C# でリスト要素を数える
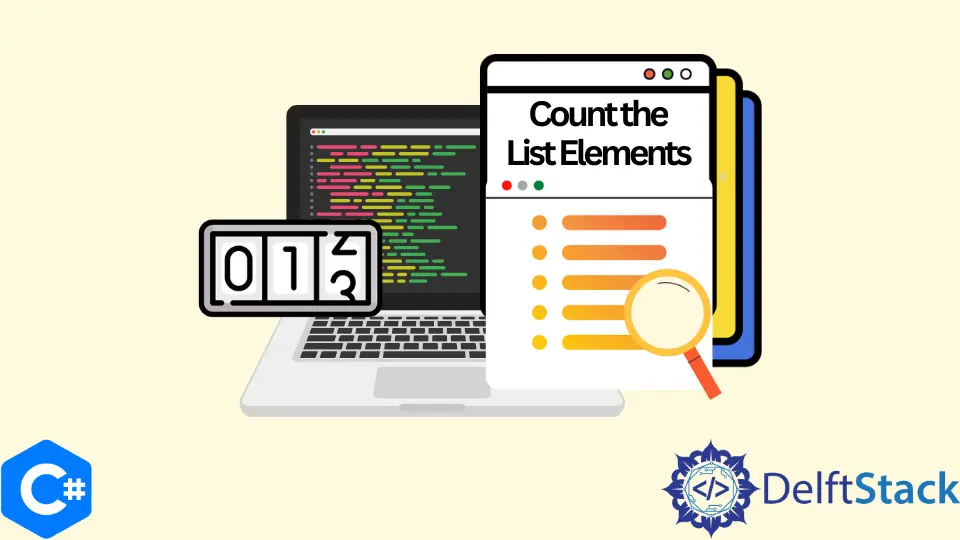
このチュートリアルでは、C# でリストの要素を数える 2つの主な方法を学習します。 最初の方法は List.Count
プロパティを使用する方法で、もう 1つは LINQ List.Count()
メソッドを使用する方法です。
Count
プロパティの理論上のパフォーマンス上の利点は、型チェックをバイパスすることです。 一方、Count()
メソッドは、オブジェクトが ICollection
(ジェネリック) であるかどうかを明らかにするため、基礎となる Count
プロパティを返します。
List.Count()
メソッドは、リストの要素をカウントするため、非常に一般的で、再利用可能で、保守が容易です。 ただし、同様の目的で他の方法を選択する際の唯一の要因はパフォーマンスです。
全体として、Count()
メソッドは、任意の IEnumerable<>
で機能し、Count
プロパティが存在するかどうかを検出し、存在する場合はそれを使用するように非常に最適化されていることが証明できるため、プログラミングにおいて非常に小さくなっています。
List.Count
プロパティを使用して、C#
でリストの要素を数える
List<T>.Count
プロパティは、List<T>
に含まれる要素をカウントします。 要素の追加中にカウントがリストの容量を超えた場合、内部配列を再割り当てすることにより、リストの容量が自動的に増加します。
C# では、タグの間に項目を配置することによって、またはプログラムによって、または配列、SqlDataSource などのさまざまなデータ ソース オブジェクトから、リストを静的に設定できます。 コントロールをリストし、整数値を返します。
コード例:
// remember to include `System.Collections.Generic` namespace to your C# project
using System;
using System.Collections.Generic;
public class countListElemenets {
public static void Main(string[] args) {
// create a `expList` list of strings
List<string> expList = new List<string>();
// add elements in the `expList` list
for (int i = 1; i < 10; i++) {
int j = i * 2;
string di = j.ToString();
expList.Add(di);
}
// count the elements of `expList` list
Console.WriteLine("Total elements of the list: " + expList.Count);
}
}
出力:
Total elements of the list: 9
C# では、リストのサイズを動的に変更でき、null 値を有効な要素として受け入れるため、値の重複が可能です。 リスト要素の値がその容量と等しくなった場合、内部配列を再割り当てすることで容量を拡張できます。
C# の List
クラスは System.Collection.Generic
名前空間に属し、インデックスによってアクセスできる要素/オブジェクトのリストを表します。 リストはさまざまなデータ型のコレクションを作成できるため、リストを検索、並べ替え、および操作するためのメソッドを提供できます。
List.Count()
メソッドを使用して C#
でリストの要素をカウントする
LINQ では、Count()
拡張メソッドを使用して、リストの要素の数を取得できます。 このメソッドを LINQ から C# アプリケーションに実装して、カウント効率を最大化できます。
System.Linq.Enumerable
名前空間に含まれるiEnumerable
の拡張メソッドです。 このメソッドの興味深い点は、任意のコレクションまたは iEnumerable
インターフェイスを実装するカスタム クラスで使用できる拡張された使いやすさです。
C# のリストは iEnumerable
を実装しているため、このコレクション型で List.Count()
メソッドを使用できます。 通常、返される結果は int または bool のいずれかになり、long
を返すには LongCount()
メソッドを使用する必要があります。
Count()
メソッドは、T
型の述語デリゲート パラメーターを取り、基準を満たすソース シーケンス内の要素の数を示す整数を返します。
コード例:
// remember to include `System.Collections.Generic` and `System.Linq` namespace to your C# project
using System;
using System.Linq;
using System.Collections.Generic;
public class countListElemenets {
public static void Main(string[] args) {
// create a `expList` list of strings
List<string> expList = new List<string>();
// add elements in the `expList` list
for (int i = 1; i < 10; i++) {
int j = i * 2;
string di = j.ToString();
expList.Add(di);
}
// count the elements of `expList` list
int result = expList.Count();
// display the result
Console.WriteLine("Total elements of the list: " + result);
}
}
出力:
Total elements of the list: 9
Count()
メソッドが基準を満たす要素のみをカウントするように基準を定義できます。 Count()
メソッドに条件を適用するには、<source>.Where(<condition>).Count()
を使用します。
単一要素のカウントを取得するには、Where()
メソッドを使用してリストをフィルタリングし、指定されたターゲットと一致する値を取得してから、Count()
メソッドを呼び出してカウントを取得します。
コード例:
// remember to include `System.Collections.Generic` and `System.Linq` namespace to your C# project
using System;
using System.Linq;
using System.Collections.Generic;
public class countListElemenets {
public static void Main(string[] args) {
// create a `expList` list of strings
List<float> expList = new List<float>();
// add elements in the `expList` list
for (int i = 1; i < 10; i++) {
float j = i * 2;
expList.Add(j);
}
// count the elements of the `expList` list in regards to the `Where` condition
int result = expList.Where(x => x.Equals(8)).Count();
// display the result
Console.WriteLine("Total elements of the list: " + result);
}
}
出力:
Total elements of the list: 1
C# では、リストはサイズが動的であり、多くのメソッドとプロパティがあり、型がジェネリックであり、宣言に <
と >
が必要なため、作業が楽になります。 このチュートリアルでは、C# でリストの要素をカウントするための非常に最適化された方法を学習しました。
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub