How to Get Length of a 2D Array in C#
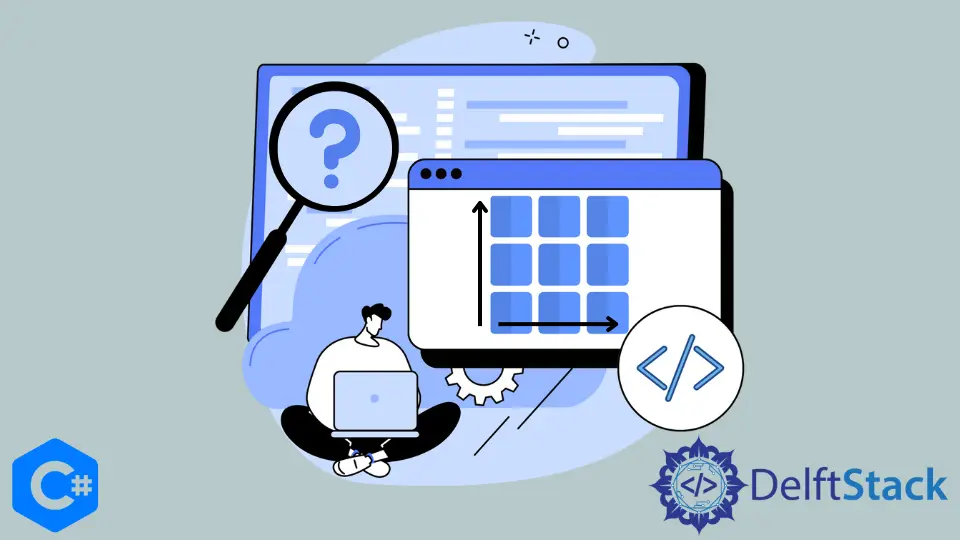
When working with multi-dimensional arrays in C#, you may often find yourself needing to determine the dimensions of a 2D array. Understanding how to get the length of a 2D array is crucial for efficient programming and managing data structures. In C#, there are two primary methods to calculate the width and height of a 2D array: the Array.GetLength()
method and the Array.GetUpperBound()
method.
In this article, we’ll explore both methods in detail, providing code examples and explanations to help you master this essential skill in C#.
Using Array.GetLength()
The Array.GetLength()
method is a straightforward way to retrieve the size of a particular dimension in a 2D array. This method requires you to specify the dimension index you want to measure. In a 2D array, dimension indices start at 0 for the first dimension (rows) and 1 for the second dimension (columns).
Here’s how you can use the Array.GetLength()
method:
using System;
class Program
{
static void Main()
{
int[,] myArray = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
int rows = myArray.GetLength(0);
int columns = myArray.GetLength(1);
Console.WriteLine("Number of rows: " + rows);
Console.WriteLine("Number of columns: " + columns);
}
}
Output:
Number of rows: 3
Number of columns: 3
The GetLength()
method retrieves the number of elements along the specified dimension. In this example, calling myArray.GetLength(0)
returns 3, indicating there are three rows, while myArray.GetLength(1)
returns 3, showing there are three columns. This method is useful for scenarios where you need to iterate over the array or perform operations based on its dimensions.
In addition to its simplicity, Array.GetLength()
is particularly efficient because it directly accesses the array’s metadata, making it a go-to choice for developers needing quick dimension checks. It’s worth noting that this method can be applied to arrays of any rank, making it versatile for multi-dimensional structures beyond just 2D arrays.
Using Array.GetUpperBound()
Another method to determine the dimensions of a 2D array is by using the Array.GetUpperBound()
method. This method returns the highest index of a specified dimension, which can then be used to calculate the length by adding one. It’s slightly less intuitive than GetLength()
, but it can be useful in certain contexts.
Here’s how to use the Array.GetUpperBound()
method:
using System;
class Program
{
static void Main()
{
int[,] myArray = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
int rows = myArray.GetUpperBound(0) + 1;
int columns = myArray.GetUpperBound(1) + 1;
Console.WriteLine("Number of rows: " + rows);
Console.WriteLine("Number of columns: " + columns);
}
}
Output:
Number of rows: 3
Number of columns: 3
In this example, GetUpperBound(0)
returns 2, which is the highest index for the rows (0, 1, 2). By adding 1, we calculate the total number of rows as 3. Similarly, GetUpperBound(1)
gives us the highest index for the columns, which is also 2, leading us to the same conclusion about the number of columns.
The GetUpperBound()
method is particularly advantageous when working with arrays of varying dimensions, as it provides the flexibility to handle different ranks. However, it requires an additional step of adding one to convert the index to a count, which might not be as straightforward as GetLength()
for some programmers.
Conclusion
Understanding how to get the length of a 2D array in C# is essential for effective data manipulation. Both Array.GetLength()
and Array.GetUpperBound()
offer reliable methods to retrieve the dimensions of your arrays, each with its own advantages. Whether you prefer the simplicity of GetLength()
or the versatility of GetUpperBound()
, mastering these methods will enhance your programming skills and help you manage data structures more effectively.
FAQ
-
What is a 2D array in C#?
A 2D array in C# is a data structure that allows you to store data in a table format, consisting of rows and columns. -
Can I use these methods for arrays of different dimensions?
Yes, bothGetLength()
andGetUpperBound()
can be used for multi-dimensional arrays, not just 2D arrays.
-
What happens if I use an invalid dimension index?
Using an invalid dimension index will throw anIndexOutOfRangeException
. -
Are there performance differences between the two methods?
Generally,GetLength()
is slightly more efficient since it directly accesses the array’s metadata. -
Can I use these methods on jagged arrays?
No, jagged arrays are arrays of arrays, and you would need to use different techniques to determine their lengths.
#. This article explores two main methods: Array.GetLength() and Array.GetUpperBound(). Discover code examples and detailed explanations to enhance your C# programming skills. Get started today!
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn