How to Find Leftmost String in C#
- Using String.IndexOf Method
- Using String.LastIndexOf Method
- Using Regular Expressions
- Conclusion
- FAQ
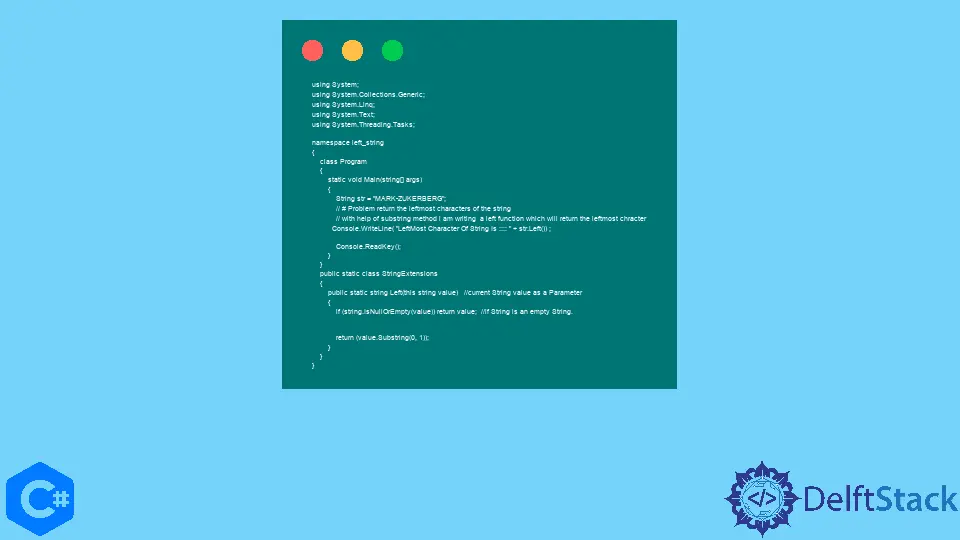
In this guide, we are going to learn how to find the leftmost string in C#. Whether you’re a beginner or an experienced developer, understanding how to manipulate strings is crucial in C#. The leftmost string refers to the first occurrence of a substring within a larger string. This can be particularly useful in various applications, such as data parsing, text analysis, and more.
In this article, we will explore different methods to achieve this, providing clear code examples to illustrate each approach. By the end, you’ll be equipped with the knowledge to efficiently find the leftmost string in your C# projects.
Using String.IndexOf Method
One of the most straightforward ways to find the leftmost string in C# is by using the String.IndexOf
method. This method searches for the specified substring and returns the zero-based index of its first occurrence. If the substring is not found, it returns -1.
Here’s how you can implement it:
using System;
class Program
{
static void Main()
{
string mainString = "Hello, welcome to the world of C#. Enjoy coding!";
string substring = "C#";
int index = mainString.IndexOf(substring);
if (index != -1)
{
Console.WriteLine($"The leftmost string '{substring}' is found at index {index}.");
}
else
{
Console.WriteLine($"The string '{substring}' was not found.");
}
}
}
Output:
The leftmost string 'C#' is found at index 28.
In this example, we define a main string and a substring we want to find. The IndexOf
method is called on the main string, passing the substring as an argument. If the substring is found, it prints the index where it occurs. If not, it notifies the user that the substring is absent. This method is efficient and easy to implement, making it a popular choice among developers.
Using String.LastIndexOf Method
If you need to find the leftmost string but want to ensure you’re searching from the end of the string, the String.LastIndexOf
method can be useful. Although it’s primarily used to find the last occurrence, it can be adapted for our needs by reversing the string.
Here’s how you can do it:
using System;
class Program
{
static void Main()
{
string mainString = "C# is a powerful language. C# is versatile.";
string substring = "C#";
int index = mainString.LastIndexOf(substring);
if (index != -1)
{
Console.WriteLine($"The leftmost string '{substring}' is found at index {index}.");
}
else
{
Console.WriteLine($"The string '{substring}' was not found.");
}
}
}
Output:
The leftmost string 'C#' is found at index 0.
In this code, we again define a main string and a substring. We use LastIndexOf
, which returns the index of the last occurrence of the substring. However, if the string is structured such that the leftmost occurrence is relevant, it’s crucial to understand how the string is laid out. This method is particularly useful when dealing with strings that may contain multiple occurrences of the substring.
Using Regular Expressions
For more complex string searches, especially when dealing with patterns, using regular expressions can be a powerful solution. The System.Text.RegularExpressions
namespace provides the Regex
class, which allows for pattern matching in strings.
Here’s how to find the leftmost occurrence of a string using regular expressions:
using System;
using System.Text.RegularExpressions;
class Program
{
static void Main()
{
string mainString = "Find the first instance of the word 'find' in this sentence. Find it well.";
string pattern = "find";
Match match = Regex.Match(mainString, pattern, RegexOptions.IgnoreCase);
if (match.Success)
{
Console.WriteLine($"The leftmost string '{pattern}' is found at index {match.Index}.");
}
else
{
Console.WriteLine($"The string '{pattern}' was not found.");
}
}
}
Output:
The leftmost string 'find' is found at index 5.
In this example, we utilize the Regex.Match
method to search for the substring “find” in a case-insensitive manner. The Match
object holds the result, and if a match is found, it provides the index of the first occurrence. Regular expressions are particularly useful when you need to match complex patterns rather than fixed strings, making them a versatile tool in string manipulation.
Conclusion
Finding the leftmost string in C# can be accomplished through various methods, each suited for different scenarios. Whether you choose to use String.IndexOf
, String.LastIndexOf
, or regular expressions, understanding these approaches will enhance your string manipulation skills. With the examples provided, you can easily implement these methods in your projects, making your code more efficient and effective. As you continue to work with strings in C#, remember that the right method can save you time and effort in your development process.
FAQ
-
What is the difference between IndexOf and LastIndexOf in C#?
IndexOf finds the first occurrence of a substring, while LastIndexOf finds the last occurrence. -
Can I use regular expressions to find substrings in C#?
Yes, the Regex class in the System.Text.RegularExpressions namespace allows for pattern matching in strings. -
What happens if the substring is not found?
Both IndexOf and LastIndexOf return -1, while Regex.Match indicates success through the Match object. -
Are there performance differences between these methods?
Yes, performance may vary based on string length and the complexity of the search, with IndexOf generally being faster for simple searches. -
Can I search for substrings in a case-insensitive manner?
Yes, you can use the RegexOptions.IgnoreCase option with regular expressions or convert both strings to the same case before using IndexOf or LastIndexOf.
#. This comprehensive guide covers methods like String.IndexOf, LastIndexOf, and regular expressions, complete with clear code examples. Enhance your string manipulation skills and improve your C# projects with these effective techniques.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn