How to Extend a Class in C#
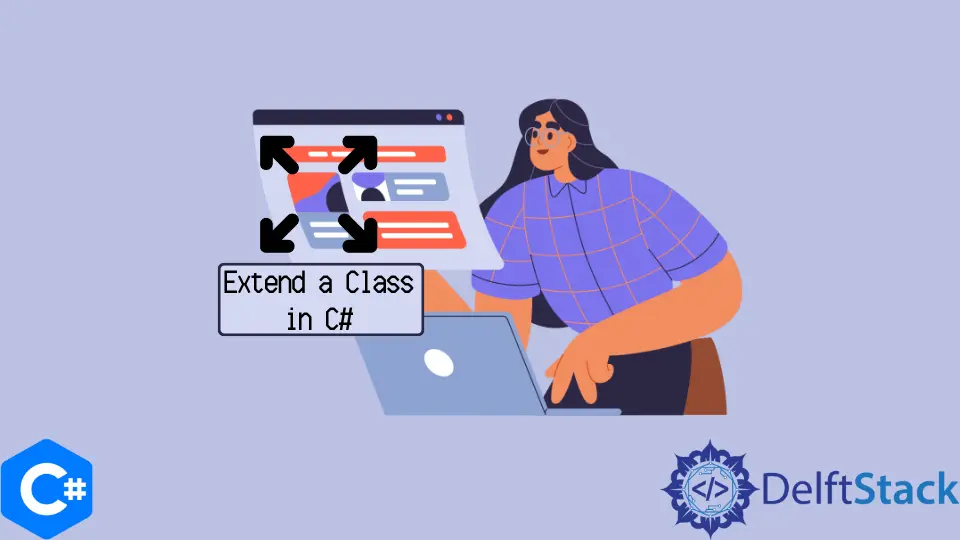
This tutorial will teach you how to extend a class using the C# programming language.
Use Inheritance to Extend a Class in C#
When working with Object-Oriented Programming (OOP
), inheritance is associated with a hierarchical level. Although it is feasible to cast a derived
class to its base
class, we cannot do the reverse.
It is impossible to cast from a base
class to a derived
class. Take a look at the following example to understand better.
In the following example, we’ll add properties to the new object one at a time and then use the new object to extend a single property from the previous one.
-
To begin, import the following libraries.
using System; using System.Collections.Generic; using System.Linq; using System.Text.RegularExpressions;
-
Create a
Person
class havingFirstname
andLastname
properties.public class Person { public string Firstname { get; set; } public string Lastname { get; set; } public Person(string fname, string lname) { Firstname = fname; Lastname = lname; } }
-
Constructor overloading will now be used to build a
Student
from aPerson
.public class Student : Person { public Student(Person person, string code) : base(person.Firstname, person.Lastname) { this.code = code; } public Student(Person person) : base(person.Firstname, person.Lastname) {} public string code { get; set; } }
-
In the
Main()
method, we’ll create aPerson
object and populate it with data as below.Person person = new Person("Muhammad Zeeshan", "Khan");
-
Then, create two
Student
objects nameds1
ands2
and pass theperson
object andcode
as parameters.Student s1 = new Student(person, "3229"); Student s2 = new Student(person, "3227");
-
Lastly, we’ll print the
Student
objects holdingPerson
properties.Console.WriteLine(s1.code); Console.WriteLine(s2.code);
-
Complete Source Code.
using System; using System.Collections.Generic; using System.Linq; using System.Text.RegularExpressions; public class Person { public string Firstname { get; set; } public string Lastname { get; set; } public Person(string fname, string lname) { Firstname = fname; Lastname = lname; } } public class Student : Person { public Student(Person person, string code) : base(person.Firstname, person.Lastname) { this.code = code; } public Student(Person person) : base(person.Firstname, person.Lastname) {} public string code { get; set; } } static class Program { static void Main(string[] args) { Person person = new Person("Muhammad Zeeshan", "Khan"); Student s1 = new Student(person, "3229"); Student s2 = new Student(person, "3227"); Console.WriteLine(s1.code); Console.WriteLine(s2.code); } }
Output:
3229 3227
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn