Carriage Return in C#
-
Use Parameter-Less
Console.WriteLine()
to Add a New Line inC#
-
Inject New Lines Within the Same String in
C#
-
Use
Environment.NewLine
inC#
-
Use the ASCII Literal of a New Line in
C#
-
Use the
\r\n
Character to Insert a New Line inC#
-
Insert New Lines in ASP.NET for an Existing String in
C#
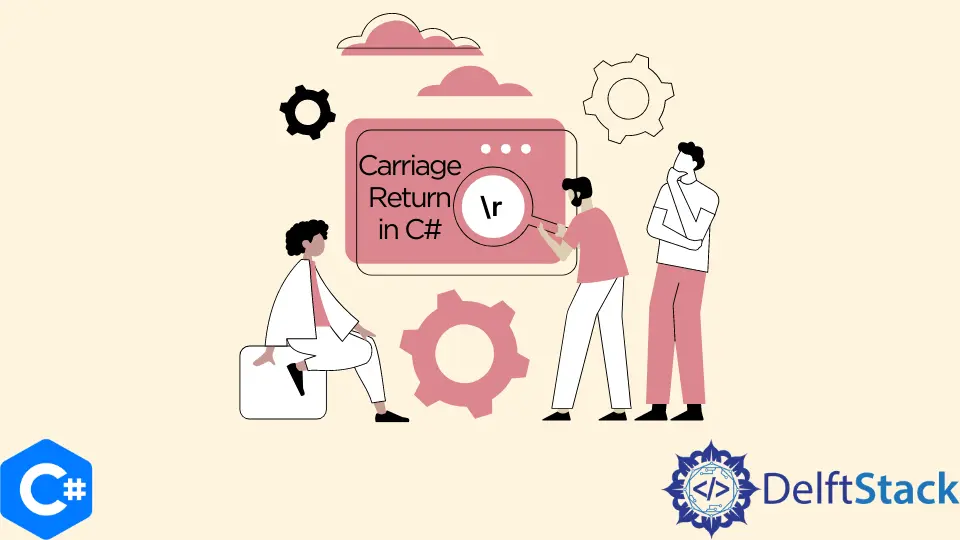
This article will explain how to produce a carriage return in a string using the C# programming language. Creating a carriage return in the C# string may be accomplished differently.
The following techniques, which all operate similarly to construct a carriage return in the C# string, are examined and implemented.
Use Parameter-Less Console.WriteLine()
to Add a New Line in C#
Using a parameterless console.WriteLine()
is the simplest approach to adding a new line to a console program. For instance:
Console.WriteLine("This is a line");
Console.WriteLine();
Console.WriteLine("This is the second line");
Output:
This is a line
This is the second line
To use the Console.WriteLine()
method without any arguments results in a line terminator, as seen above in Microsoft’s documentation. As a convenience, the WriteLine()
function inserts a single line break between each set of two lines.
In addition to the default spacing, you may alter this setting to suit your needs. For example, the Console.WriteLine()
method may be rewritten to print two lines instead of a single one.
Code like the following might take care of the problem.
Console.Out.NewLine = "\r\n\r\n";
Console.WriteLine("This is the first line");
Console.WriteLine();
Console.WriteLine("This is the second line");
Consoles may use the above code. Insert two lines by using WriteLine()
.
A string might thus be passed in this manner. The current input string will be extended by one line when you use WriteLine()
.
The console, on the other hand, has no parameters. Instead of only one line, WriteLine()
will now input two.
Use this code, and you’ll get something like this.
This is the first line
This is the second line
Take notice that after the first console, there is another line. Use WriteLine()
for the second console, then two blank lines.
There is a single blank line after the third call to WriteLine()
.
Inject New Lines Within the Same String in C#
Putting \n
anywhere inside your string is the second option to add a new line to an existing string. For example:
Console.WriteLine("This is a line.\nThis is the second line.");
The above code will run as shown below.
This is a line.
This is the second line.
As noticed, \n
could successfully insert a new line inside the same string.
A single backslash performs a particular action or function with escape sequence and characters. When inserted within a code string literal, an escape sequence represents everything except itself double-quoted.
Escape sequences may be translated. String mistakes cause an escaped sequence problem. \t
tabs, \a
beeps.
Use Environment.NewLine
in C#
\r\n
is the Windows equivalent of a newline. There are a few ways to insert newlines in a C# string, and the optimal one depends on your software.
If you want to start a new line in a string, you may use Environment.NewLine
. For instance:
Console.WriteLine("This is a line." + Environment.NewLine + "This is the second line.");
Output:
This is a line.
This is the second line.
The above code will produce the same result as the previous result.
A new line, line feed, line break, carriage return, CRLF
, and end of the line signify the same thing when used in conjunction with NewLine
’s features. A newline character such as the escape characters \r
and \n
or vbCrLf
(in Visual Basic) may be used with NewLine
in combination with language-specific newline support Microsoft C# and C/C++.
The console automatically inserts a new line after any text it has processed. WriteLine
and the StringBuilder
class methods for adding lines.
Use the ASCII Literal of a New Line in C#
This method is similar to the \n
. On the other hand, you might use the literal ASCII character that denotes a new line instead.
For example:
Console.WriteLine("This is a line. \x0AThis is the second line.");
Output:
This is a line.
This is the second line.
This ASCII literal for a new line, x0A
, will likewise insert a new line into the text seen above.
Use the \r\n
Character to Insert a New Line in C#
You may inject \r\n
into a string to introduce a new line. For example:
Console.WriteLine("This is a line. \r\nThis is the second line.");
Output:
This is a line.
This is the second line.
The code above correctly inserts a new line with the use of \r\n
. Is there a significant difference between the two terms, \r\n
and \n
?
\n
stands for a new line, and \r
stands for carriage return, which moves the cursor to the far left of the document. To print from the beginning of a line from the left, you need to use \n
for Line Feed and \r
for Carriage Return to the most left position; this is from ancient computers syntax preserved on the Windows platform.
\r\n
is a Windows Style\n
is a POSIX Style\r
is an old pre-OS X Macs Style, Modern Mac’s using POSIX Style
Insert New Lines in ASP.NET for an Existing String in C#
Line breaks in HTML or ASP.NET are represented by the <br/>
tag. It is often necessary to transform an existing string that contains newlines into HTML code that includes <br/>
tags.
You may use regular expressions to convert a string’s new lines to HTML line breaks using the following approach.
public static string ConvertToLineBreaks(this string inputString) {
Regex regEx = new Regex(@"(\\n|\\r)+");
return regEx.Replace(inputString, "");
}
The approach shown above is intended to be used as an extension method. Include the method in a separate file, then use a using
statement to include it in your code.
Finally, call the method as follows.
string html = inputString.ConvertToLineBreaks();
You can test the above regular expression here.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn