How to Convert Double to String in C#
-
Use
ToString()
to Convertdouble
tostring
in C# -
Use
String.Format
to Convertdouble
tostring
in C# -
Use String Interpolation to Convert
double
tostring
in C# - Conclusion
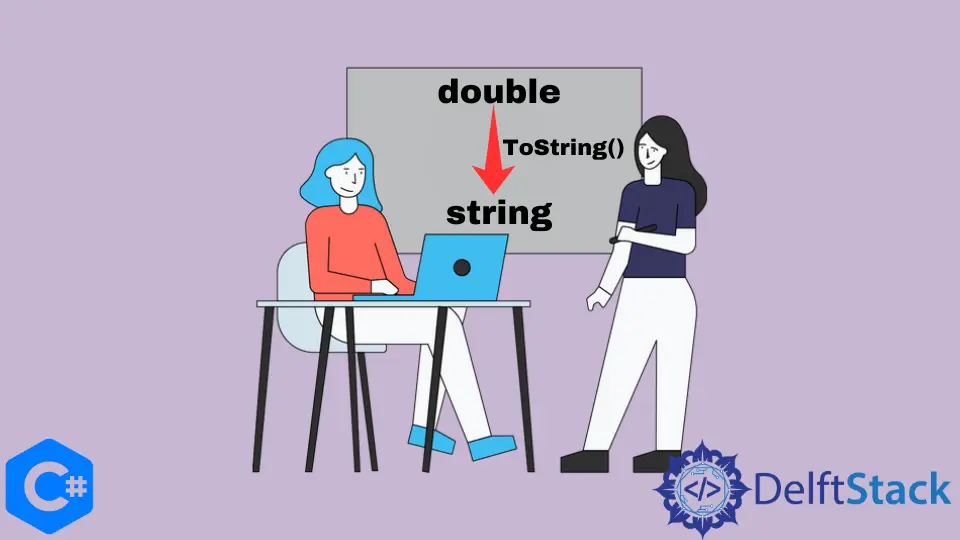
Converting a double
to a string
is a common operation in C#, essential for scenarios such as displaying numeric data in user interfaces, logging, or formatting output. In C#, there are multiple methods to achieve this conversion, each with its advantages and use cases.
In this article, we will explore three widely used methods: ToString()
, String.Format
, and string interpolation.
Use ToString()
to Convert double
to string
in C#
One straightforward and commonly used method to convert numerical data types, such as double
, into string
representations is the ToString()
method.
The ToString()
method is a versatile and fundamental method available for all objects in C#, including numerical types like double
.
Syntax:
string strRepresentation = yourDoubleVariable.ToString();
To convert yourDoubleVariable
to a string
, we call the ToString()
method on this variable and store the result in a string
variable named strRepresentation
.
Let’s consider a practical example where we have a double
variable named myDouble
that we want to convert to a string
using the ToString()
method:
using System;
class Program {
static void Main() {
double myDouble = 0.09434;
string myString = myDouble.ToString();
Console.WriteLine("Double Value: " + myDouble);
Console.WriteLine("String Representation: " + myString);
}
}
In this code, we begin by declaring a double
variable named myDouble
and initializing it with the value 0.09434
. The next line demonstrates the conversion process using the ToString()
method:
string myString = myDouble.ToString();
Here, myDouble
is the double
variable we wish to convert, and the ToString()
method is invoked on it. This method transforms the double
value into its string
representation, and the result is stored in the myString
variable.
Subsequently, we use Console.WriteLine
to display both the original double
value and its string
representation:
Console.WriteLine("Double Value: " + myDouble);
Console.WriteLine("String Representation: " + myString);
When we run this program, it will output:
Double Value: 0.09434
String Representation: 0.09434
The ToString()
method is a simple yet powerful tool for converting double
values into string
representations in C#. Its application is not limited to double
; it can be employed for various data types, providing a flexible solution for string
conversion needs.
Use String.Format
to Convert double
to string
in C#
In addition to the ToString()
method, C# offers another powerful tool for converting a double
to a string
through the String.Format
method. This method provides a more flexible way to control the format of the resulting string
, allowing for precise customization of numeric representations.
The String.Format
method allows you to create a composite format string
where you can embed placeholders for variables to be inserted. When applied to a double
, it formats the numeric value according to the specified format string
.
Here’s a simple example:
using System;
class Program {
static void Main() {
double myDouble = 3.14;
string myString = string.Format("{0}", myDouble);
Console.WriteLine("String: " + myString);
}
}
Output:
String: 3.14
In the example above, we declare a double
variable named myDouble
with the value 3.14
. To convert this numeric value to a string
using String.Format
, we call the string.Format
method and provide a format string as the first argument.
The format string contains a placeholder {0}
, which indicates the position where the myDouble
value will be inserted.
The resulting myString
variable will now hold the value "3.14"
as a string
. This method allows for more complex formatting scenarios where you can control not only the placement of variables but also their format.
For instance, you can format the string
with a specified number of decimal places like this:
using System;
class Program {
static void Main() {
double myDouble = 3.14159265359;
string formattedString = string.Format("{0:F2}", myDouble);
Console.WriteLine("String: " + formattedString);
}
}
Output:
String: 3.14
In this case, the :F2
format specifier within the placeholder {0}
instructs String.Format
to format the myDouble
value with two decimal places.
Use String Interpolation to Convert double
to string
in C#
String interpolation is a concise and expressive way to create a string
in C#. Introduced in C# 6.0, it simplifies the process of embedding expressions directly into string literals.
When it comes to converting a double
to a string
, string interpolation provides an elegant alternative to the more traditional methods like ToString()
and String.Format
.
The syntax for string interpolation involves placing the expression to be evaluated inside curly braces {}
within a string
. When applied to a double
, the expression is replaced with the string
representation of the numeric value.
Here’s an example:
using System;
class Program {
static void Main() {
double myDouble = 3.14;
string myString = $"{myDouble}";
Console.WriteLine($"Original Double: {myDouble}");
Console.WriteLine($"Converted String: {myString}");
}
}
Output:
Original Double: 3.14
Converted String: 3.14
In the example above, we declare a double
variable named myDouble
with the value 3.14
. To convert this numeric value to a string
using string interpolation, we create a string literal using the $
symbol followed by curly braces {}
.
Inside the curly braces, we place the expression myDouble
, and during execution, this expression is replaced with the string
representation of the myDouble
value.
The resulting myString
variable will now hold the value "3.14"
as a string
. String interpolation is not only concise but also improves code readability by directly embedding expressions into the string
where they logically belong.
You can also apply format specifiers within the curly braces to control the formatting of the numeric value:
using System;
class Program {
static void Main() {
double myDouble = 3.14159265359;
string formattedString = $"{myDouble:F2}";
Console.WriteLine($"Original Double: {myDouble}");
Console.WriteLine($"Formatted String: {formattedString}");
}
}
In this example, :F2
specifies that the myDouble
value should be formatted with two decimal places.
Output:
Original Double: 3.14159265359
Formatted String: 3.14
Conclusion
Converting a double
to a string
in C# is a fundamental operation in many applications. Understanding the various methods available allows you to choose the one that best fits your specific needs.
Whether you opt for the simplicity of ToString()
, the formatting control of String.Format
, or the expressiveness of string interpolation, each method provides a reliable way to convert a double
value to string
in C#.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn