Nested Classes in C#
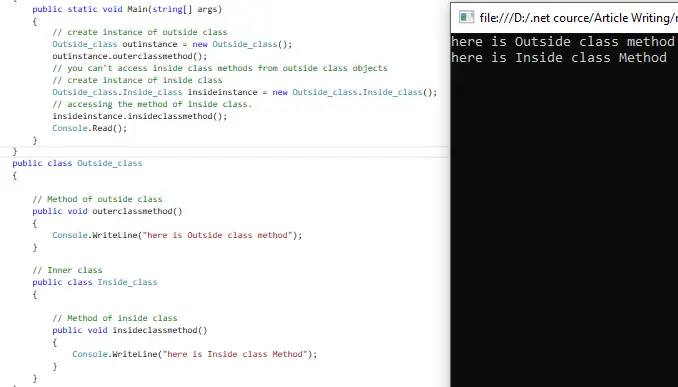
This tutorial will introduce nested classes in the C# programming language.
A class is a blueprint or pattern defined by the user and used to build things. It is a single instance that combines methods and fields (member functions that define actions).
Nested Classes in C#
In C#, a user can define a class inside another, called nested classes. Its primary purpose is to limit the scope of the nested class.
Compared to regular classes, nested classes offer the additional possibility of private modifiers and protection. If you only need to invoke this class from within the parent class context, it’s usually an excellent idea to define it as a nested class.
Suppose this class will be used outside of the library. It is usually more accessible for the user to define it as a separate same-level class, regardless of whether the two classes have any conceptual relationship.
Even if it is technically feasible to build a public class nesting inside a public parent class, this is rarely a good idea.
Reasons to Use Nested Classes in C#
There are several strong reasons to use nested classes.
- It is a practical technique of grouping classes only used once.
- Encapsulation is improved.
- Nested classes can make code easier to read and maintain.
- It facilitates logical class grouping.
If a class is only valuable to one other class, it makes sense to include it in that class to keep the two together. It is more simplified by nesting such helper classes.
Along with public and internal access modifiers, a nested class can include private and protected internal access modifiers.
There are occasions when implementing an interface returned from within the class is beneficial. Still, the implementation of that interface should be entirely hidden from the outside world.
For example, before yield was introduced to C#, we embedded the enumerator implementation inside a collection as a private class to build them. The collection members would have simple access, but the outside world would not need or see the intricacies of how this is done.
Implement Nested Classes in C#
Below is the code to illustrate the concept of nested class.
The class Program
is the main class of the program, which has a public static main method. We created the outside class Outside_class
as public and another class inside named Inside_class
as public.
Further, we created two methods: inside the Outside_class
and another inside the Inside_class
. We can not access the Inside_class
methods from Outside_class
objects.
To access the method inside this, we need to create an instance of the Inside_class
.
using System;
public class Program {
public static void Main(string[] args) {
// create instance of outside class
Outside_class outinstance = new Outside_class();
outinstance.outerclassmethod();
// you can't access inside class methods from outside class objects
// create instance of inside class
Outside_class.Inside_class insideinstance = new Outside_class.Inside_class();
// accessing the method of inside class.
insideinstance.insideclassmethod();
Console.Read();
}
}
public class Outside_class {
// Method of outside class
public void outerclassmethod() {
Console.WriteLine("here is Outside class method");
}
// Inner class
public class Inside_class {
// Method of inside class
public void insideclassmethod() {
Console.WriteLine("here is Inside class Method");
}
}
}
Below is an image of the code using nested classes with output.
It is already clear that the nested classes make our code easily readable. We can put together all those classes used with each other.