How to Compress and Decompress a String in C#
- Definition of Compression
-
the .NET Data Compression Algorithms in
C#
-
Use
GZip
to Compress a String inC#
-
Use
GZip
to Decompress a String inC#
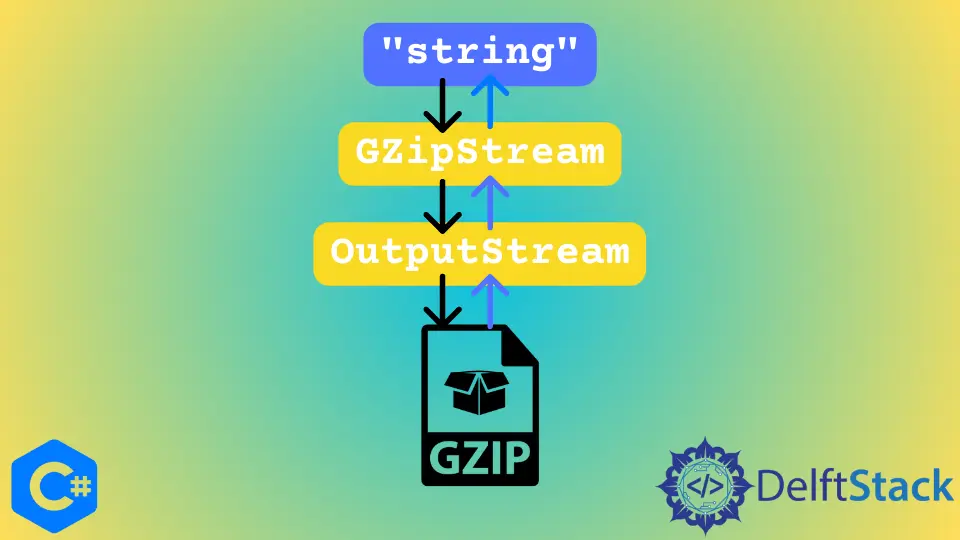
This article will demonstrate how we can apply compression methods to the System.IO
file system.
The Compression
namespace can compress and decompress a string’s value. Compressing the values should result in a considerable size reduction in bytes.
Definition of Compression
In Physics, compression refers to a size decrease resulting from forces acting inwards on a mass. When we talk about data compression, we refer to changing data into a more compact format without any discernible loss of content.
Data compression is the process of encoding already-existing information into as few bits as is humanly practical using algorithms. The efficacy of various algorithms varies to varying degrees.
Still, they generally involve compromise in terms of the time needed to compress the data or the amount of processing power required by the CPU.
the .NET Data Compression Algorithms in C#
There are many alternative compression methods, but for this discussion’s sake, we will concentrate on GZip
. Although it is feasible to utilize a third-party library such as SharpZipLib
, we will use the GZipStream
class native to the .NET Framework and found in the System.IO.Compression
namespace.
Additionally, we will strongly emphasize compressing and decompressing string data; the procedures for dealing with other kinds, such as byte arrays and streams, will be somewhat modified.
Use GZip
to Compress a String in C#
The most basic implementation of GZipStream
requires users to provide an underlying stream and a compression option as inputs. Compression mode dictates whether you want to compress or decompress the data; the underlying stream is changed based on the compression method.
The code below uses a memory stream as our underlying output stream. The output stream is wrapped in a GZipStream
container.
When we send our input data into the GZipStream
, the data travels down the pipeline to the output stream in its compressed form. We may guarantee that the data is cleaned up by placing the Write
action in its own using
block.
The save()
method code is available in the source code at the end of this article.
public static byte[] Compress(string str) {
var bytes = Encoding.UTF8.GetBytes(str);
using (var msi = new MemoryStream(bytes)) using (var memoryStream = new MemoryStream()) {
using (var gZipStream = new GZipStream(memoryStream, CompressionMode.Compress)) {
save(msi, gZipStream);
}
return memoryStream.ToArray();
}
}
Use GZip
to Decompress a String in C#
When decompressing data, the stream being decompressed becomes an input stream. The GZipStream
will continue to enclose it, but the flow will now be reversed such that reading data from the GZipStream
will convert the compressed data into uncompressed data.
The CompressionMode.Decompress
mode is used to decompress a string.
public static string Decompress(byte[] bytes) {
using (var msi = new MemoryStream(bytes)) using (var memoryStream = new MemoryStream()) {
using (var gZipStream = new GZipStream(msi, CompressionMode.Decompress)) {
save(gZipStream, memoryStream);
}
return Encoding.UTF8.GetString(memoryStream.ToArray());
}
}
Complete Source Code to Compress and Decompress a String in C#
using System;
using System.IO;
using System.IO.Compression;
using System.Text;
class HelloWorld {
public static void save(Stream source, Stream destination) {
byte[] bytes = new byte[4096];
int count;
while ((count = source.Read(bytes, 0, bytes.Length)) != 0) {
destination.Write(bytes, 0, count);
}
}
public static byte[] Compress(string str) {
var bytes = Encoding.UTF8.GetBytes(str);
using (var msi = new MemoryStream(bytes)) using (var memoryStream = new MemoryStream()) {
using (var gZipStream = new GZipStream(memoryStream, CompressionMode.Compress)) {
save(msi, gZipStream);
}
return memoryStream.ToArray();
}
}
public static string Decompress(byte[] bytes) {
using (var msi = new MemoryStream(bytes)) using (var memoryStream = new MemoryStream()) {
using (var gZipStream = new GZipStream(msi, CompressionMode.Decompress)) {
save(gZipStream, memoryStream);
}
return Encoding.UTF8.GetString(memoryStream.ToArray());
}
}
static void Main(string[] args) {
byte[] string1 = Compress("stringstringstringstringstringstringstringstring");
Console.WriteLine("Zipped Size: " + string1.Length + " bytes");
string string2 = Decompress(string1);
Console.WriteLine("Unzipped Size: " + string2.Length + " bytes");
}
}
Output:
Zipped Size: 29 bytes
Unzipped Size: 48 bytes
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn