Agregar al archivo de texto en C#
-
Agregar a un archivo de texto con el método
File.AppendAllText()
enC#
-
Agregar a un archivo de texto con la clase
StreamWriter
enC#
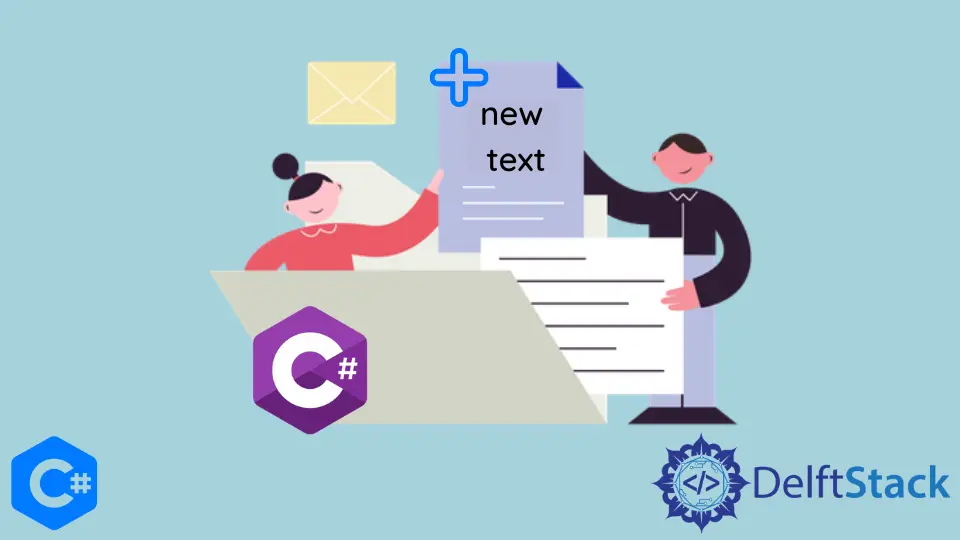
Este tutorial discutirá los métodos para agregar a un archivo de texto en C#.
Agregar a un archivo de texto con el método File.AppendAllText()
en C#
El método File.AppendAllText()
en C# se usa para abrir un archivo existente, agregar todo el texto al final del archivo y luego cerrar el archivo. Si el archivo no existe, el método File.AppendAllText()
crea un nuevo archivo vacío y escribe los datos en él. El método File.AppendAllText()
toma la ruta del archivo y el texto que se va a escribir como argumentos. El siguiente ejemplo de código nos muestra cómo agregar datos a un archivo de texto con el método File.AppendAllText()
en C#.
using System;
using System.IO;
namespace append_to_file {
class Program {
static void Main(string[] args) {
File.AppendAllText(@"C:\File\file.txt", "This is the new text" + Environment.NewLine);
}
}
}
file.txt
antes de ejecutar el código:
this is all the text in this file
file.txt
después de ejecutar el código:
this is all the text in this file This is the new text
En el código anterior, agregamos el texto This is the new text
y una nueva línea al final del archivo file.txt
dentro de la ruta C:\File
con el método File.AppendAllText()
en C#.
Agregar a un archivo de texto con la clase StreamWriter
en C#
Podemos lograr el mismo objetivo con la clase StreamWriter
. La clase StreamWriter
se utiliza para escribir texto en una secuencia o un archivo en C#. El método SreamWriter.WriteLine()
escribe una línea completa en C#. Podemos inicializar un objeto de la clase StreamWriter
con el método File.AppendText()
para inicializar una instancia de la clase StreamWriter
que agregaría los datos al archivo. El siguiente ejemplo de código nos muestra cómo podemos agregar datos al final de un archivo de texto con la clase StreamWriter
en C#.
using System;
using System.IO;
namespace append_to_file {
class Program {
static void Main(string[] args) {
using (StreamWriter sw = File.AppendText(@"C:\File\file.txt")) {
sw.WriteLine("This is the new text");
}
}
}
}
file.txt
antes de ejecutar el código:
this is all the text in this file
file.txt
después de ejecutar el código:
this is all the text in this file This is the new text
En el código anterior, agregamos el texto This is the new text
y una nueva línea al final del archivo file.txt
dentro de la ruta C:\File
con el método sw.WriteLine()
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn