How to Append to Text File in C#
-
Append to a Text File With the
File.AppendAllText()
Method in C# -
Append to a Text File With the
StreamWriter
Class in C# -
Append to a Text File With the
File.AppendText()
Method in C# - Conclusion
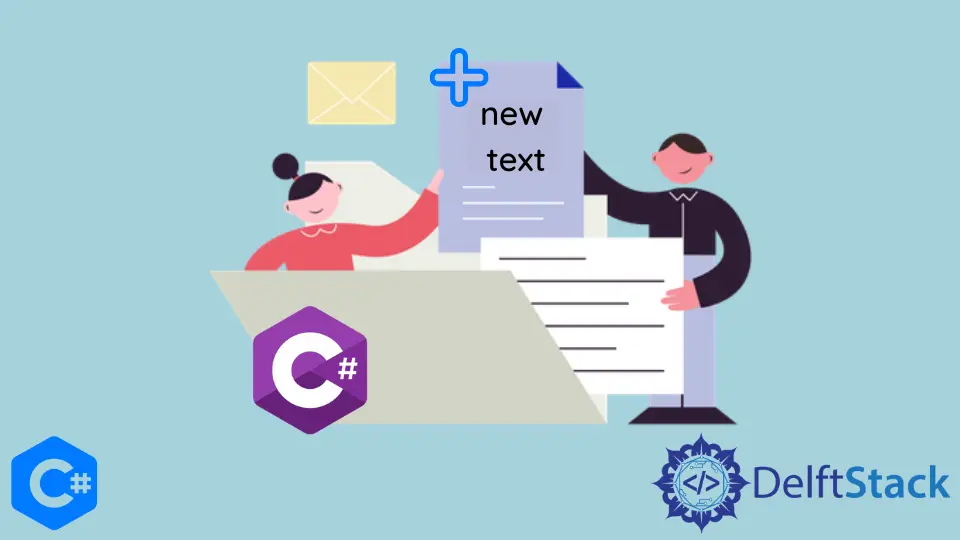
In this comprehensive guide, we delve into the practical aspects of appending text to files in C# – a fundamental skill for any developer working with file I/O operations in the .NET framework. Specifically, we explore three distinct methods: the File.AppendAllText()
method, the StreamWriter
class, and the File.AppendText()
method.
Each method offers its unique approach to appending text, and through detailed examples, we demonstrate the usage and nuances of these methods. Our aim is to provide clear, executable examples that not only elucidate the syntax but also highlight best practices in resource management and error handling in C# file manipulation.
Append to a Text File With the File.AppendAllText()
Method in C#
The File.AppendAllText()
method in C# is used to open an existing file, append all the text to the end of the file and then close the file. If the file does not exist, the File.AppendAllText()
method creates a new empty file and writes the data in it.
The File.AppendAllText()
method takes the file path and the text to be written as its arguments. The following code example shows us how to append data to a text file with the File.AppendAllText()
method in C#.
using System;
using System.IO;
class Program {
static void Main() {
string filePath = "example.txt";
string textToAppend = "Hello, World!\n";
File.AppendAllText(filePath, textToAppend);
Console.WriteLine("Text appended to the file successfully.");
}
}
We begin by importing the System.IO
namespace, housing the File
class.
filePath
is designated to store the path leading to the text file where we intend to append text. The string textToAppend
contains the content we wish to add to the file.
We invoke the File.AppendAllText
method, passing filePath
and textToAppend
as arguments. This method takes care of the entire process, handling the opening of the file, writing the text, and subsequently closing the file.
If the file specified in filePath
does not exist, it is automatically created.
Finally, we utilize Console.WriteLine
to present a message on the console, confirming the successful completion of the operation.
example.txt
before running code:
this is all the text in this file \n
Output:
Append to a Text File With the StreamWriter
Class in C#
We can achieve the same goal with the StreamWriter
class. The StreamWriter
class is used to write text to a stream or a file in C#.
The SreamWriter.WriteLine()
method writes a whole line in C#. We can initialize an object of the StreamWriter
class with the File.AppendText()
method to initialize an instance of the StreamWriter
class that would append the data to the file.
The following code example shows us how we can append data to the end of a text file with the StreamWriter
class in C#.
using System;
using System.IO;
class Program {
static void Main() {
string filePath = "example.txt";
string textToAppend = "Hello, World!\n";
using (StreamWriter writer = new StreamWriter(filePath, true)) {
writer.WriteLine(textToAppend);
}
Console.WriteLine("Text appended to the file successfully.");
}
}
We begin by including the System.IO
namespace, essential for our file I/O operations. We define filePath
with the path leading to our target file.
The string textToAppend
is what we intend to add to the file.
Next, we instantiate the StreamWriter
using filePath
and set the second parameter to true
. This setting activates the appending mode. With writer.WriteLine
, we proceed to write our string to the file, appending a new line at the end.
Crucially, we enclose the StreamWriter
in a using
statement. This ensures proper disposal of the StreamWriter
, which in turn automatically takes care of flushing and closing the file.
Finally, upon completing the operation, we print a confirmation message to the console, signaling that the text has been successfully appended.
example.txt
before running code:
this is all the text in this file \n
Output:
Append to a Text File With the File.AppendText()
Method in C#
In the world of C# programming, file manipulation is a fundamental skill. One common task is appending text to an existing file, which can be seamlessly achieved using the File.AppendText
method.
This method is part of the System.IO
namespace and provides a simple yet powerful way to add content to the end of a file.
using System;
using System.IO;
class Program {
static void Main() {
string filePath = "example.txt";
string textToAppend = "Added line.\n";
using (StreamWriter sw = File.AppendText(filePath)) {
sw.WriteLine(textToAppend);
}
Console.WriteLine("Text has been appended to the file.");
}
}
We begin by importing the System.IO
namespace. Our filePath
variable contains the path to the text file. The string textToAppend
holds the content that will be added to the file.
We invoke File.AppendText
with the filePath
, which returns a StreamWriter
object.
We use the StreamWriter.WriteLine
method to write the textToAppend
to the file, including a new line character at the end. To ensure proper disposal of resources and the closure of the file once the operation is complete, we enclose the StreamWriter
object within a statement.
Finally, we print a message to the console using Console.WriteLine
, indicating the successful completion of the operation.
example.txt
before running code:
this is all the text in this file \n
Output:
Conclusion
In conclusion, this article has walked you through three effective approaches to appending text to a file in C#: the File.AppendAllText()
method, the StreamWriter
class, and the File.AppendText()
method. Through our focused exploration, we’ve seen how each method serves the same purpose but differs in implementation and use-case scenarios.
Whether you’re dealing with simple text additions or more complex file manipulations, these methods provide the necessary tools for efficient and safe file operations in C#. By understanding these techniques, you can enhance your file handling capabilities in C#, ensuring that your code is not only functional but also follows the best practices of the .NET ecosystem.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn