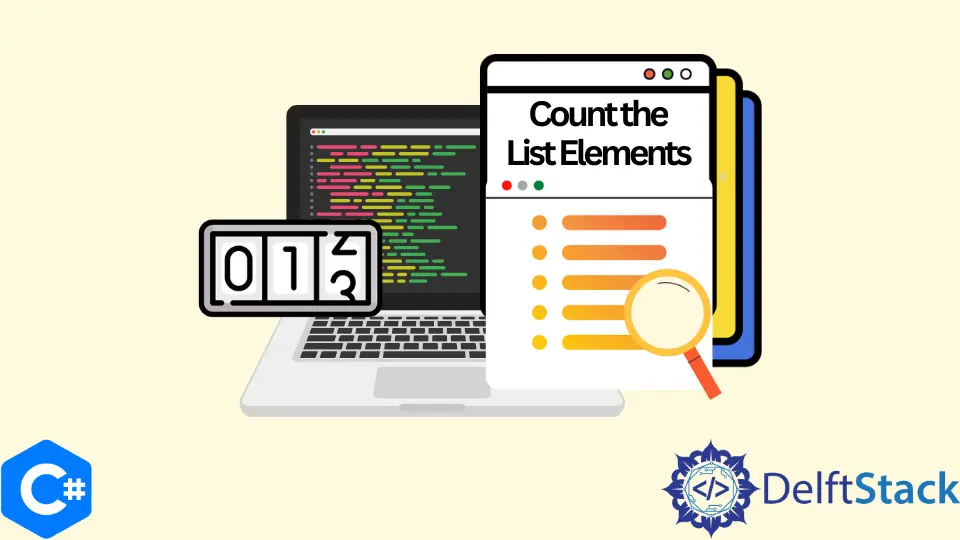
In this tutorial, you will learn the two primary ways to count elements of a list in C#. The first way is using the List.Count
property, and the other is using LINQ List.Count()
method.
A theoretical performance benefit of the Count
property is bypassing the type checking. On the other hand, the Count()
method returns the underlying Count
property as it reveals whether an object is ICollection
(generic) or not.
The List.Count()
method is extremely generic, reusable, and maintainable as it counts the elements of a list. However, performance is the only factor in choosing other methods for a similar purpose.
Overall, the Count()
method is extremely small in programming as it can work on any IEnumerable<>
and can be proven extremely optimized to detect if a Count
property exists and, if so, use that.
Use the List.Count
Property to Count the Elements of a List in C#
The List<T>.Count
property counts the elements contained in the List<T>
. If the count exceeds the capacity of a list while adding the elements, its capacity is automatically increased by reallocating the internal array.
In C#, you can populate a list statically by placing items between tags, programmatically, or from various data source objects such as arrays, SqlDataSource, etc. You can access the Count
property of a list as ListName.Count
from a list control and return an integer value.
Example Code:
// remember to include `System.Collections.Generic` namespace to your C# project
using System;
using System.Collections.Generic;
public class countListElemenets {
public static void Main(string[] args) {
// create a `expList` list of strings
List<string> expList = new List<string>();
// add elements in the `expList` list
for (int i = 1; i < 10; i++) {
int j = i * 2;
string di = j.ToString();
expList.Add(di);
}
// count the elements of `expList` list
Console.WriteLine("Total elements of the list: " + expList.Count);
}
}
Output:
Total elements of the list: 9
In C#, a list can be resized dynamically, and as it accepts null values as valid elements, it allows value duplication. If the values of list elements become equal to their capacity, it can extend its capacity by reallocating the internal array.
The List
class in C# belongs to the System.Collection.Generic
namespace and represents a list of elements/objects which can be accessed by index. It can provide methods to search, sort, and manipulate lists as the lists can create a collection of different data types.
Use the List.Count()
Method to Count the Elements of a List in C#
In LINQ, the Count()
extension method can be used to get the count of the list’s elements. You can implement this method from LINQ in your C# application to maximize its counting efficiency.
It is an extension method of iEnumerable
included in the System.Linq.Enumerable
namespace. The interesting thing about this method is its extended usability which enables it to be used with any collection or a custom class implementing the iEnumerable
interface.
As a list in C# implements iEnumerable
, the List.Count()
method can be used with this collection type. Generally, its returned result will either be an int or a bool, and you need to use the LongCount()
method to return a long
.
The Count()
method takes a predicate delegate parameter of type T
and returns an integer indicating the number of elements in the source sequence that meet the criteria.
Example Code:
// remember to include `System.Collections.Generic` and `System.Linq` namespace to your C# project
using System;
using System.Linq;
using System.Collections.Generic;
public class countListElemenets {
public static void Main(string[] args) {
// create a `expList` list of strings
List<string> expList = new List<string>();
// add elements in the `expList` list
for (int i = 1; i < 10; i++) {
int j = i * 2;
string di = j.ToString();
expList.Add(di);
}
// count the elements of `expList` list
int result = expList.Count();
// display the result
Console.WriteLine("Total elements of the list: " + result);
}
}
Output:
Total elements of the list: 9
You can define criteria so that the Count()
method only counts the elements which meet the criteria. Use <source>.Where(<condition>).Count()
to apply a condition to the Count()
method.
To get the count of a single element, filter the list using the Where()
method to obtain matching values with the specified target, and then get the count by invoking the Count()
method.
Example Code:
// remember to include `System.Collections.Generic` and `System.Linq` namespace to your C# project
using System;
using System.Linq;
using System.Collections.Generic;
public class countListElemenets {
public static void Main(string[] args) {
// create a `expList` list of strings
List<float> expList = new List<float>();
// add elements in the `expList` list
for (int i = 1; i < 10; i++) {
float j = i * 2;
expList.Add(j);
}
// count the elements of the `expList` list in regards to the `Where` condition
int result = expList.Where(x => x.Equals(8)).Count();
// display the result
Console.WriteLine("Total elements of the list: " + result);
}
}
Output:
Total elements of the list: 1
In C#, the list makes life easier as it is dynamic in size, with many methods and properties, and generic in type, which requires <
and >
in its declaration. In this tutorial, you have learned the extremely optimized ways to count the elements of a list in C#.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub