How to Copy a Object in C#
-
Copy an Object With the
MemberWiseClone()
Method inC#
-
Copy an Object With the Parameterized Constructor Method in
C#
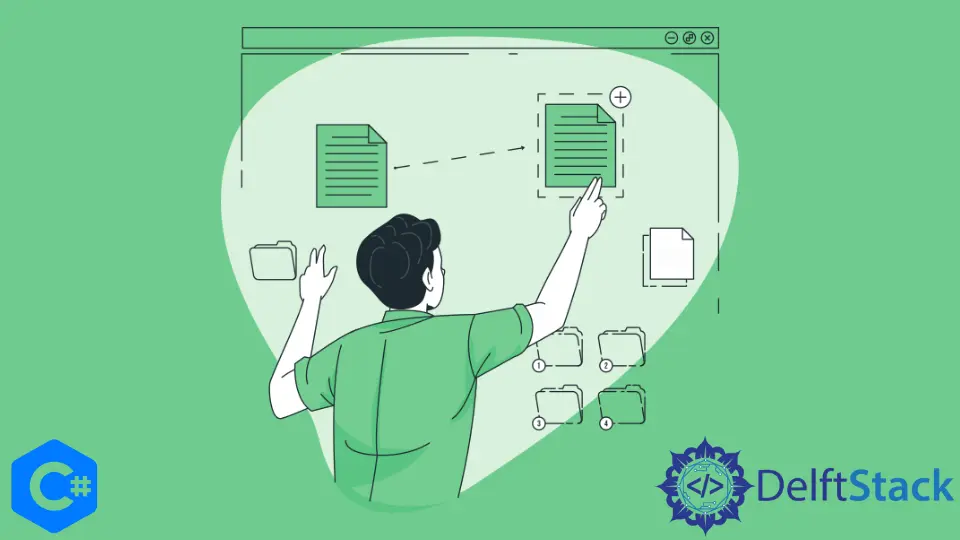
This tutorial will introduce methods to copy an object in C#.
Copy an Object With the MemberWiseClone()
Method in C#
Unfortunately, there is no built-in way of creating a separate copy of an object in C#. This phenomenon is demonstrated in the following code example.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace copy_an_object {
class myClass {
public String test;
}
class Program {
static void Main(string[] args) {
myClass a = new myClass();
a.test = "This is a test";
myClass b = a;
b.test = "This is not a test";
Console.WriteLine(a.test);
Console.WriteLine(b.test);
}
}
}
Output:
This is not a test
This is not a test
We get the same output both times because an instance of a class in C# is a reference-type variable that points to a specific memory location. Due to the Object-Oriented nature of C#, the new pointer b
is also pointing to the memory location of a
.
If we want to create a separate memory location for b
, we have to rely on some user-defined approaches. The MemberWiseClone()
method is used to create a separate copy of the values of the calling object in C#. The return type of the MemberWiseClone()
function is object. The following code example shows us how to create a separate copy of a class object with the MemberWiseClone()
function in C#.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace copy_an_object {
class myClass {
public String test;
public object Clone() {
return this.MemberwiseClone();
}
}
class Program {
static void Main(string[] args) {
myClass a = new myClass();
a.test = "This is a test";
myClass b = (myClass)a.Clone();
b.test = "This is not a test";
Console.WriteLine(a.test);
Console.WriteLine(b.test);
}
}
}
Output:
This is a test
This is not a test
This time it is clear from the output that there is a separate copy for each class object. We implemented the MemberWiseClone()
function inside the Clone()
function of the myClass
class. The Clone()
function returns a separate copy of the class object. The return value of the Clone()
function is type-casted to myClass
and pointed to by the b
class object.
Copy an Object With the Parameterized Constructor Method in C#
We can create a parameterized class constructor to achieve the same goal in C#. We can pass the previous class object to the constructor of a new class object and copy all the values from it. The following code example shows us how we can create a separate copy of a class object with the parameterized constructor method in C#
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace copy_an_object {
class MyClass {
public String test;
public MyClass() {}
public MyClass(MyClass other) {
test = other.test;
}
}
class Program {
static void Main(string[] args) {
MyClass a = new myClass();
a.test = "This is a test";
MyClass b = new MyClass(a);
b.test = "This is not a test";
Console.WriteLine(a.test);
Console.WriteLine(b.test);
}
}
}
Output:
This is a test
This is not a test
We created a parameterized constructor for the MyClass
class that takes an object of the MyClass
class and copies the test
string from it. In the main function, we created the object a
of the MyClass
class and passed it to the constructor of b
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn