Key-Value Pair List in C#
- The Key-Value Pair as a Data Structure in C#
-
Use
List<KeyValuePair<string, int>>
to Create a Key-Value Pair List in C# -
Use
Dictionary<TKey, TValue>
to Create a Key-Value Pair List in C# -
Use
KeyValuePair<TKey, TValue>[]
Array to Create a Key-Value Pair List in C# -
Use
List<Tuple<TKey, TValue>>
to Create a Key-Value Pair List in C# - Choosing the Right Implementation
- Conclusion
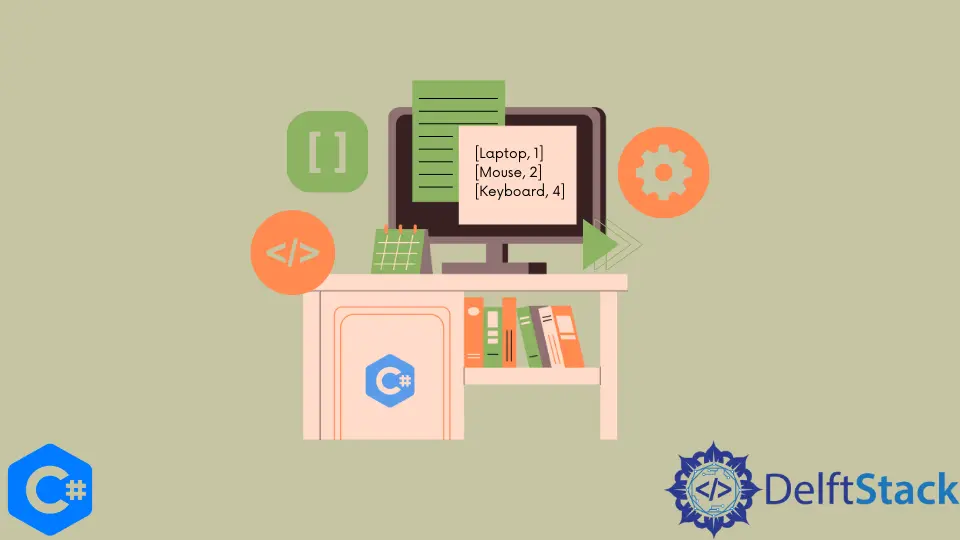
Key-value pair lists play a fundamental role in organizing and managing data efficiently in C# programming. These structures allow developers to map keys to corresponding values, facilitating easy retrieval and manipulation of information.
In C#, there are several methods to implement key-value pair lists, each with unique functionalities and best-fit scenarios. If you have coded previously, you must be familiar with key-value pairs used in data structures like maps, dictionaries, etc.
C# did something special with it, and it provides us with a dedicated class for key-value pairs. We can use them just like any other data structure.
In this tutorial, we will learn about the properties of key-value pairs in C# and see how to create a key-value pair list.
The Key-Value Pair as a Data Structure in C#
At its core, a key-value pair list associates a unique key with a specific value. The key acts as an identifier, allowing rapid access to the associated value.
This structure is crucial in various programming tasks, ranging from data storage and retrieval to organizing information in dictionaries and associative arrays.
A key-value pair is a data structure or, more precisely, a generic struct defined in System.Collections.Generic
that holds two related items. They can have different or similar data types.
They can also be a key-value pair. The key is a unique identifier of the data, synonymous with the primary key in a database.
There can be only one key but multiple values corresponding to that key. This property helps create a mapping between two entities, making them useful in data structures like Dictionary, HashMap, etc.
Use List<KeyValuePair<string, int>>
to Create a Key-Value Pair List in C#
The List<T>
class in C# provides a dynamic and resizable array to store elements of a specified type. Utilizing this class in combination with the KeyValuePair<TKey, TValue>
structure enables developers to create lists specifically tailored to manage pairs of string keys and integer values.
Syntax:
List<KeyValuePair<string, int>>
This syntax represents a declaration of a list that contains elements of the type KeyValuePair<string, int>
. Here’s a breakdown of the syntax:
List<>
: This is a generic collection type in C# that represents a list. It allows you to store and manipulate a collection of items of a specific type.KeyValuePair<string, int>
: This is a data structure that holds a pair of values - a key of typestring
and a value of typeint
. In this context, it’s used as the type of elements that the list will contain.
So, when List<KeyValuePair<string, int>>
is used, it creates a dynamic list that can hold elements, and in this case, specifically holds pairs of strings as keys and integers as values.
The code below initializes a List
of KeyValuePair<string, int>
, populating it with three key-value pairs: "Laptop"
mapped to 1
, "Mouse"
mapped to 2
, and "Keyboard"
mapped to 4
. The foreach
loop iterates through each element in the list, printing each key-value pair to the console.
However, the output would display the ToString()
representation of the KeyValuePair
object, which includes both the key and the value in a formatted string. Therefore, the output would be something like representing the three key-value pairs stored in the list.
using System;
using System.Collections.Generic;
class Program {
static void Main() {
var list = new List<KeyValuePair<string, int>>();
list.Add(new KeyValuePair<string, int>("Laptop", 1));
list.Add(new KeyValuePair<string, int>("Mouse", 2));
list.Add(new KeyValuePair<string, int>("Keyboard", 4));
foreach (var element in list) {
Console.WriteLine(element);
}
}
}
Output:
[Laptop, 1]
[Mouse, 2]
[Keyboard, 4]
The above example shows that creating a key-value pair List is no different from any other list creation. The unique thing is the ability to store a pair of information together.
Use Dictionary<TKey, TValue>
to Create a Key-Value Pair List in C#
The Dictionary<TKey, TValue>
class in C# represents a collection of keys and values stored as pairs, where each key is unique. This structure enables developers to store and retrieve values based on their associated keys with exceptional efficiency, making it a fundamental tool for handling key-value pairs.
The syntax for using Dictionary<TKey, TValue>
in C# is:
Dictionary<TKey, TValue> dictionaryName = new Dictionary<TKey, TValue>();
Where:
Dictionary<TKey, TValue>
: This is the generic class in C# used to create a collection of key-value pairs. TheTKey
represents the type of keys stored in the dictionary, andTValue
represents the type of values associated with these keys.dictionaryName
: This is the name given to the instance of the dictionary you’re creating. You can replacedictionaryName
with any meaningful identifier that suits your context.
The Dictionary<TKey, TValue>
is a widely used data structure in C# that represents a collection of key-value pairs. It provides fast access to values based on their keys, making it highly efficient for large datasets.
using System;
using System.Collections.Generic;
class Program {
static void Main() {
// Creating a Dictionary
Dictionary<string, int> keyValuePairs = new Dictionary<string, int>();
// Adding key-value pairs
keyValuePairs.Add("One", 1);
keyValuePairs.Add("Two", 2);
keyValuePairs.Add("Three", 3);
// Accessing values by keys
Console.WriteLine(keyValuePairs["Two"]); // Output: 2
// Iterating through key-value pairs
foreach (var pair in keyValuePairs) {
Console.WriteLine($"Key: {pair.Key}, Value: {pair.Value}");
}
}
}
Output:
2
Key: One, Value: 1
Key: Two, Value: 2
Key: Three, Value: 3
This code demonstrates the use of a Dictionary<string, int>
to manage key-value pairs. Initially, an empty dictionary named keyValuePairs
is created to store string keys mapped to integer values.
Three key-value pairs are added using the Add
method: "One"
with a value of 1
, "Two"
with a value of 2
, and "Three"
with a value of 3
.
The code showcases key-based value retrieval using Console.WriteLine(keyValuePairs["Two"])
, which outputs the value associated with the key "Two"
(resulting in 2
).
Lastly, a foreach
loop is utilized to iterate through the dictionary, printing each key-value pair’s key and value to the console using string interpolation.
Use KeyValuePair<TKey, TValue>[]
Array to Create a Key-Value Pair List in C#
Another approach involves using an array of KeyValuePair<TKey, TValue>
, allowing for a collection of key-value pairs within an array structure.
The KeyValuePair<TKey, TValue>[]
array represents an indexed collection of key-value pairs, where each element consists of a unique key of type TKey
and an associated value of type TValue
. This structure allows developers to create arrays specifically tailored to manage pairs of data in an ordered sequence.
The syntax for using KeyValuePair<TKey, TValue>[]
arrays in C# is:
KeyValuePair<TKey, TValue>[] arrayName = new KeyValuePair<TKey, TValue>[arraySize];
Where:
KeyValuePair<TKey, TValue>[]
: This represents an array ofKeyValuePair
structures.TKey
andTValue
denote the types of the keys and values in the key-value pairs, respectively.arrayName
: This is the identifier given to the array instance you’re creating. You can replacearrayName
with any meaningful name that suits your context.arraySize
: This is the size of the array, i.e., the number of elements the array will contain. It’s specified within square brackets during array initialization.
Example:
using System;
using System.Collections.Generic;
class Program {
static void Main() {
// Creating an array of KeyValuePairs
var keyValuePairs =
new[] { new KeyValuePair<string, int>("One", 1), new KeyValuePair<string, int>("Two", 2),
new KeyValuePair<string, int>("Three", 3) };
// Accessing values by keys
foreach (var pair in keyValuePairs) {
Console.WriteLine($"Key: {pair.Key}, Value: {pair.Value}");
}
}
}
Output:
Key: One, Value: 1
Key: Two, Value: 2
Key: Three, Value: 3
This code snippet illustrates the creation and utilization of an array of KeyValuePair<string, int>
elements. Initially, an array named keyValuePairs
is initialized using the shorthand syntax for array creation and initialization.
This array holds three elements, each being a KeyValuePair<string, int>
. Each KeyValuePair
within the array represents a distinct key-value pair, where the string keys ("One"
, "Two"
, and "Three"
) are paired with corresponding integer values (1
, 2
, and 3
).
Subsequently, a foreach
loop is utilized to iterate through the array, retrieving each KeyValuePair
element and printing its key and value to the console using string interpolation. This displays all the key-value pairs stored in the array, showcasing their keys and associated values to the console output.
Use List<Tuple<TKey, TValue>>
to Create a Key-Value Pair List in C#
The List<Tuple<TKey, TValue>>
structure represents a dynamic list that stores tuples, where each tuple holds a key-value pair. A tuple is an ordered set of elements, and in this case, it consists of a TKey
representing the key and a TValue
representing the associated value.
This structure enables developers to create lists specifically tailored to manage pairs of data sequentially. Utilizing a List
of Tuple
offers another avenue to store key-value pairs.
The syntax for using List<Tuple<TKey, TValue>>
in C# is as follows:
List<Tuple<TKey, TValue>> listName = new List<Tuple<TKey, TValue>>();
Where:
List<Tuple<TKey, TValue>>
: This denotes the creation of aList
containing tuples with keys of typeTKey
and values of typeTValue
.TKey
represents the type of keys stored in the tuple, andTValue
represents the type of values associated with these keys.listName
: This is the identifier given to the instance of the list you’re creating. You can replacelistName
with any meaningful name that suits your context.
Example usage:
using System;
using System.Collections.Generic;
class Program {
static void Main() {
// Creating a List of Tuples
var keyValuePairs =
new List<Tuple<string, int>> { Tuple.Create("One", 1), Tuple.Create("Two", 2),
Tuple.Create("Three", 3) };
// Accessing values by keys
foreach (var pair in keyValuePairs) {
Console.WriteLine($"Key: {pair.Item1}, Value: {pair.Item2}");
}
}
}
Output:
Key: One, Value: 1
Key: Two, Value: 2
Key: Three, Value: 3
This code snippet showcases the creation and utilization of a List<Tuple<string, int>>
. Initially, a List
named keyValuePairs
is instantiated, specifically defined to hold tuples where each tuple consists of a string
representing a key and an int
representing the associated value.
The List
is initialized using the shorthand syntax for list creation and initialization. Three tuples are added directly during initialization using Tuple.Create
, where each tuple represents a distinct key-value pair: "One"
mapped to 1
, "Two"
mapped to 2
, and "Three"
mapped to 3
.
Following the initialization, a foreach
loop is employed to iterate through each tuple in the list. Inside the loop, the keys and values are accessed using the Item1
and Item2
properties of each tuple, respectively, and are printed to the console using string interpolation.
This outputs all the key-value pairs stored in the list, displaying their keys and associated values in the console output.
Choosing the Right Implementation
Each method comes with its advantages and best-fit scenarios. The Dictionary<TKey, TValue>
offers fast access and efficient storage for large datasets.
The array of KeyValuePair<TKey, TValue>
provides simplicity and ease of initialization. Meanwhile, the List<Tuple<TKey, TValue>>
caters to scenarios where a list structure is preferred.
When choosing the appropriate implementation, consider factors like data size, access patterns, and ease of manipulation.
For large datasets requiring frequent access by keys, the Dictionary<TKey, TValue>
might be the optimal choice. And for simpler or smaller collections, arrays or lists might suffice.
Applications in Creating a Key-Value Pair List in C#
- A list of Key-Value pairs can be used to construct a Linked List with a key holding the data and value the link to the next node.
- In returning non-singular info that can have a pair or even more than a pair of values.
- It can be used in bulk offline querying of information and efficient storing of the results.
Conclusion
In conclusion, understanding the various methods of implementing key-value pair lists in C# is pivotal for efficient data management and retrieval. By exploring these methodologies - from dictionaries to arrays and lists - developers gain the flexibility to tailor their data structures according to specific project requirements.
These versatile implementations empower programmers to handle diverse datasets efficiently, ensuring optimal performance and ease of maintenance within their C# applications.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn