C#에서 목록 요소 계산
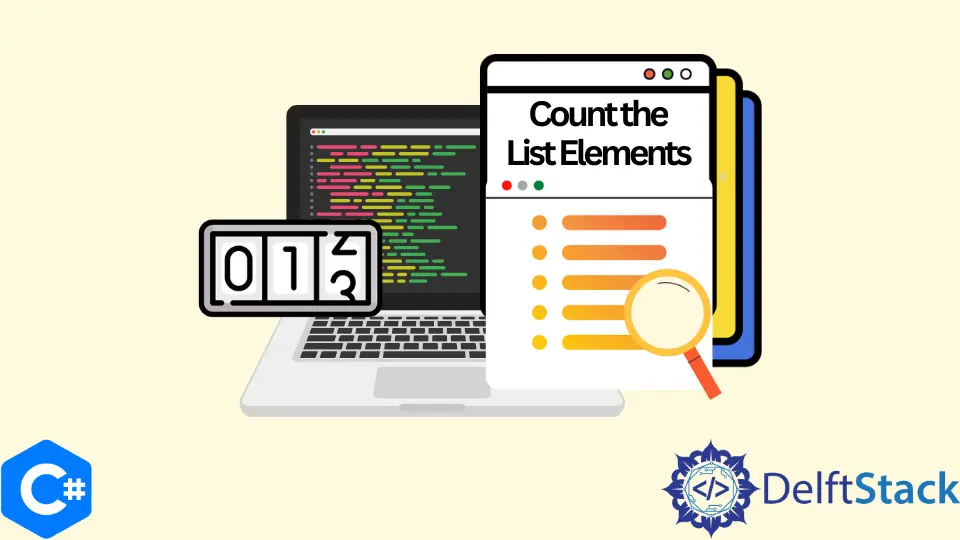
이 자습서에서는 C#에서 목록의 요소를 계산하는 두 가지 기본 방법을 배웁니다. 첫 번째 방법은 List.Count
속성을 사용하는 것이고 다른 하나는 LINQ List.Count()
메서드를 사용하는 것입니다.
Count
속성의 이론적 성능 이점은 유형 검사를 우회하는 것입니다. 반면에 Count()
메서드는 개체가 ICollection
(일반)인지 여부를 나타내기 때문에 기본 Count
속성을 반환합니다.
List.Count()
메서드는 목록의 요소를 계산할 때 매우 일반적이고 재사용 가능하며 유지 관리가 가능합니다. 그러나 유사한 목적을 위해 다른 방법을 선택하는 데 있어서 성능은 유일한 요소입니다.
전반적으로 Count()
메서드는 모든 IEnumerable<>
에서 작동할 수 있으므로 프로그래밍에서 매우 작으며 Count
속성이 존재하는지 감지하고 사용하는 경우 매우 최적화된 것으로 입증될 수 있습니다.
List.Count
속성을 사용하여 C#
에서 목록의 요소 수 계산
List<T>.Count
속성은 List<T>
에 포함된 요소를 계산합니다. 요소를 추가하는 동안 개수가 목록의 용량을 초과하면 내부 배열을 재할당하여 용량을 자동으로 늘립니다.
C#에서는 프로그래밍 방식으로 또는 배열, SqlDataSource 등과 같은 다양한 데이터 원본 개체에서 항목을 태그 사이에 배치하여 정적으로 목록을 채울 수 있습니다. 컨트롤을 나열하고 정수 값을 반환합니다.
예제 코드:
// remember to include `System.Collections.Generic` namespace to your C# project
using System;
using System.Collections.Generic;
public class countListElemenets {
public static void Main(string[] args) {
// create a `expList` list of strings
List<string> expList = new List<string>();
// add elements in the `expList` list
for (int i = 1; i < 10; i++) {
int j = i * 2;
string di = j.ToString();
expList.Add(di);
}
// count the elements of `expList` list
Console.WriteLine("Total elements of the list: " + expList.Count);
}
}
출력:
Total elements of the list: 9
C#에서는 목록의 크기를 동적으로 조정할 수 있으며 null 값을 유효한 요소로 허용하므로 값 복제를 허용합니다. 목록 요소의 값이 해당 용량과 같아지면 내부 배열을 재할당하여 용량을 확장할 수 있습니다.
C#의 List
클래스는 System.Collection.Generic
네임스페이스에 속하며 인덱스로 액세스할 수 있는 요소/개체 목록을 나타냅니다. 목록이 다양한 데이터 유형의 모음을 만들 수 있으므로 목록을 검색, 정렬 및 조작하는 방법을 제공할 수 있습니다.
List.Count()
메서드를 사용하여 C#
에서 목록의 요소 수 계산
LINQ에서 Count()
확장 메서드를 사용하여 목록 요소의 개수를 가져올 수 있습니다. C# 응용 프로그램의 LINQ에서 이 메서드를 구현하여 계산 효율성을 최대화할 수 있습니다.
System.Linq.Enumerable
네임스페이스에 포함된 iEnumerable
의 확장 메서드입니다. 이 메서드의 흥미로운 점은 iEnumerable
인터페이스를 구현하는 사용자 지정 클래스 또는 컬렉션과 함께 사용할 수 있는 확장된 유용성입니다.
C#의 목록은 iEnumerable
을 구현하므로 List.Count()
메서드를 이 컬렉션 유형과 함께 사용할 수 있습니다. 일반적으로 반환된 결과는 int 또는 bool이며 long
을 반환하려면 LongCount()
메서드를 사용해야 합니다.
Count()
메서드는 T
유형의 조건자 위임 매개변수를 사용하고 기준을 충족하는 소스 시퀀스의 요소 수를 나타내는 정수를 반환합니다.
예제 코드:
// remember to include `System.Collections.Generic` and `System.Linq` namespace to your C# project
using System;
using System.Linq;
using System.Collections.Generic;
public class countListElemenets {
public static void Main(string[] args) {
// create a `expList` list of strings
List<string> expList = new List<string>();
// add elements in the `expList` list
for (int i = 1; i < 10; i++) {
int j = i * 2;
string di = j.ToString();
expList.Add(di);
}
// count the elements of `expList` list
int result = expList.Count();
// display the result
Console.WriteLine("Total elements of the list: " + result);
}
}
출력:
Total elements of the list: 9
Count()
메서드가 기준을 충족하는 요소만 계산하도록 기준을 정의할 수 있습니다. <source>.Where(<condition>).Count()
를 사용하여 Count()
메서드에 조건을 적용합니다.
단일 요소의 개수를 가져오려면 Where()
메서드를 사용하여 목록을 필터링하여 지정된 대상과 일치하는 값을 얻은 다음 Count()
메서드를 호출하여 개수를 가져옵니다.
예제 코드:
// remember to include `System.Collections.Generic` and `System.Linq` namespace to your C# project
using System;
using System.Linq;
using System.Collections.Generic;
public class countListElemenets {
public static void Main(string[] args) {
// create a `expList` list of strings
List<float> expList = new List<float>();
// add elements in the `expList` list
for (int i = 1; i < 10; i++) {
float j = i * 2;
expList.Add(j);
}
// count the elements of the `expList` list in regards to the `Where` condition
int result = expList.Where(x => x.Equals(8)).Count();
// display the result
Console.WriteLine("Total elements of the list: " + result);
}
}
출력:
Total elements of the list: 1
C#에서 목록은 많은 메서드와 속성을 포함하는 동적 크기와 선언에 <
및 >
가 필요한 일반 유형이므로 삶을 더 쉽게 만듭니다. 이 자습서에서는 C#에서 목록의 요소를 계산하는 매우 최적화된 방법을 배웠습니다.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub